1. |
|
Data augmentation method based on AugMix.
|
|
Original |
Augmented |
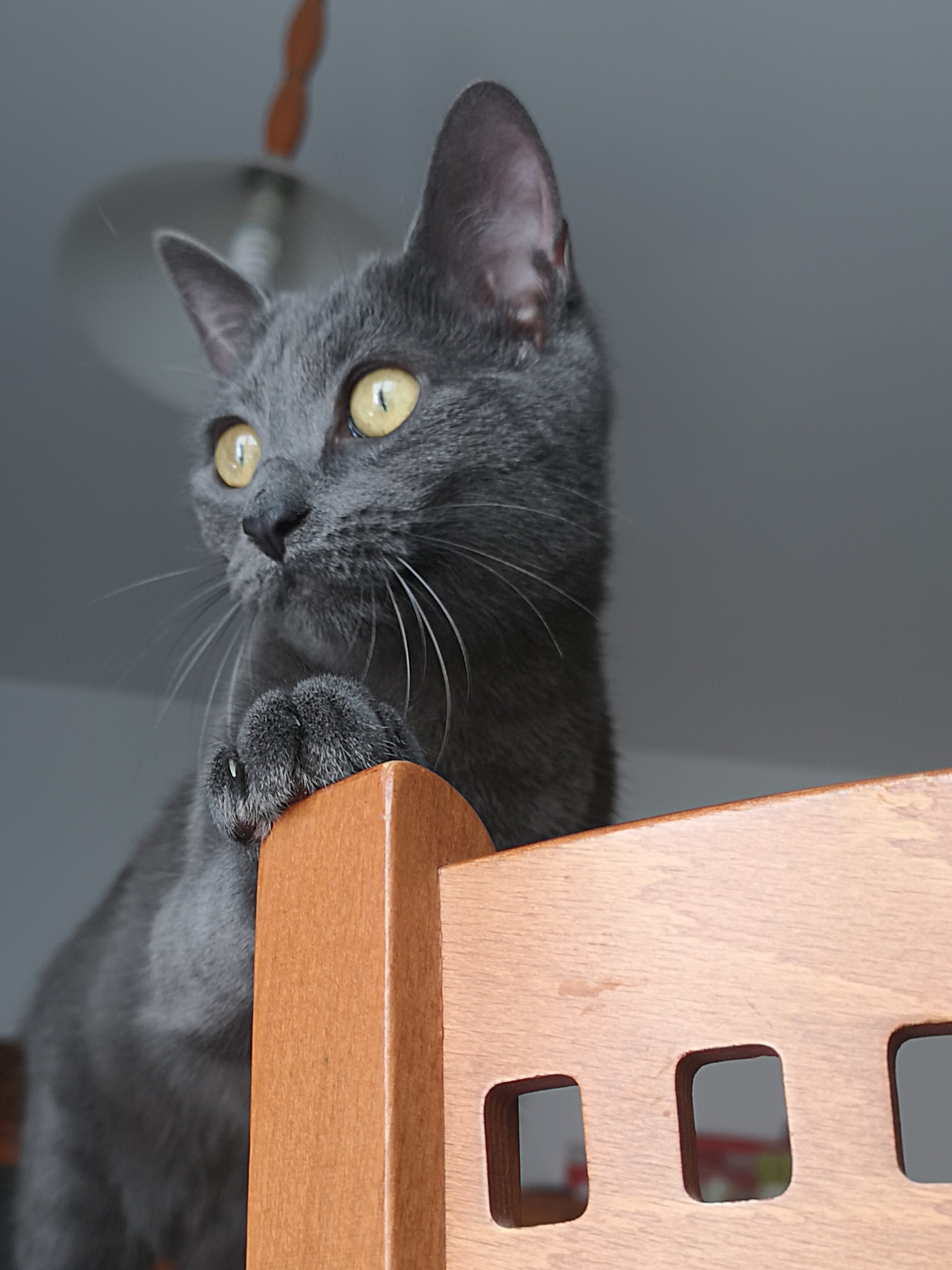 |
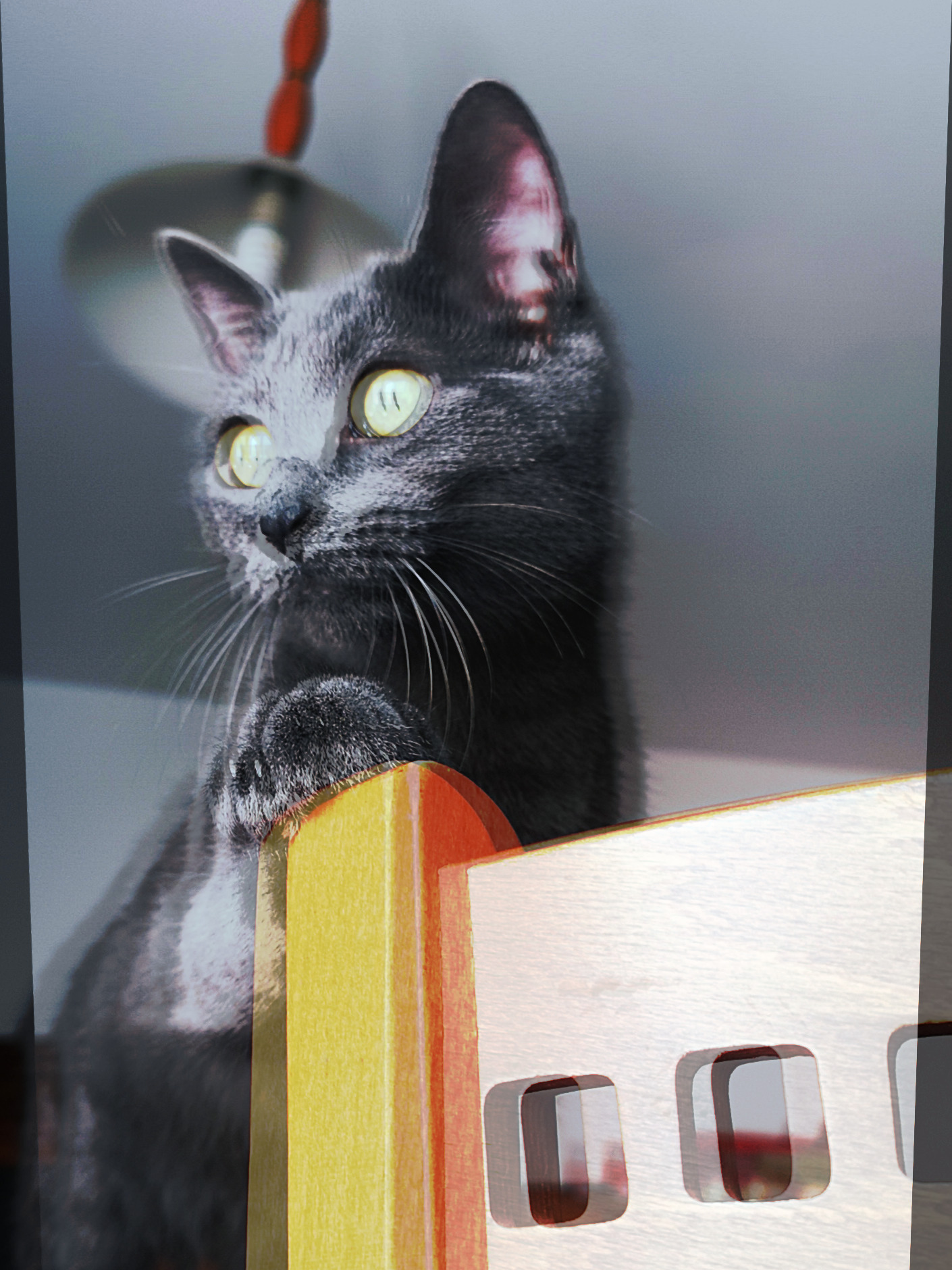 |
Original |
Augmented |
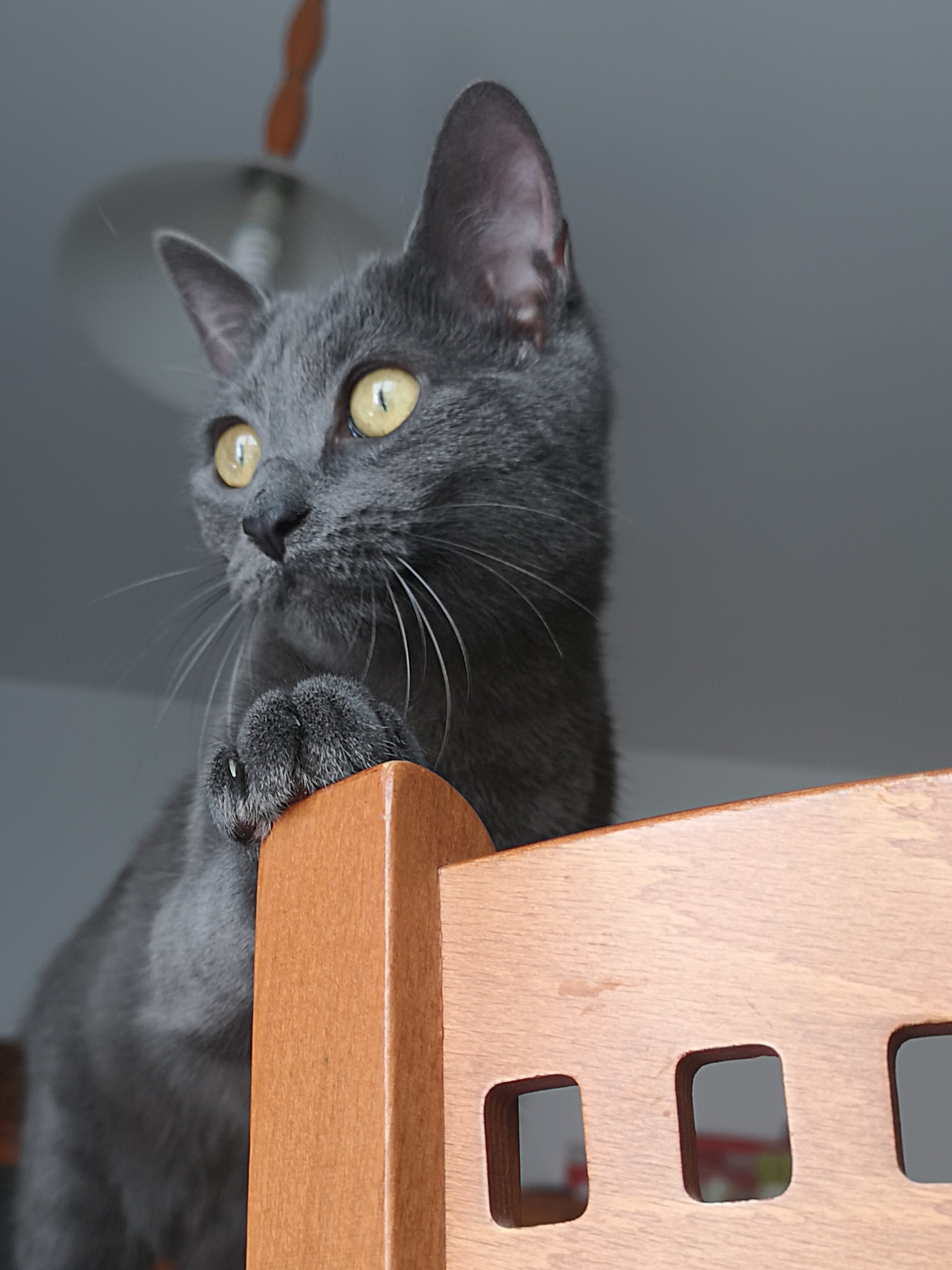 |
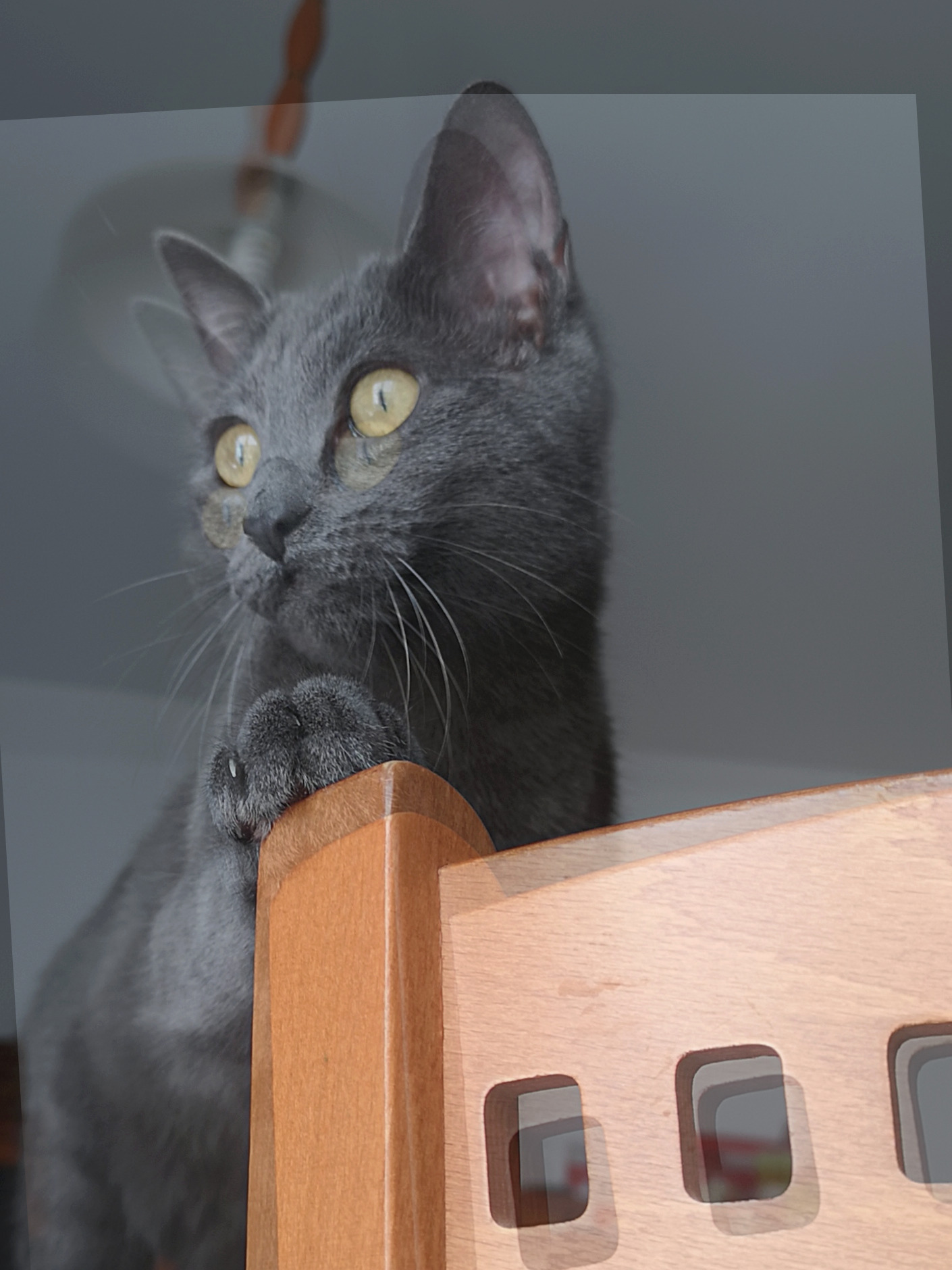 |
Original |
Augmented |
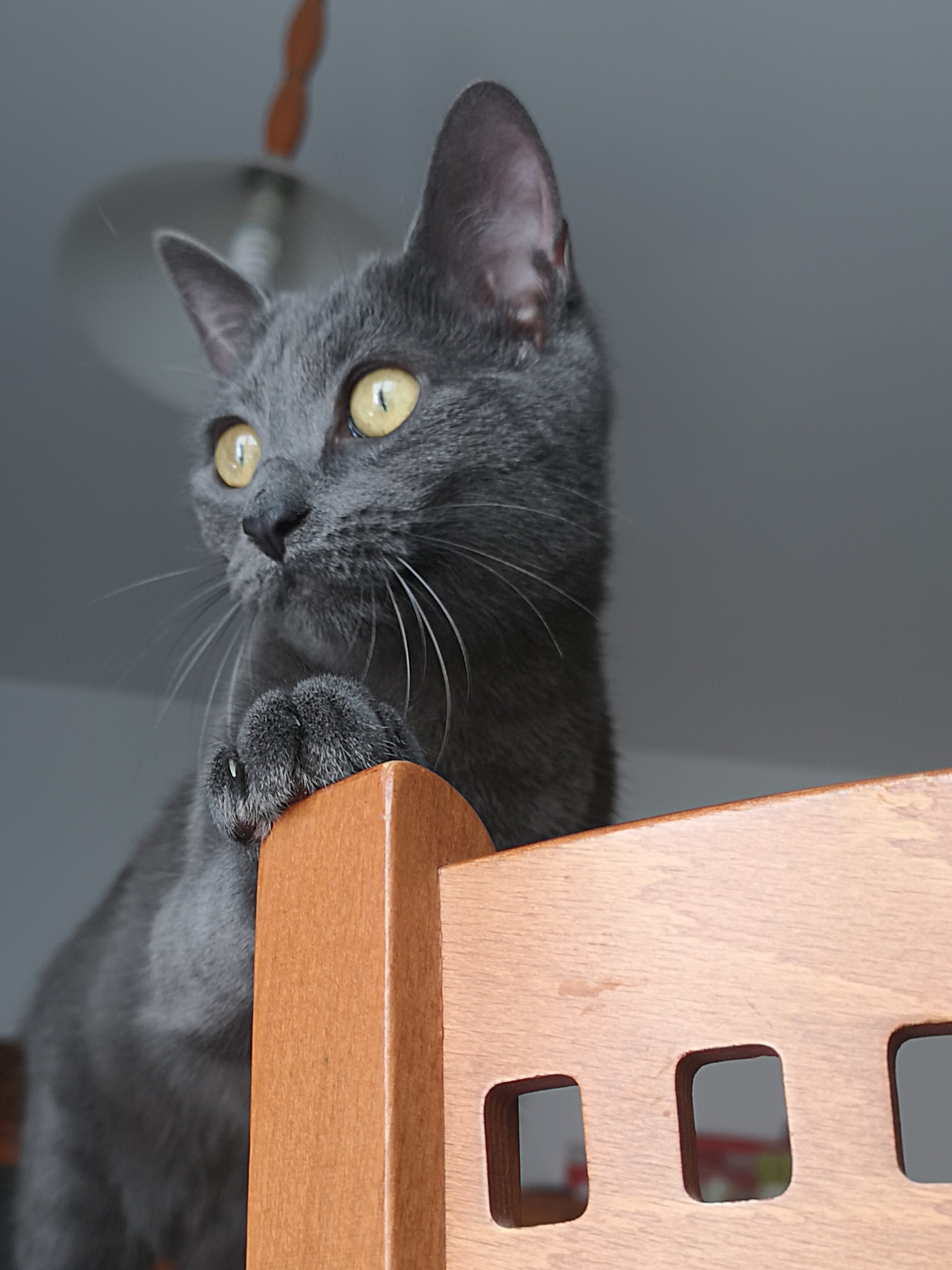 |
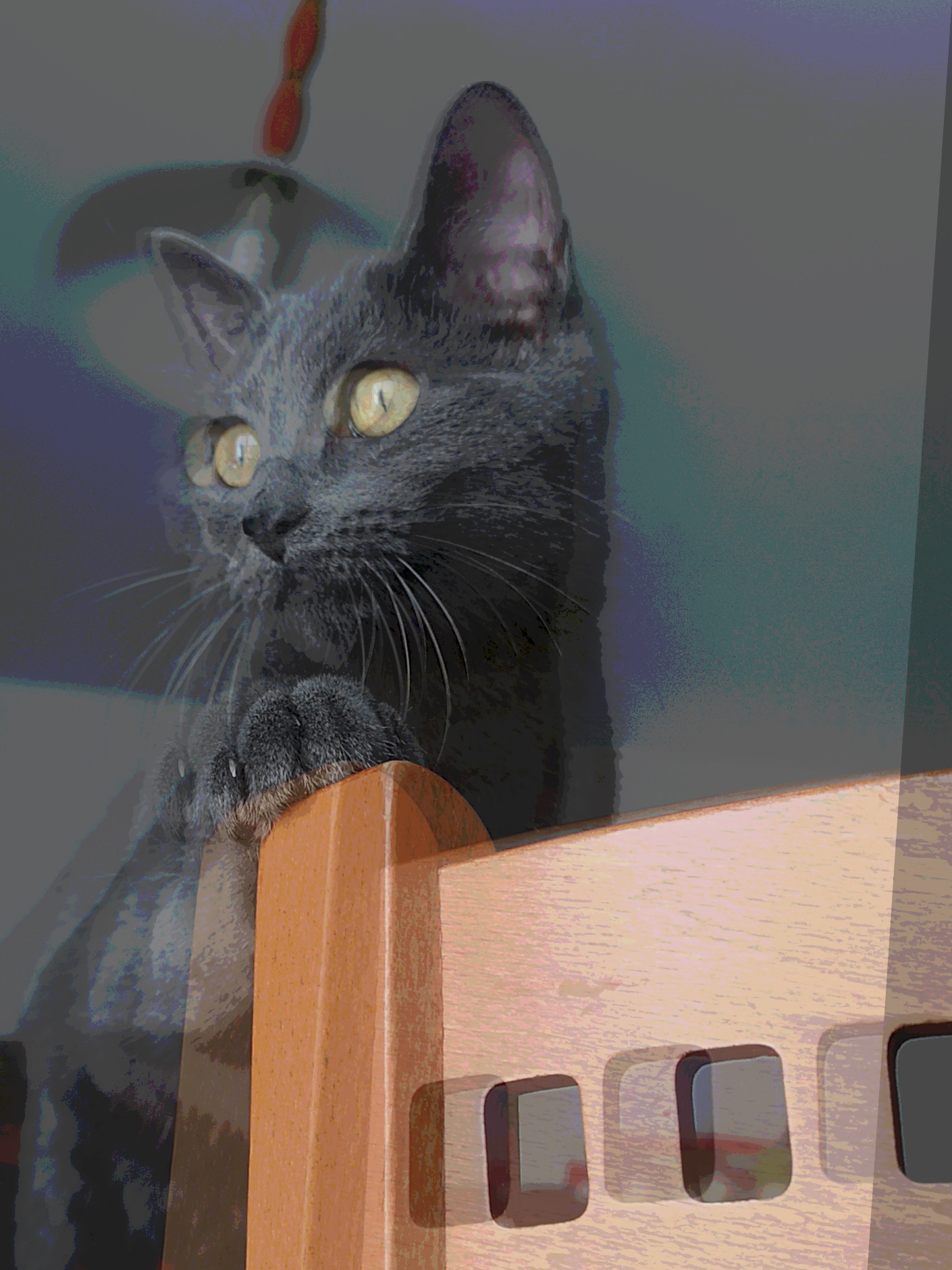 |
out_img = T.AugMix(severity=3, mixture_width=3, chain_depth=-1,
alpha=1.0, all_ops=True,
interpolation=T.InterpolationMode.BILINEAR,
fill=None)(img)
|
|
2. |
|
Data augmentation method based on AutoAugment.
|
|
Original |
Augmented |
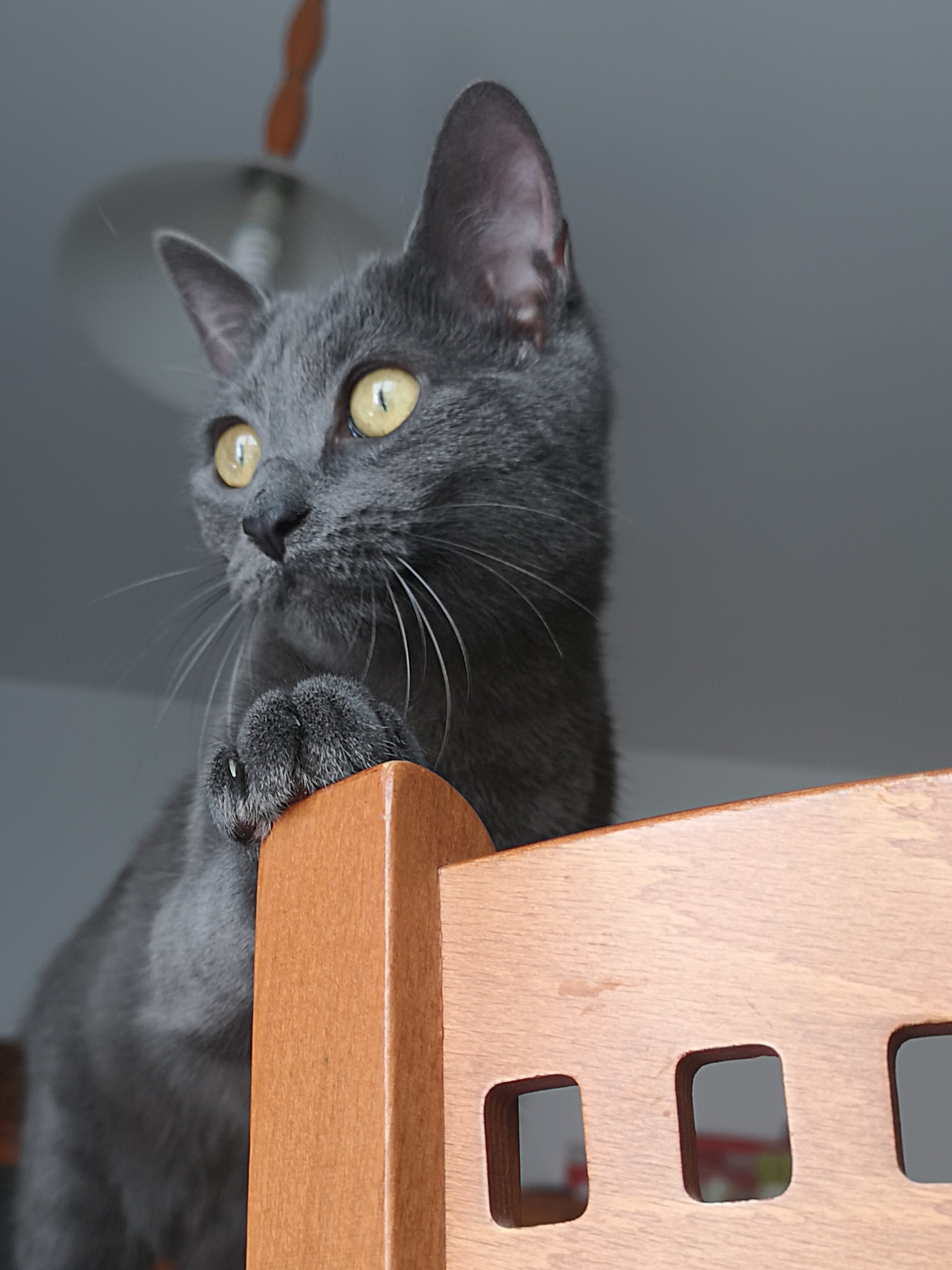 |
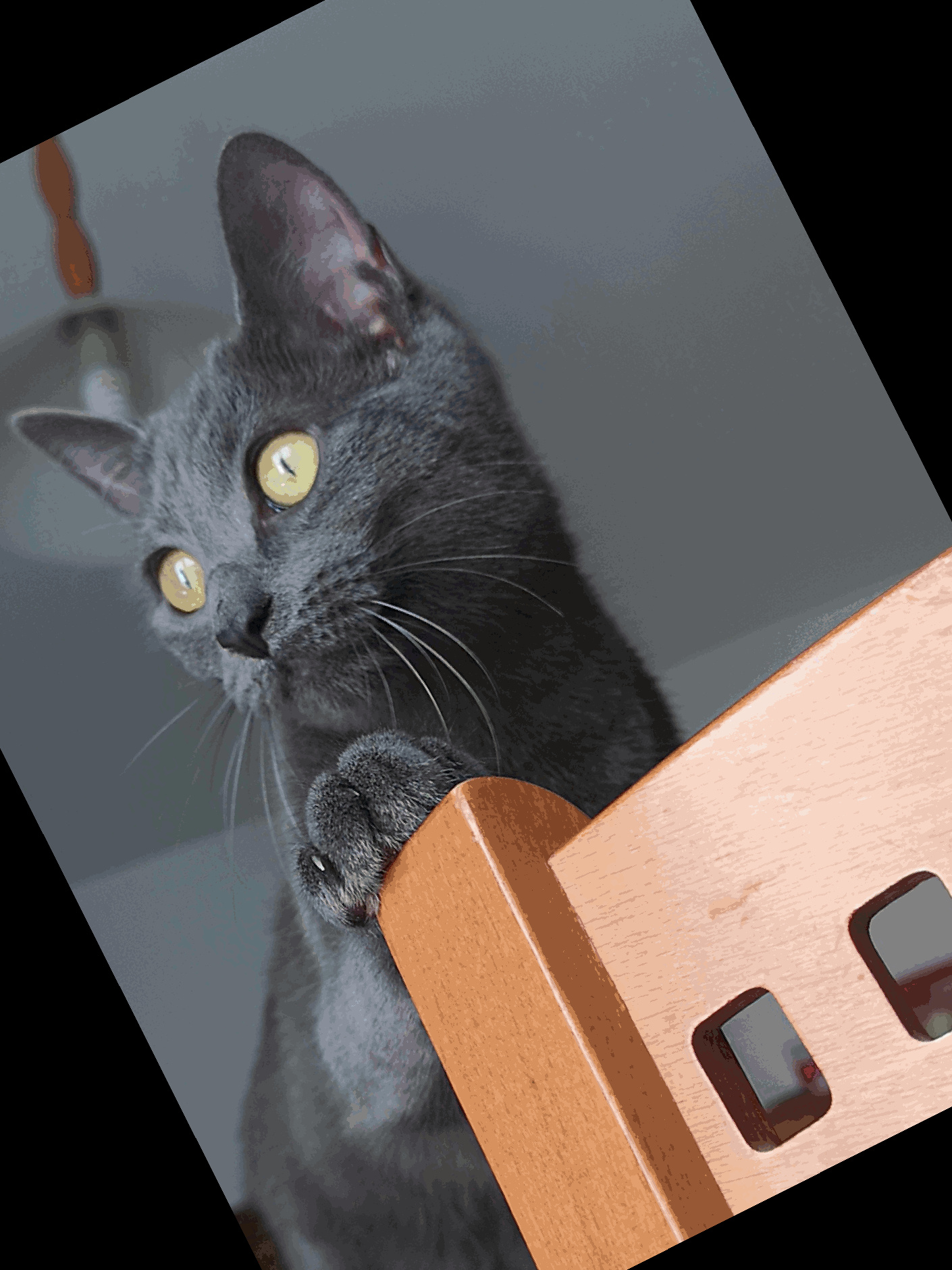 |
policy = T.AutoAugmentPolicy.IMAGENET
interpolation = T.InterpolationMode.NEAREST
out_img = T.AutoAugment(policy, interpolation, fill=None)(img)
|
|
3. |
|
Crops the given image at the center.
|
BASIC GEOMETRY
|
Original |
Augmented |
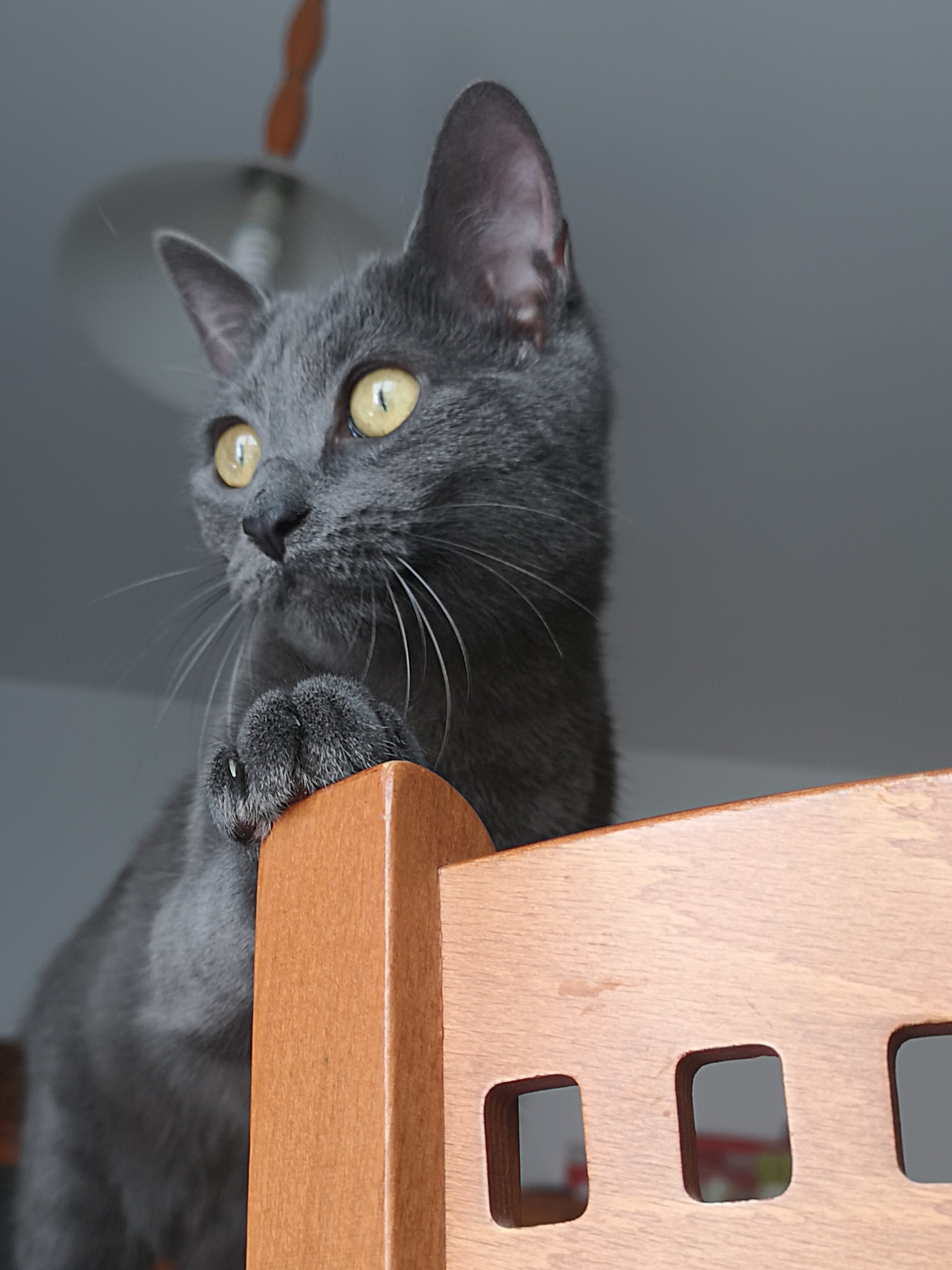 |
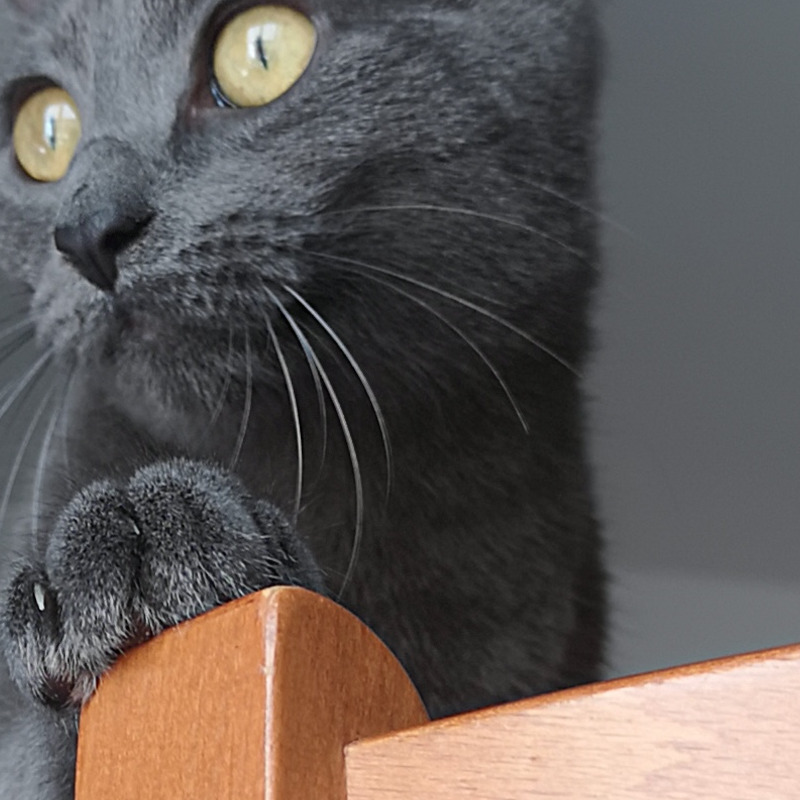 |
out_img = T.CenterCrop(size=800)(img)
|
Original |
Augmented |
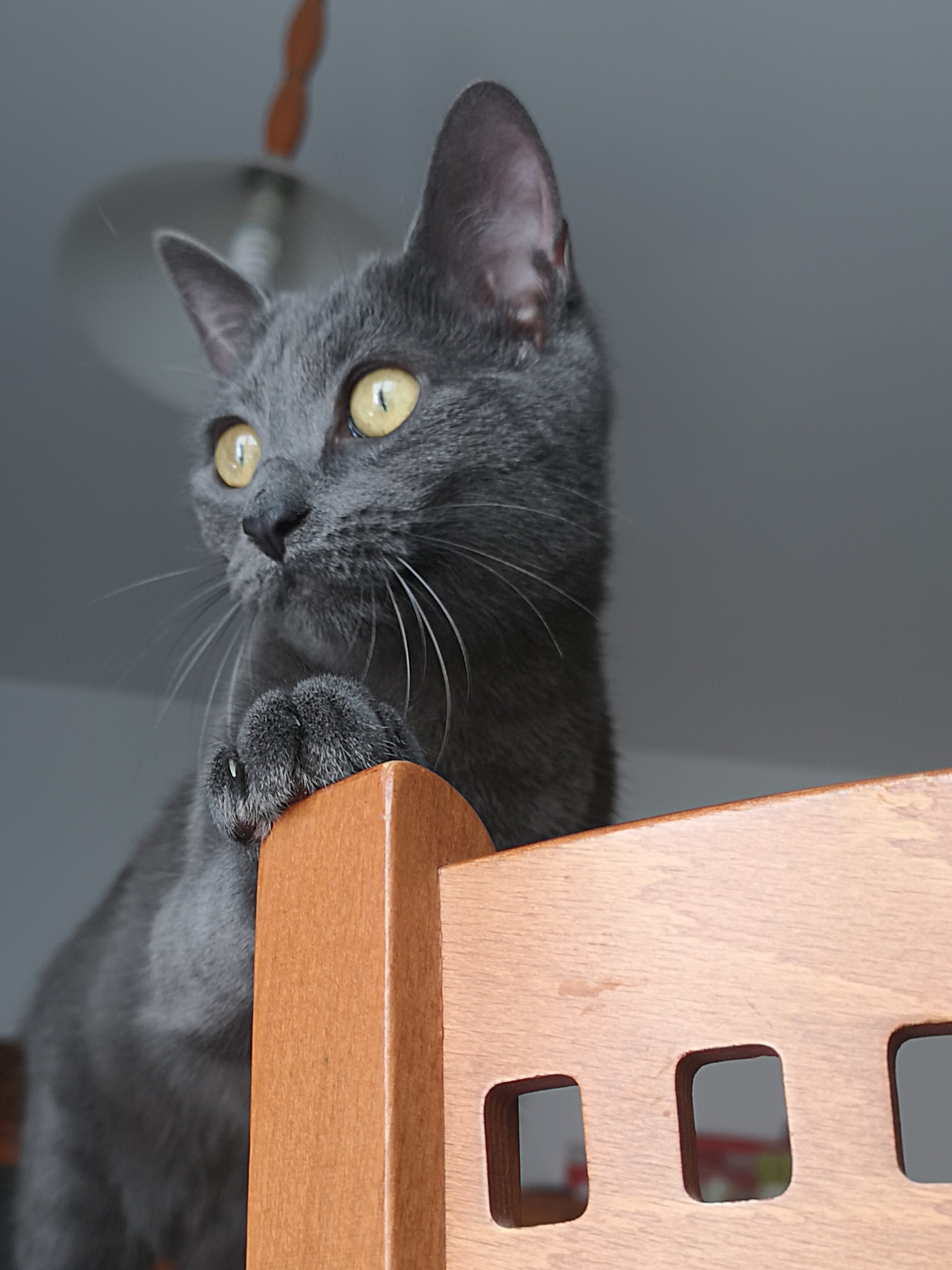 |
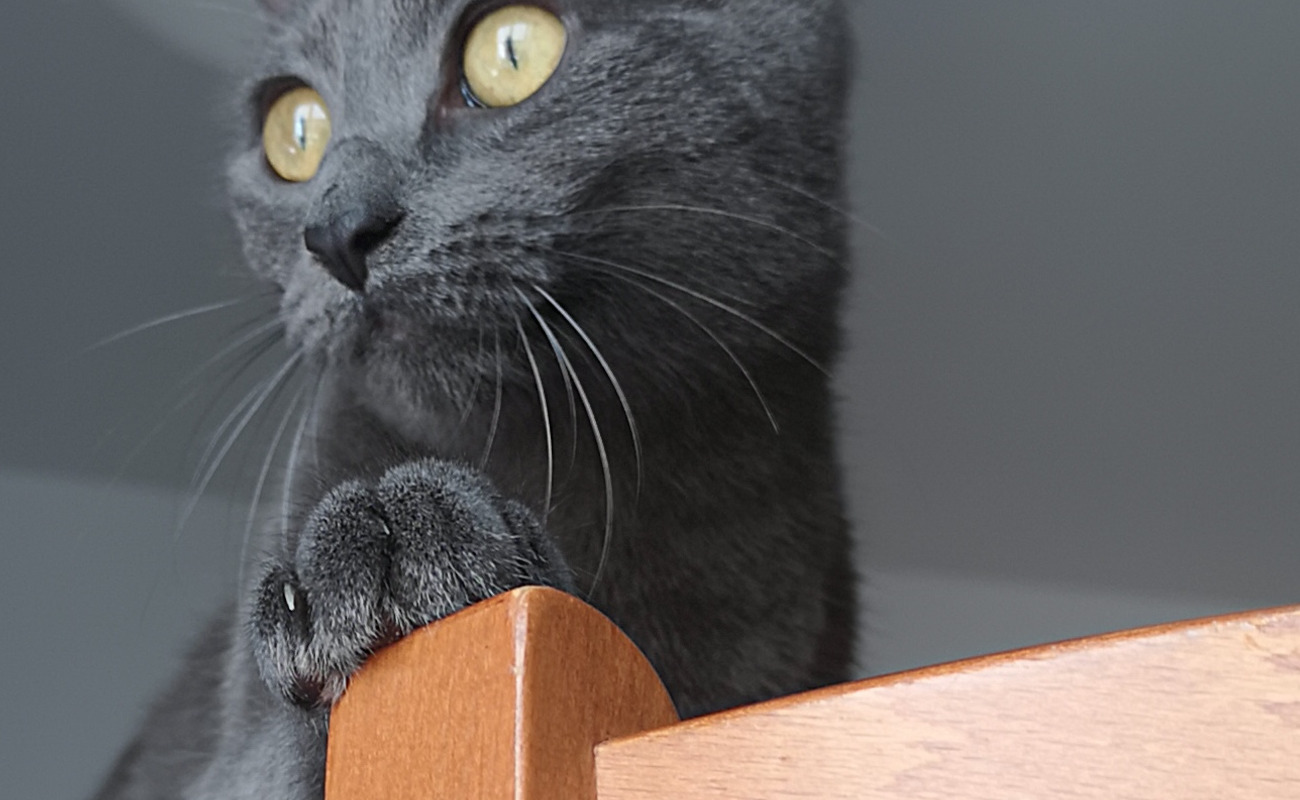 |
out_img = T.CenterCrop(size=(800,1300))(img)
|
|
4. |
|
Randomly changes the brightness, contrast, saturation and hue of an image.
|
PHOTOMETRY
|
Original |
Augmented |
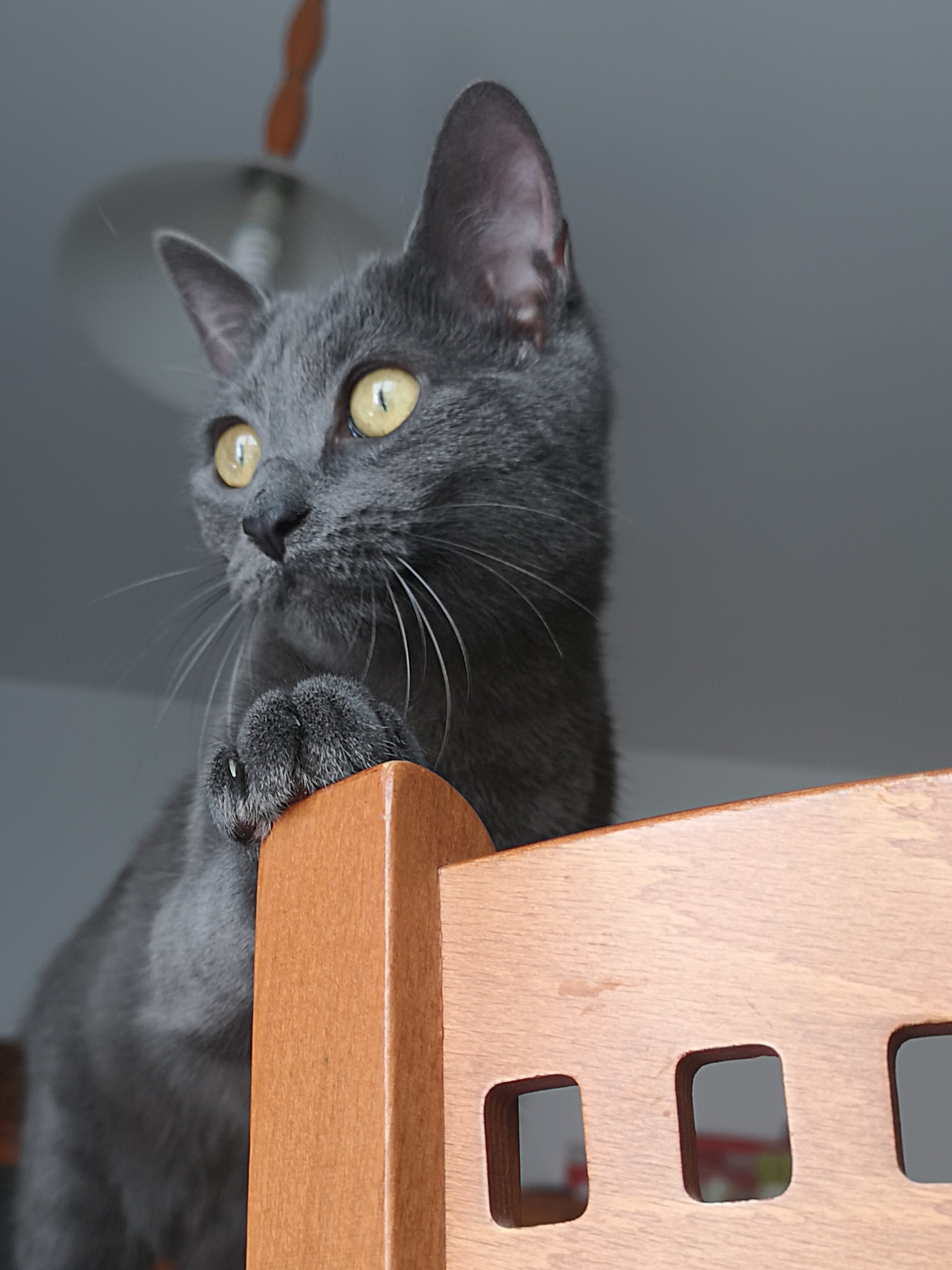 |
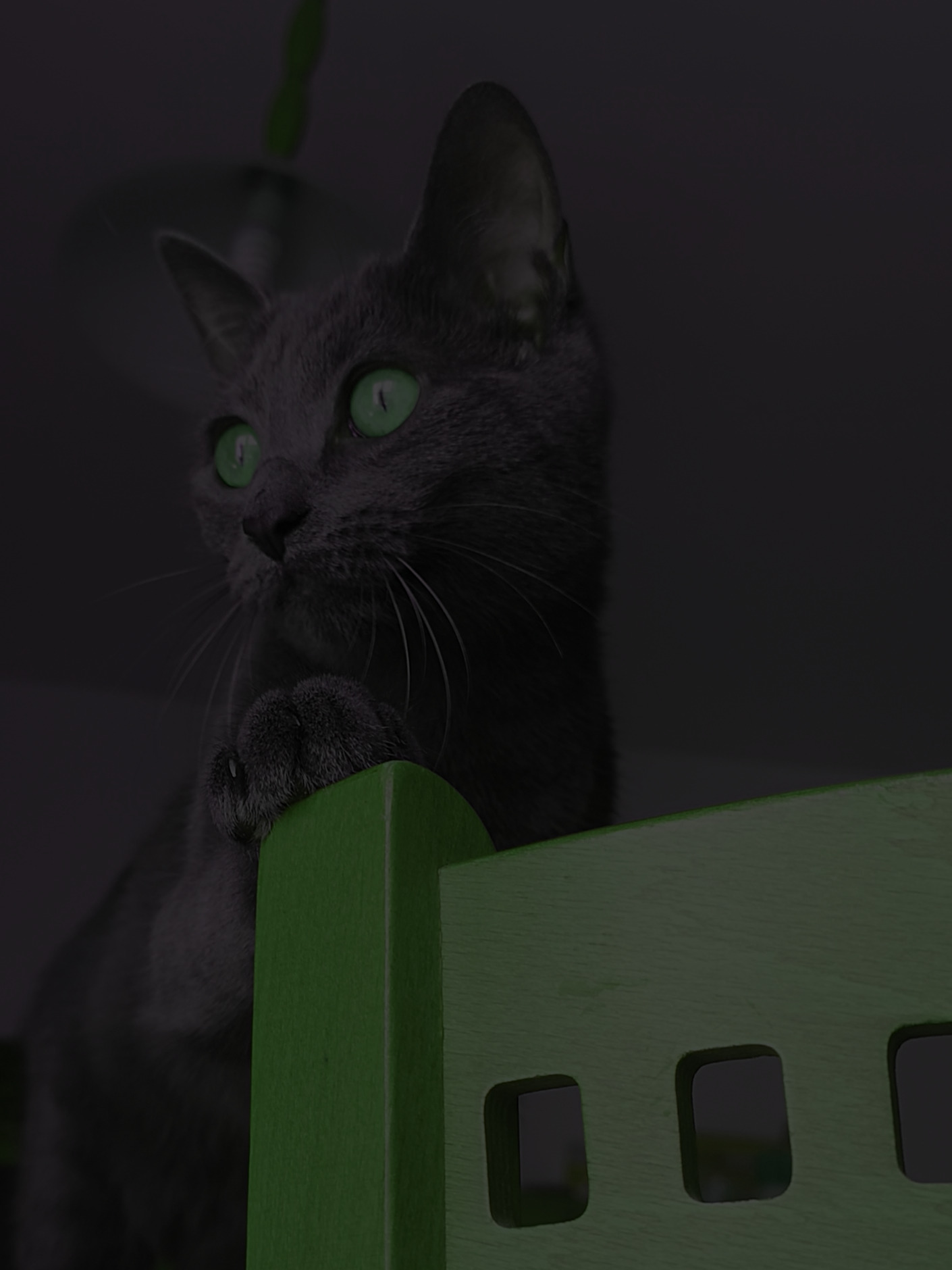 |
out_img = T.ColorJitter(brightness=(0,1), contrast=0, saturation=0,
hue=(0,0.5))(img)
|
Original |
Augmented |
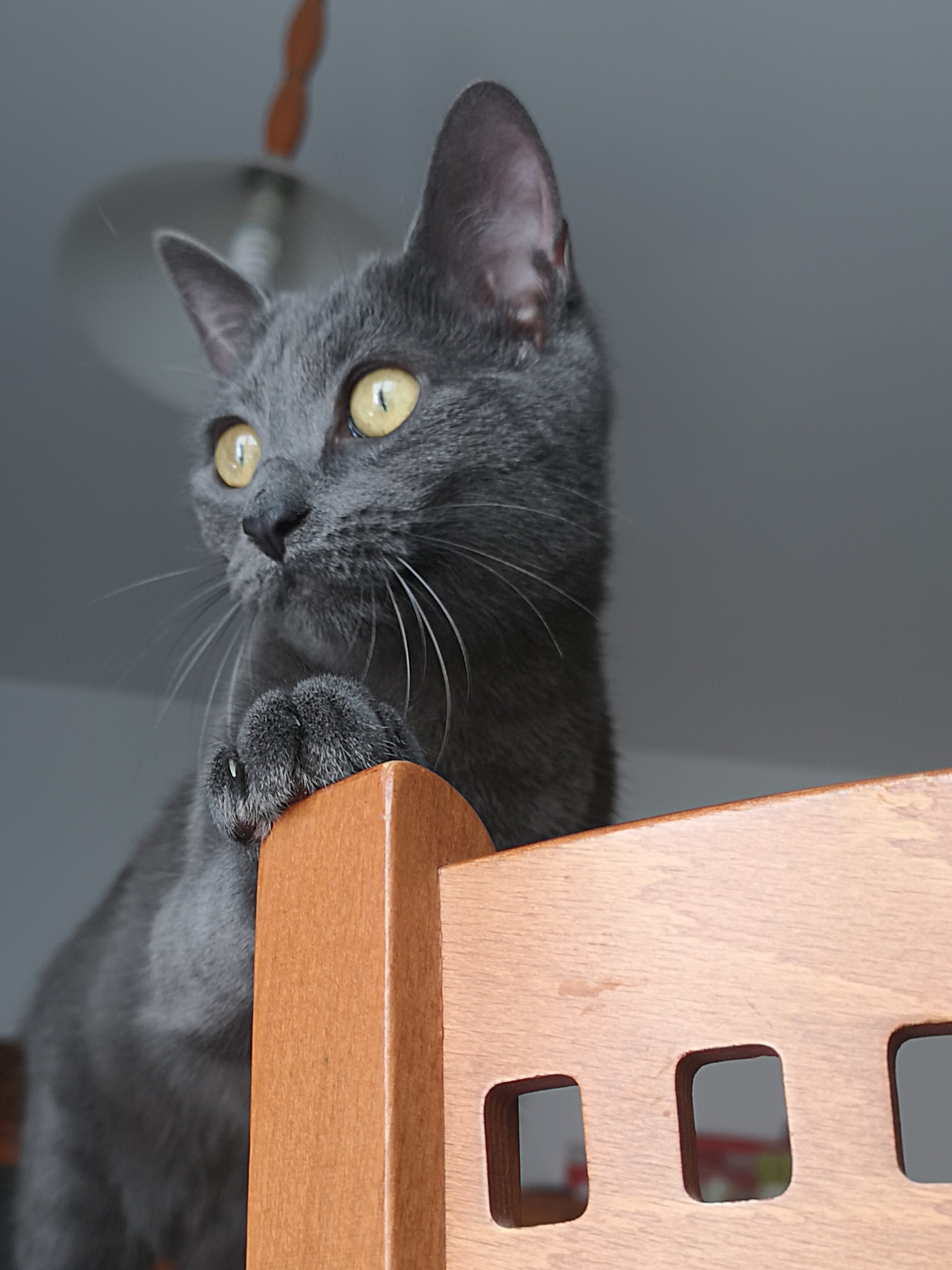 |
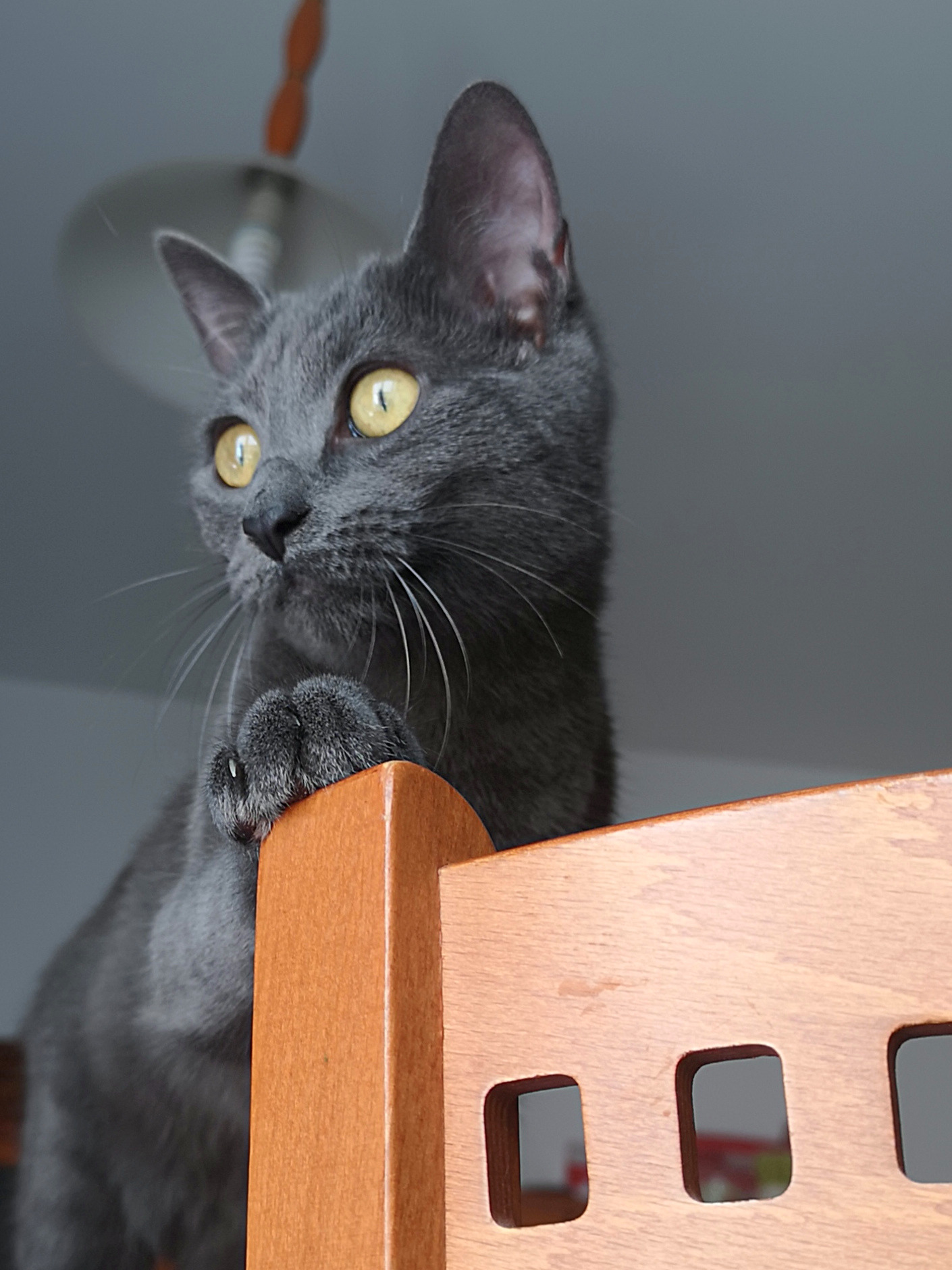 |
out_img = T.ColorJitter(brightness=0, contrast=0.5, saturation=1,
hue=0)(img)
|
|
5. |
|
Composes several transforms together.
|
|
6. |
|
Crops the given image into four corners and the central crop.
|
BASIC GEOMETRY
|
Original |
Augmented |
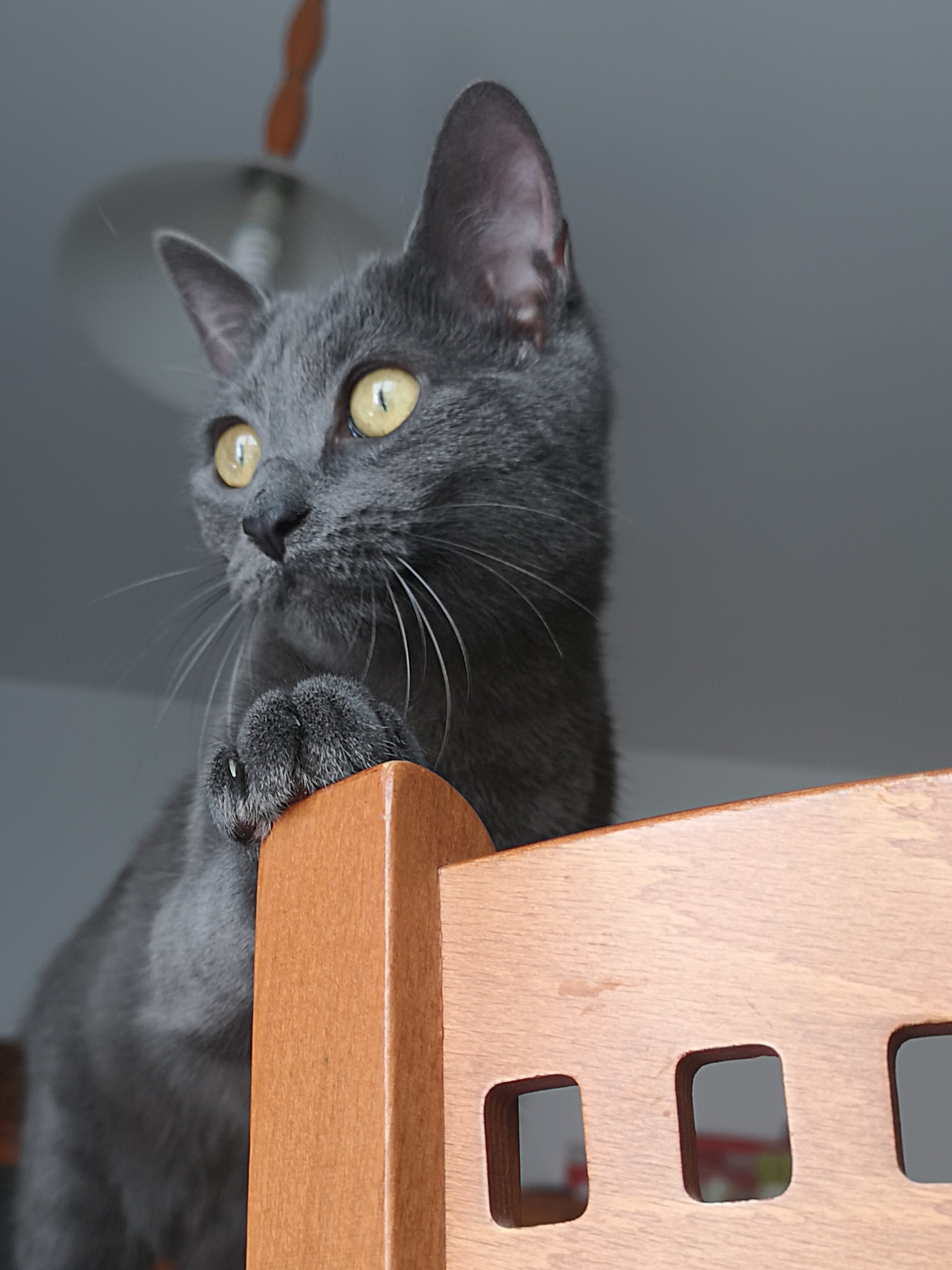 |
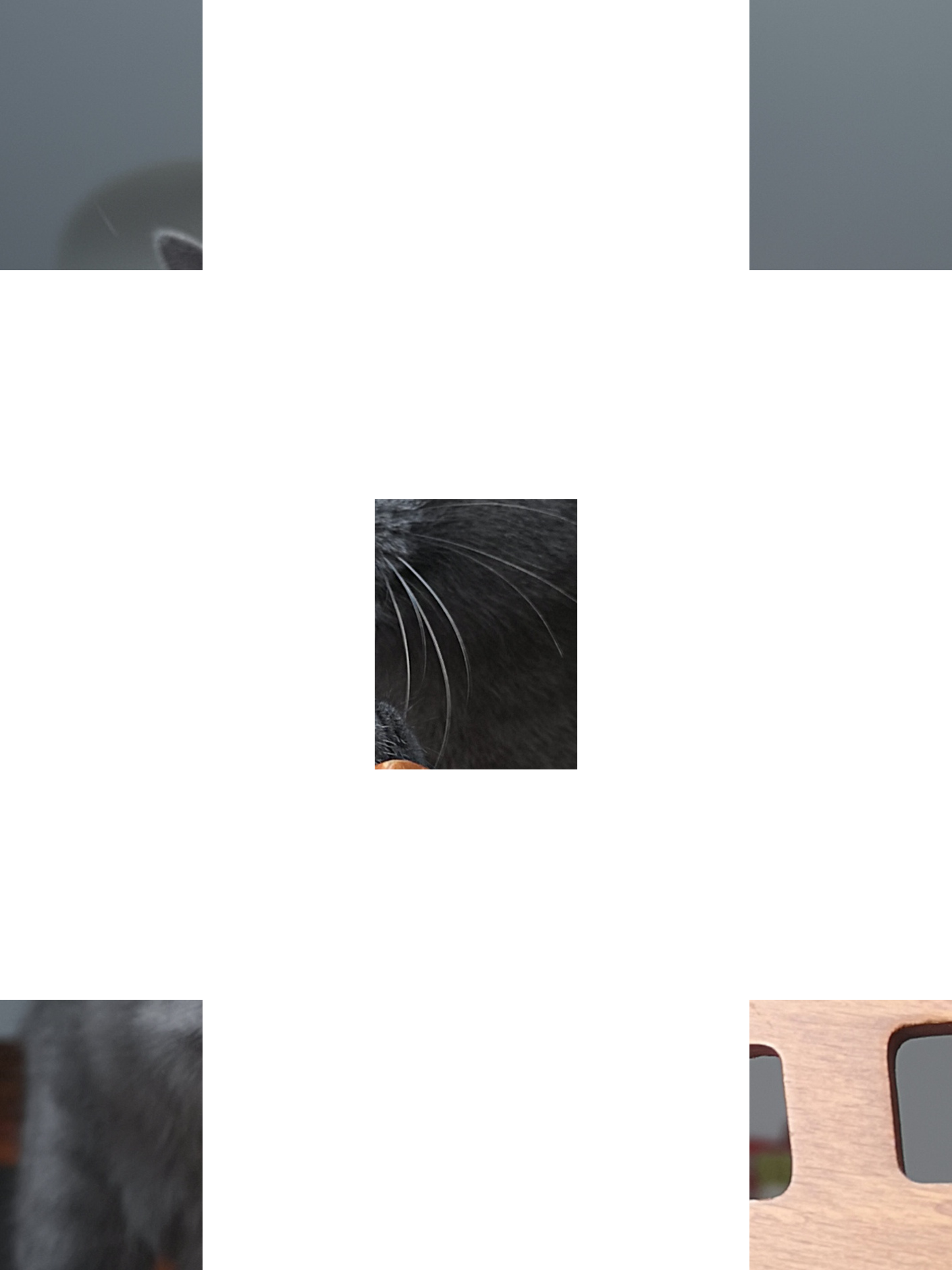 |
out_img = T.FiveCrop((400,300))(img)
|
|
7. |
|
Blurs image with randomly chosen Gaussian blur.
|
BLUR
|
Original |
Augmented |
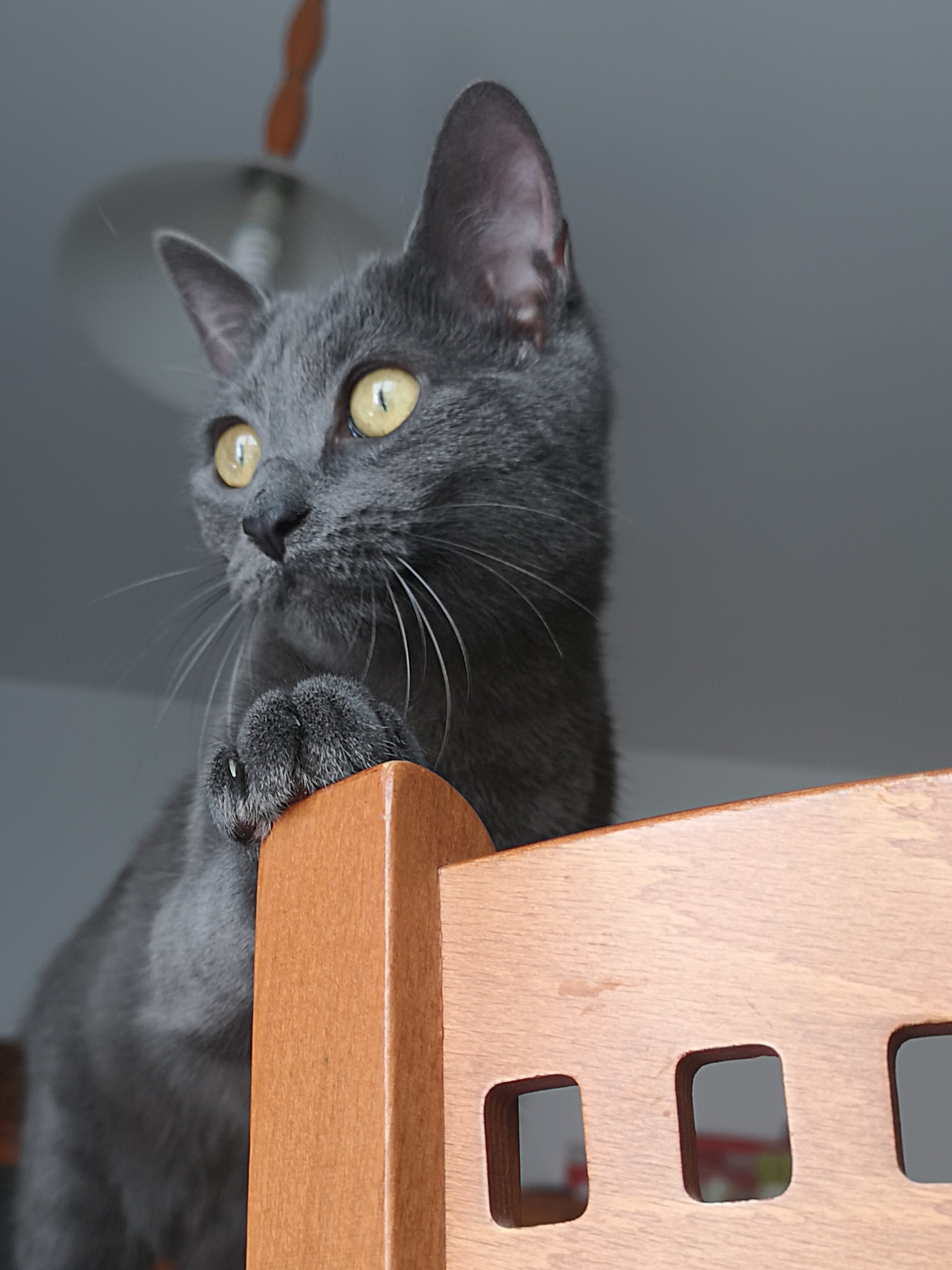 |
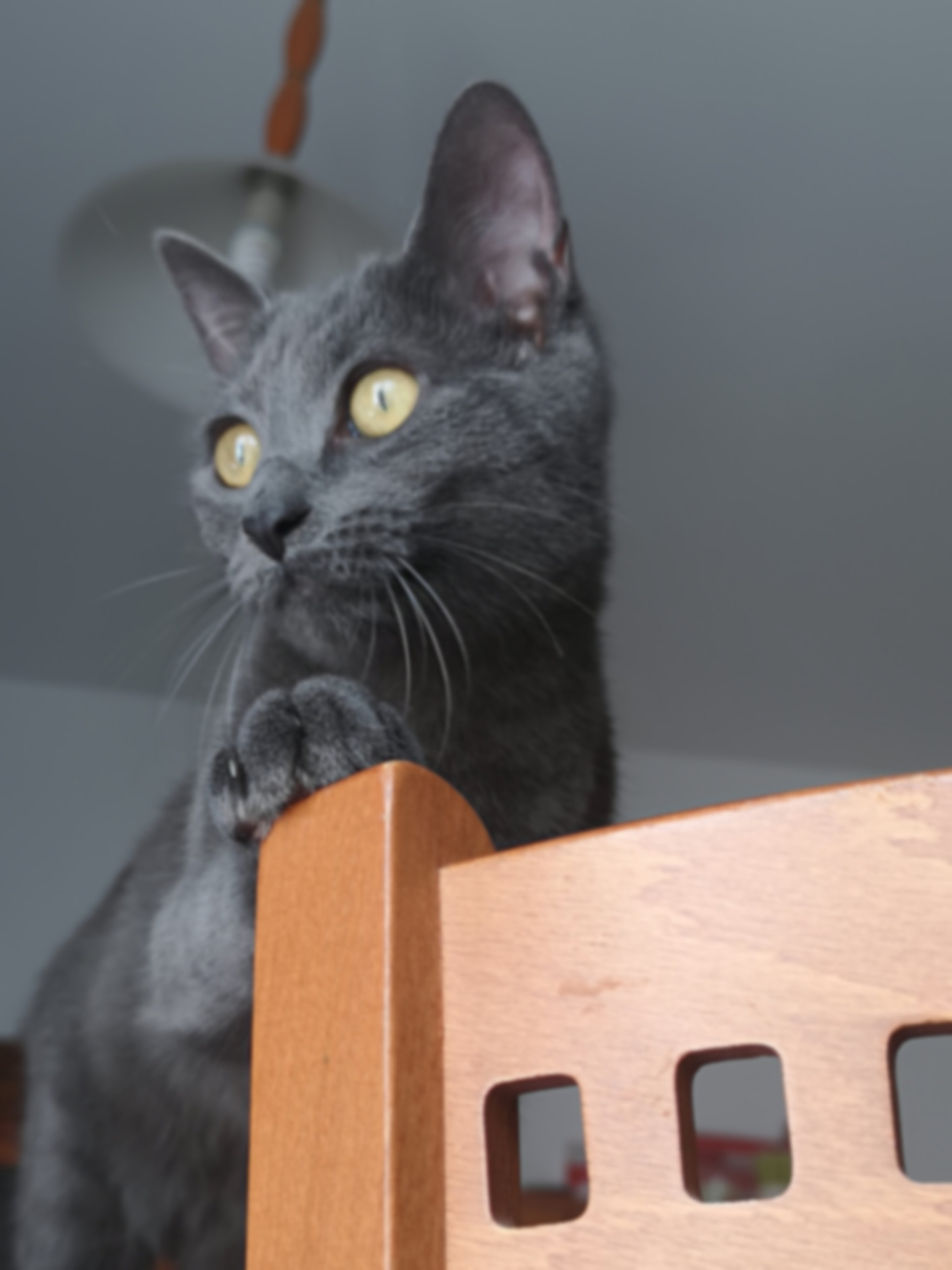 |
out_img = T.GaussianBlur(19, sigma=(2.9, 3.0))(img)
|
|
8. |
|
Converts image to grayscale.
|
PHOTOMETRY
|
Original |
Augmented |
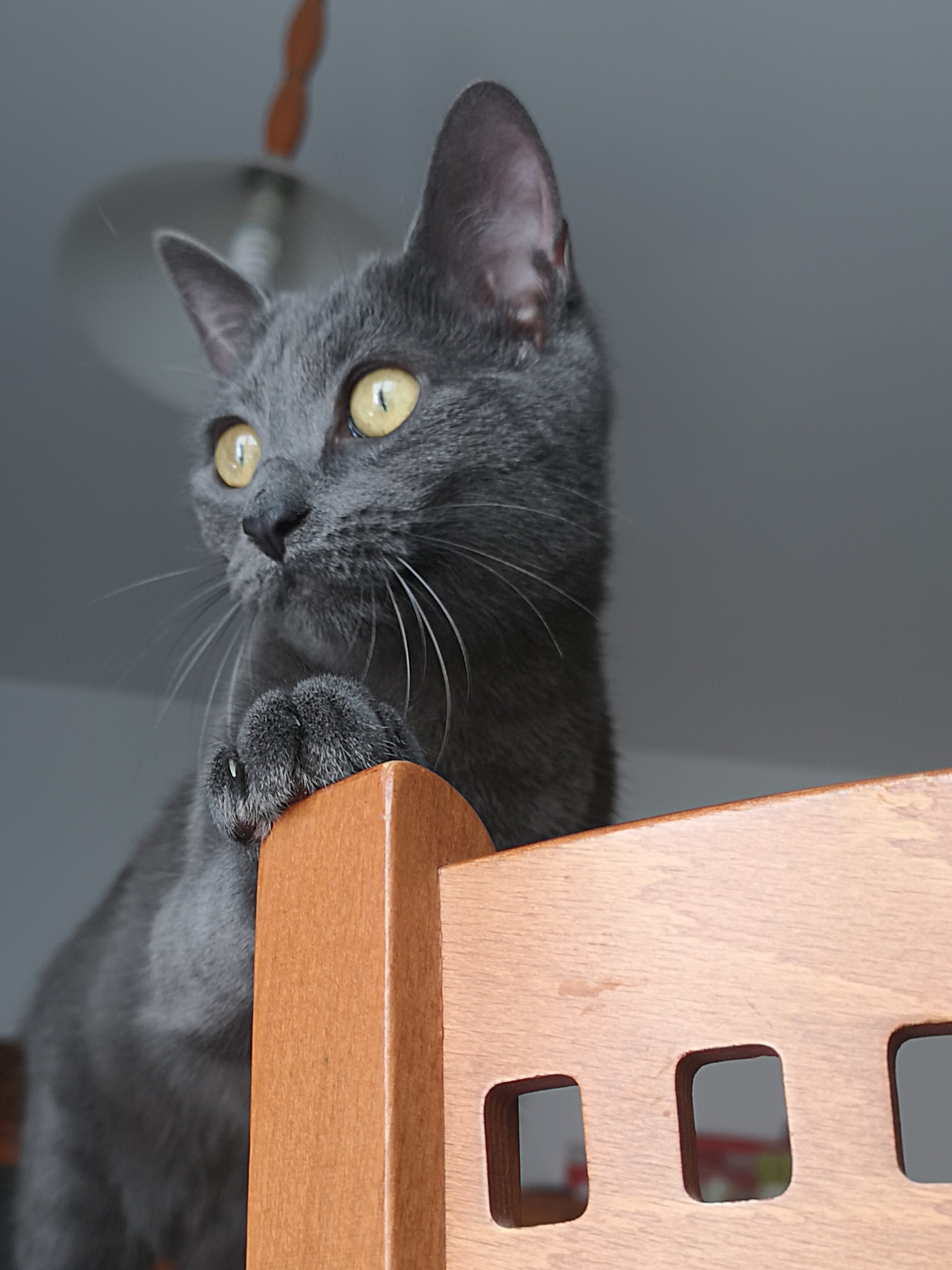 |
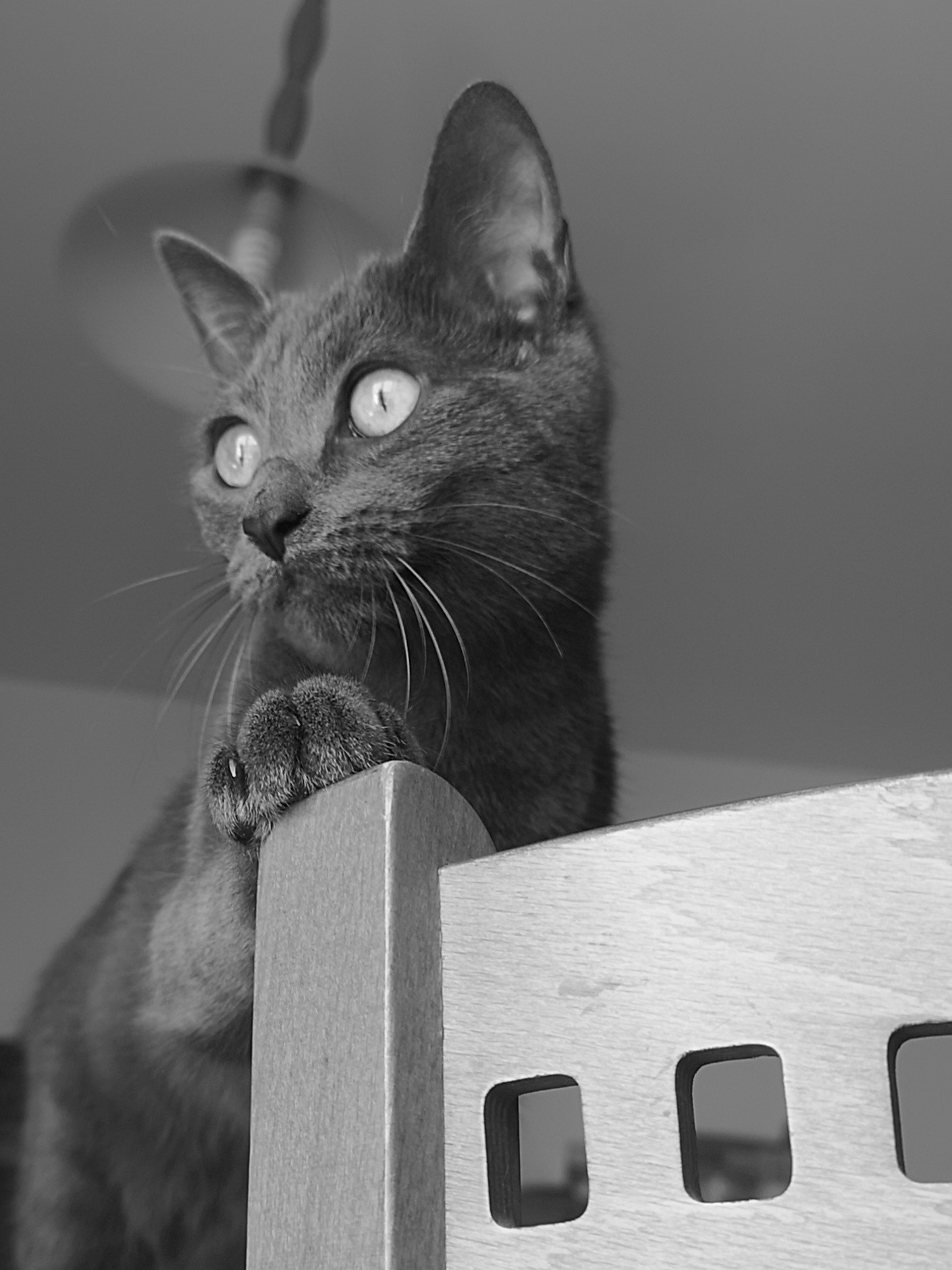 |
out_img = T.Grayscale(num_output_channels=1)(img)
|
|
9. |
|
Pads the given image on all sides with the given pad value.
|
BASIC GEOMETRY
|
Original |
Augmented |
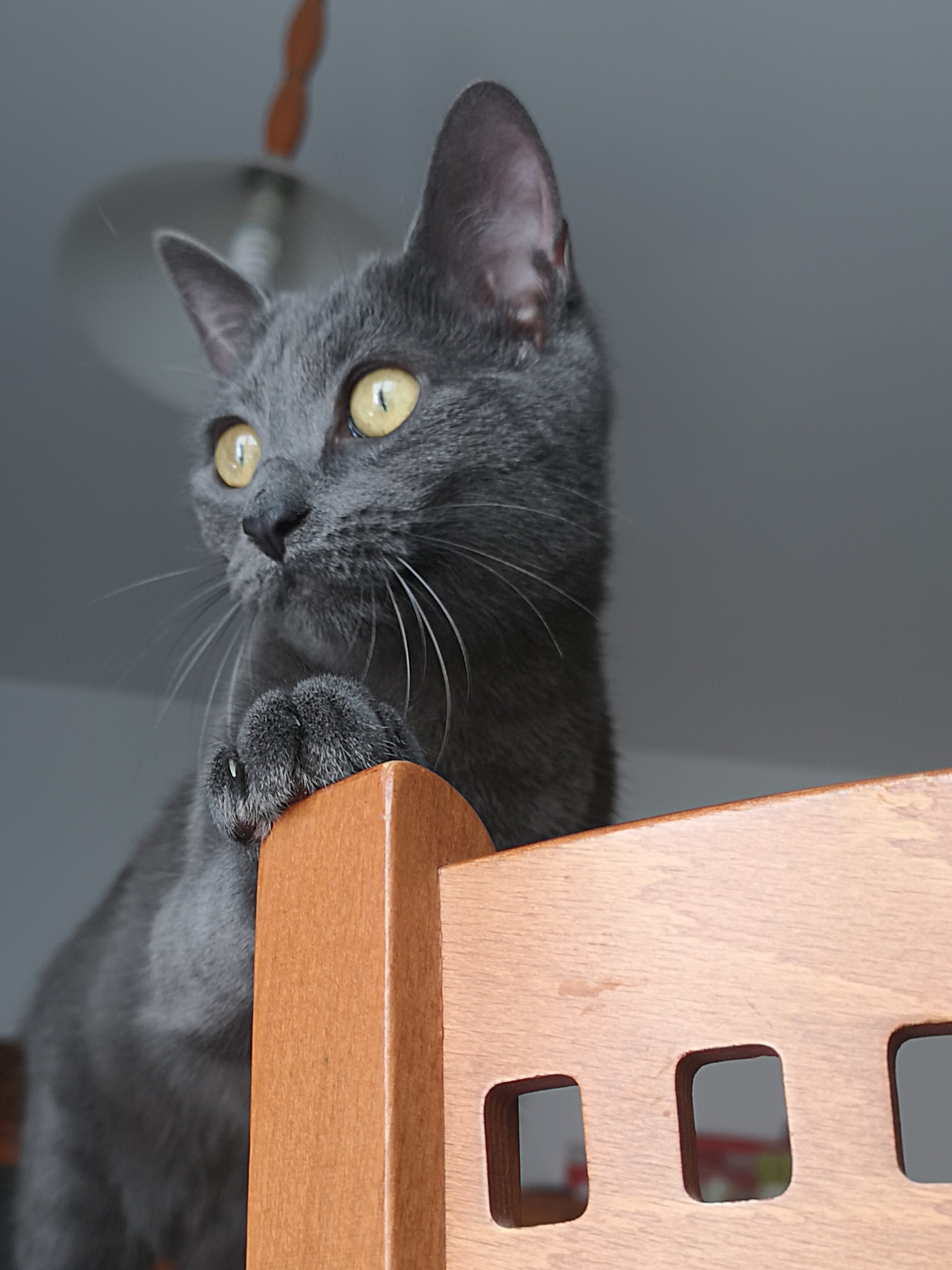 |
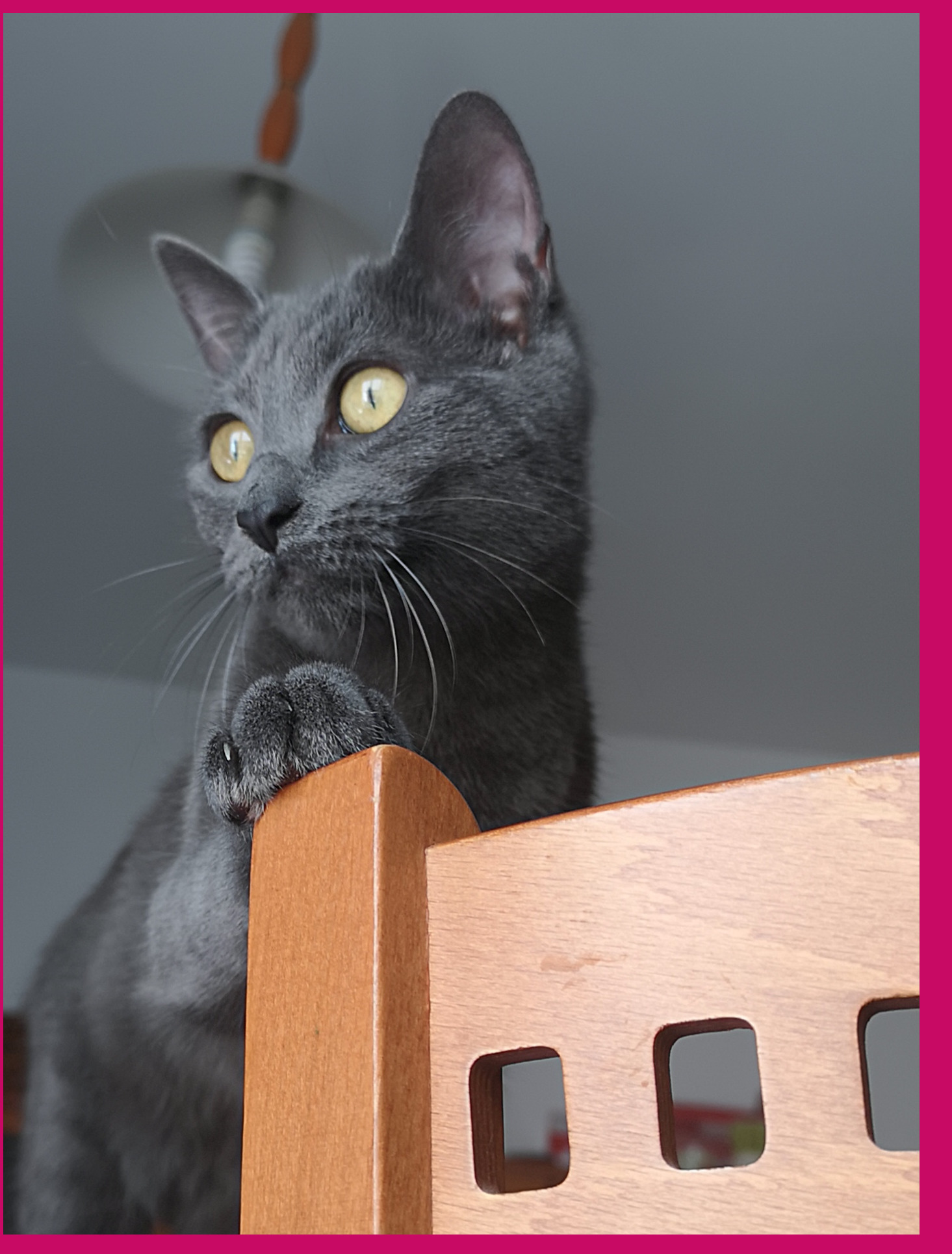 |
out_img = T.Pad((5,20,50,30), fill=(200,10,100),
padding_mode='constant')(img)
|
Original |
Augmented |
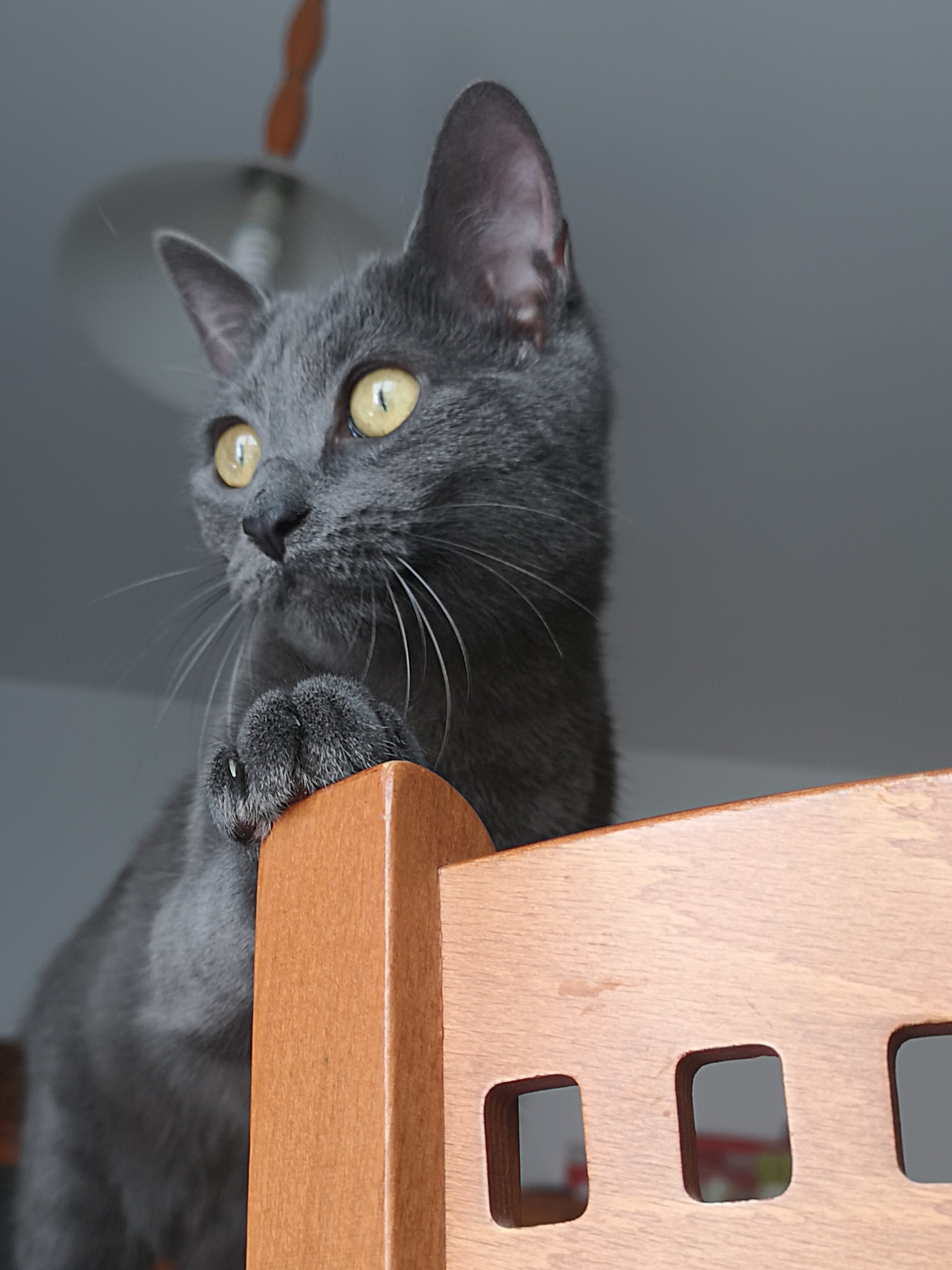 |
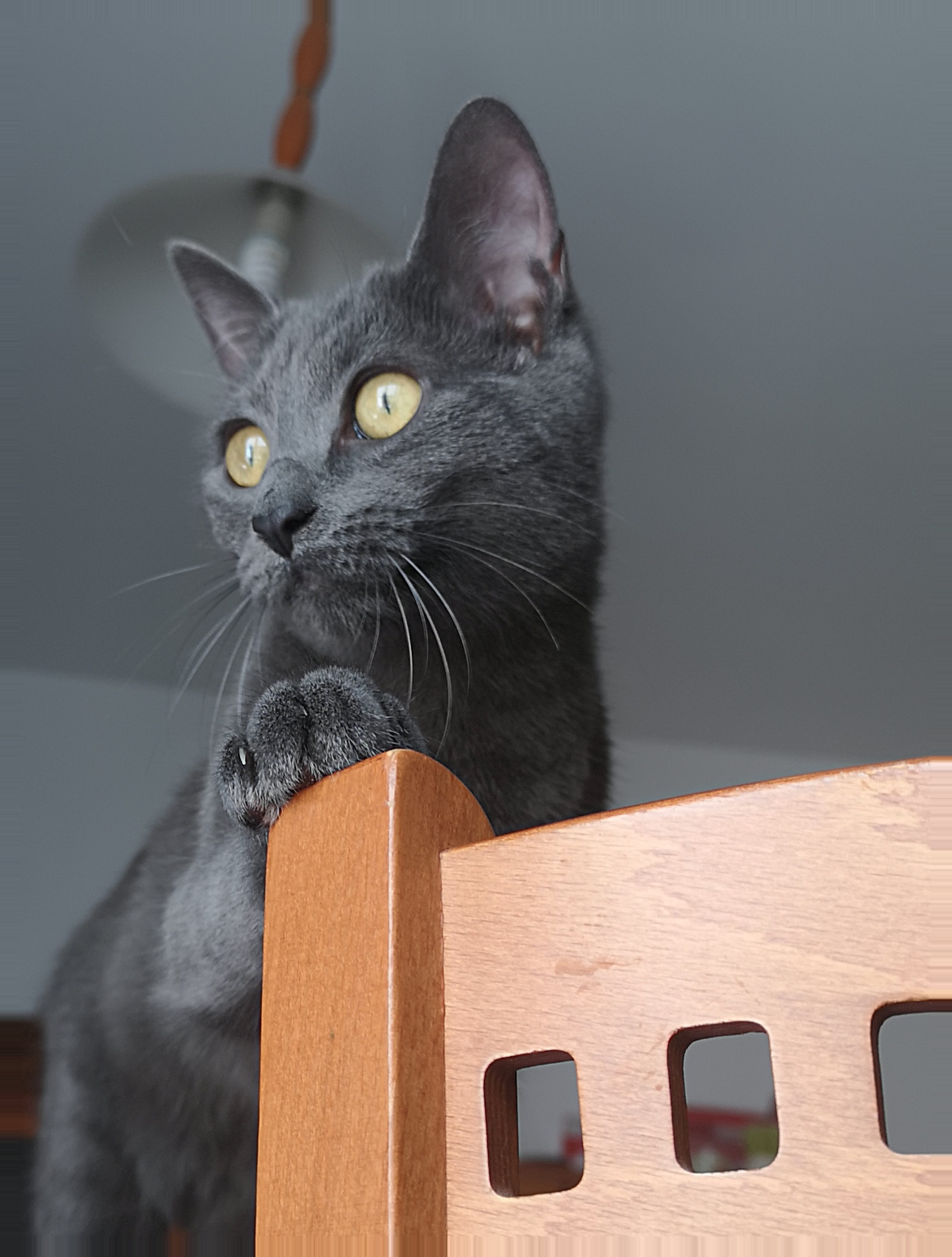 |
out_img = T.Pad(30, fill=0, padding_mode='edge')(img)
|
Original |
Augmented |
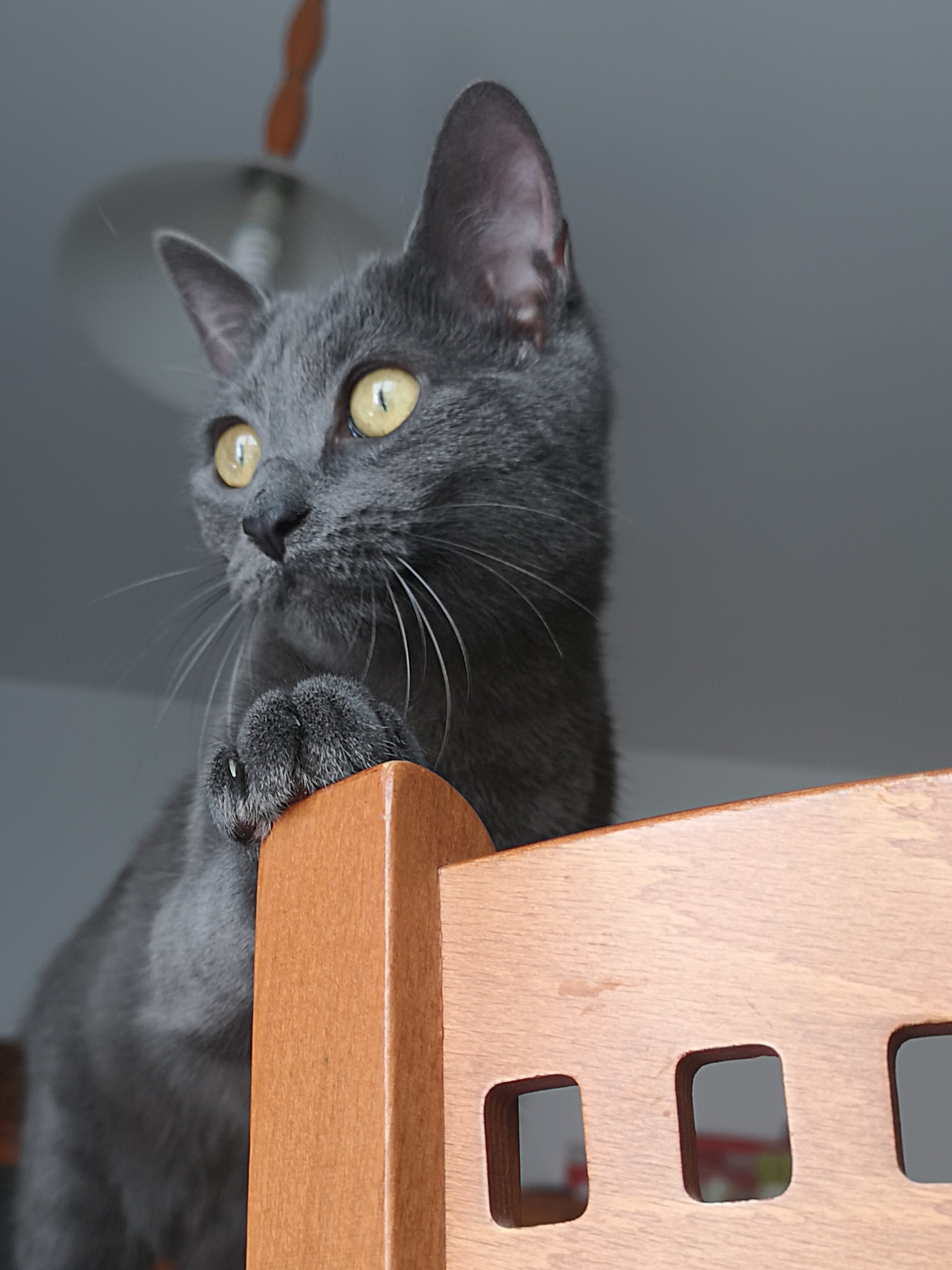 |
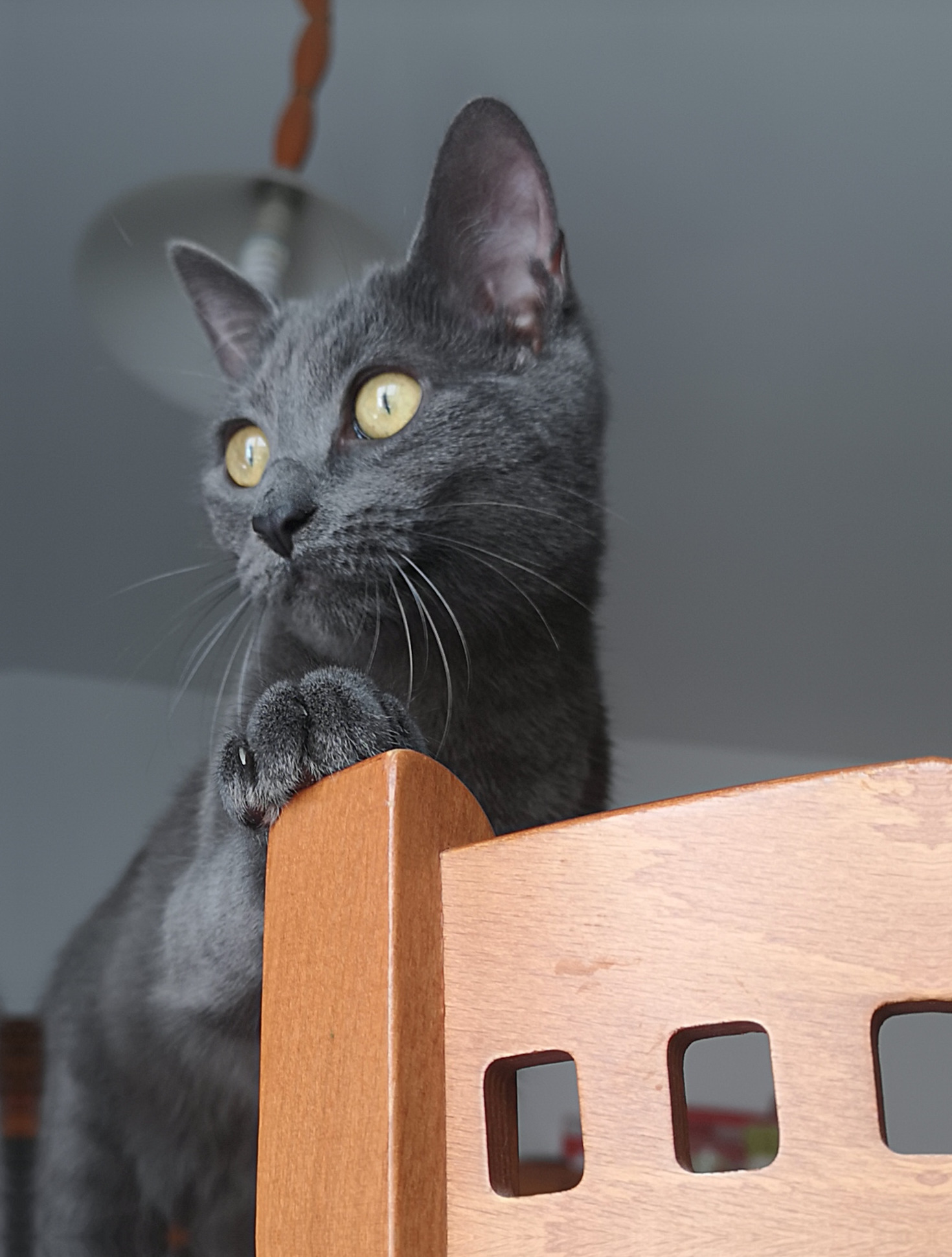 |
out_img = T.Pad(30, fill=0, padding_mode='reflect')(img)
|
|
10. |
|
Data augmentation method based on RandAugment.
|
|
Original |
Augmented |
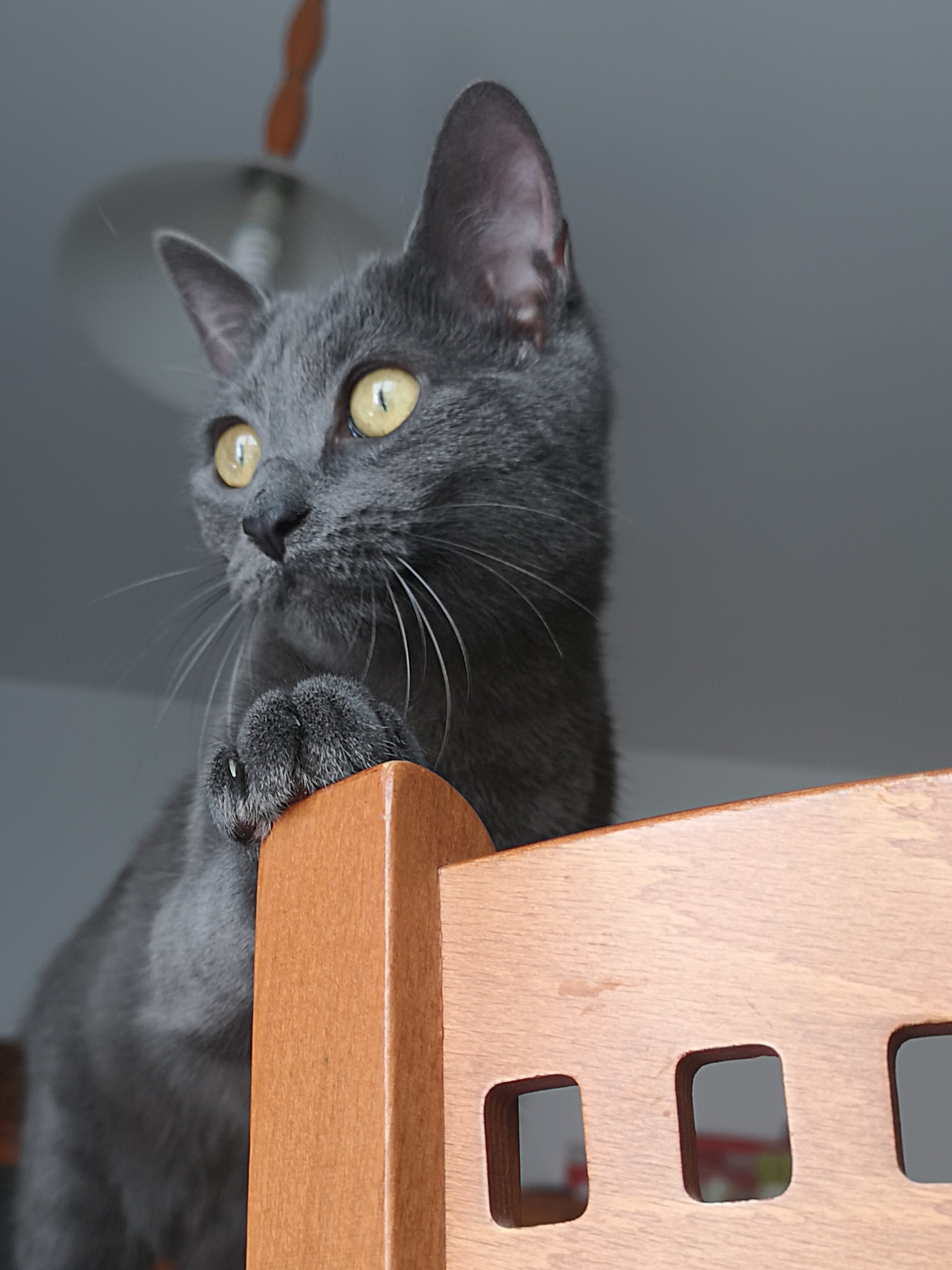 |
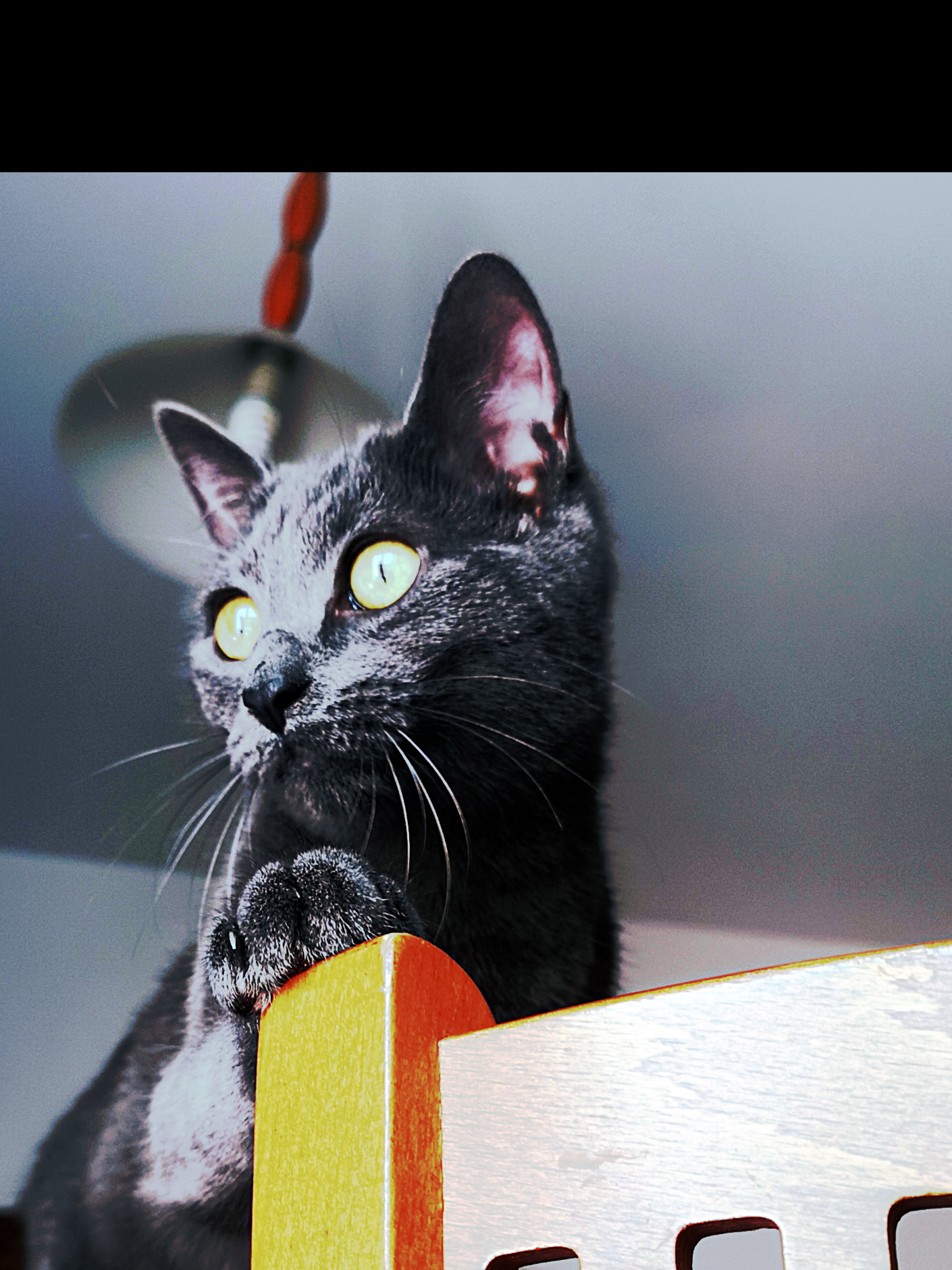 |
Original |
Augmented |
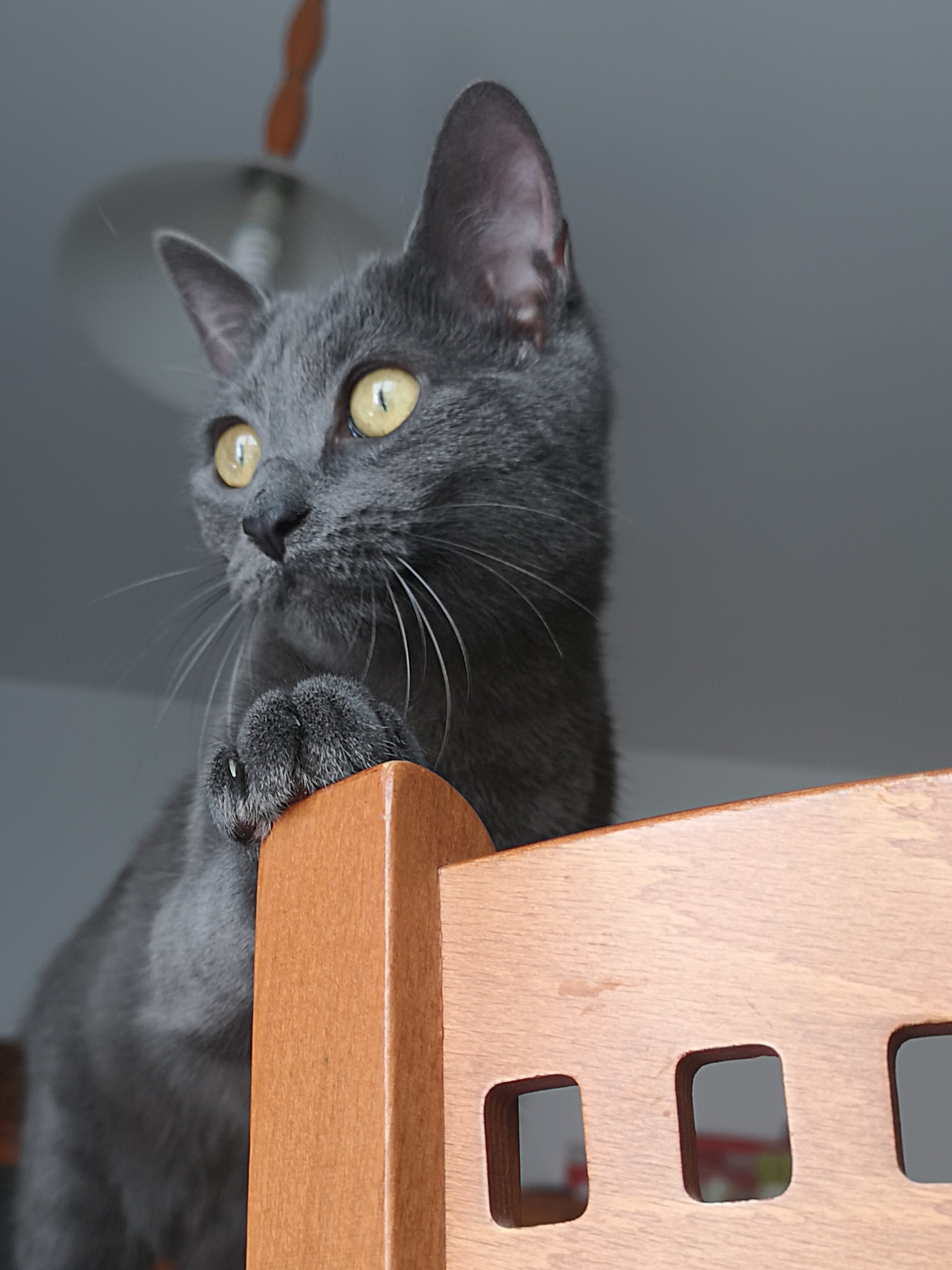 |
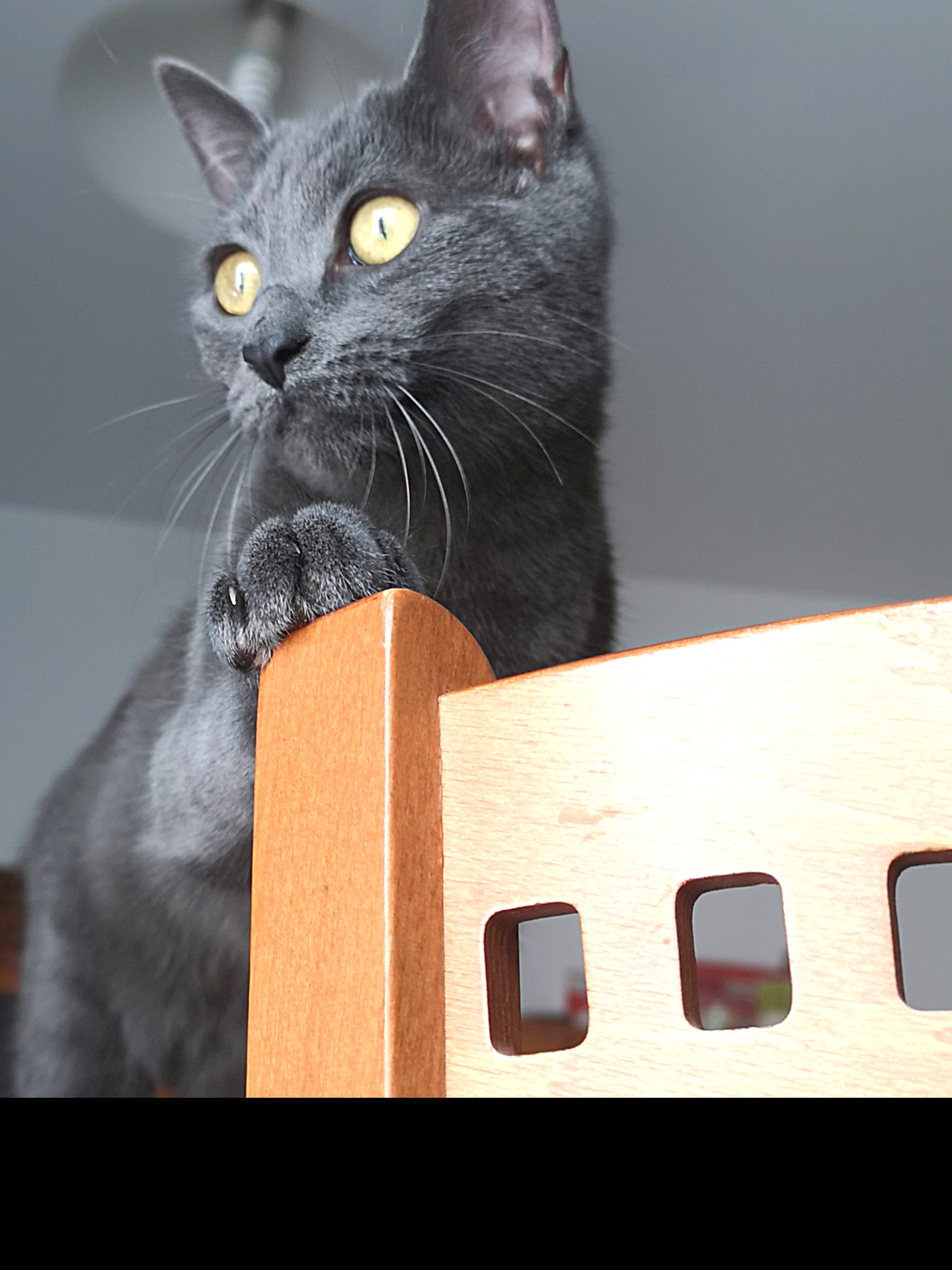 |
out_img = T.RandAugment(num_ops=2, magnitude=9, num_magnitude_bins=31,
interpolation=T.InterpolationMode.NEAREST, fill=None)(img)
|
|
11. |
|
Adjusts the sharpness of the image randomly with a given probability.
|
SHARPNESS
|
Original |
Augmented |
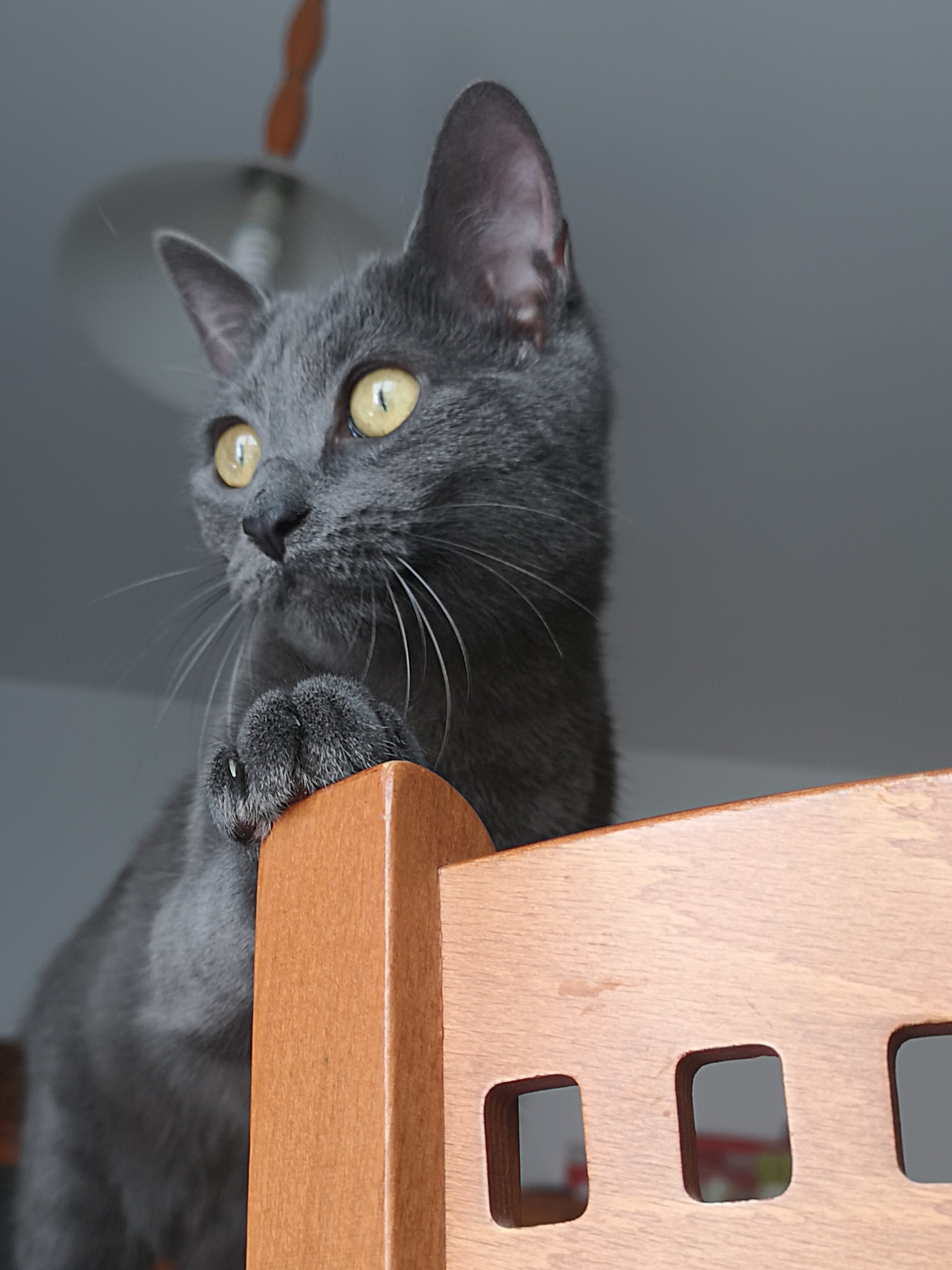 |
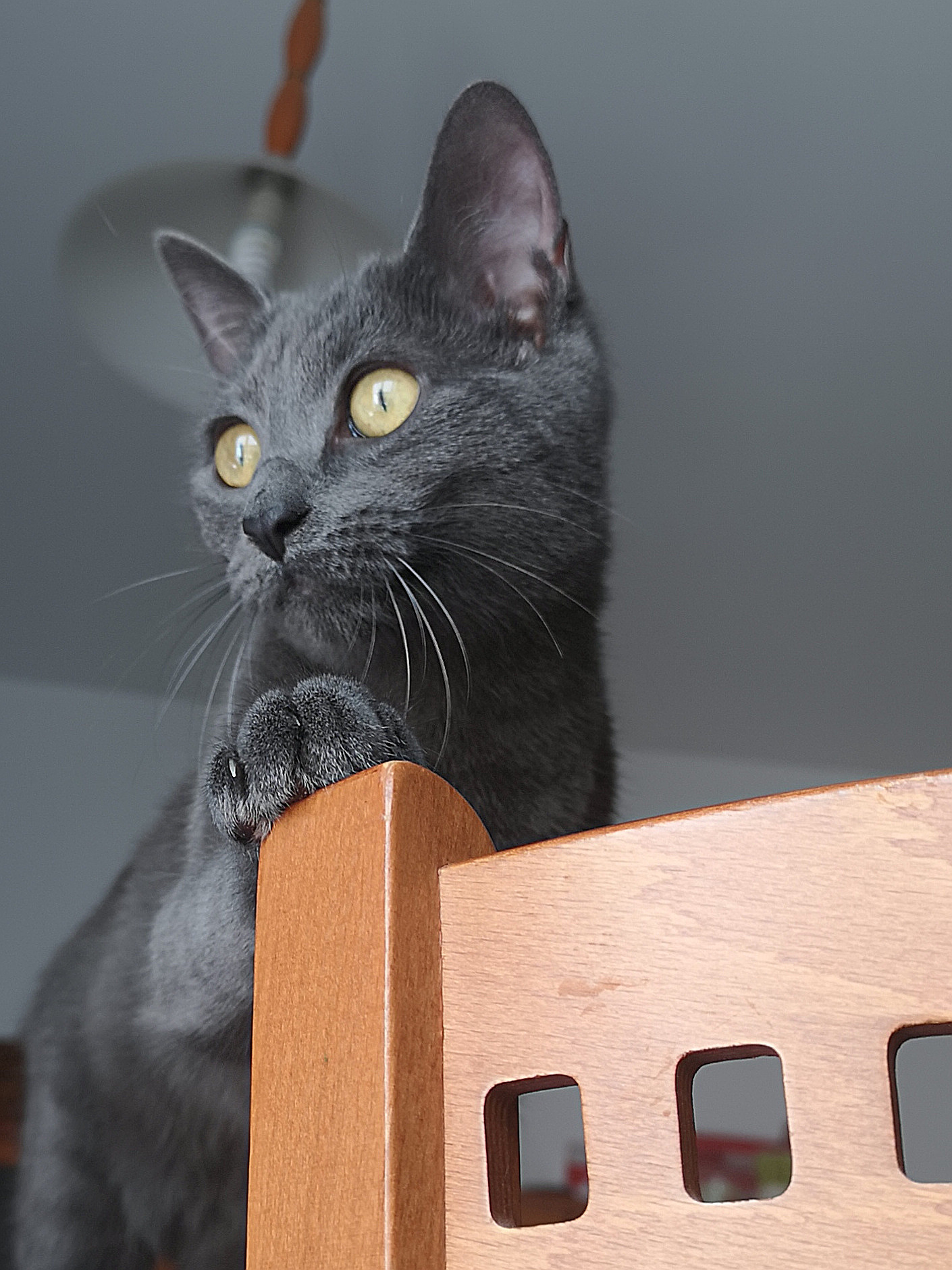 |
out_img = T.RandomAdjustSharpness(4, p=1)(img)
|
|
12. |
|
Performs a random affine transformation of the image keeping center invariant.
|
BASIC GEOMETRY
|
Original |
Augmented |
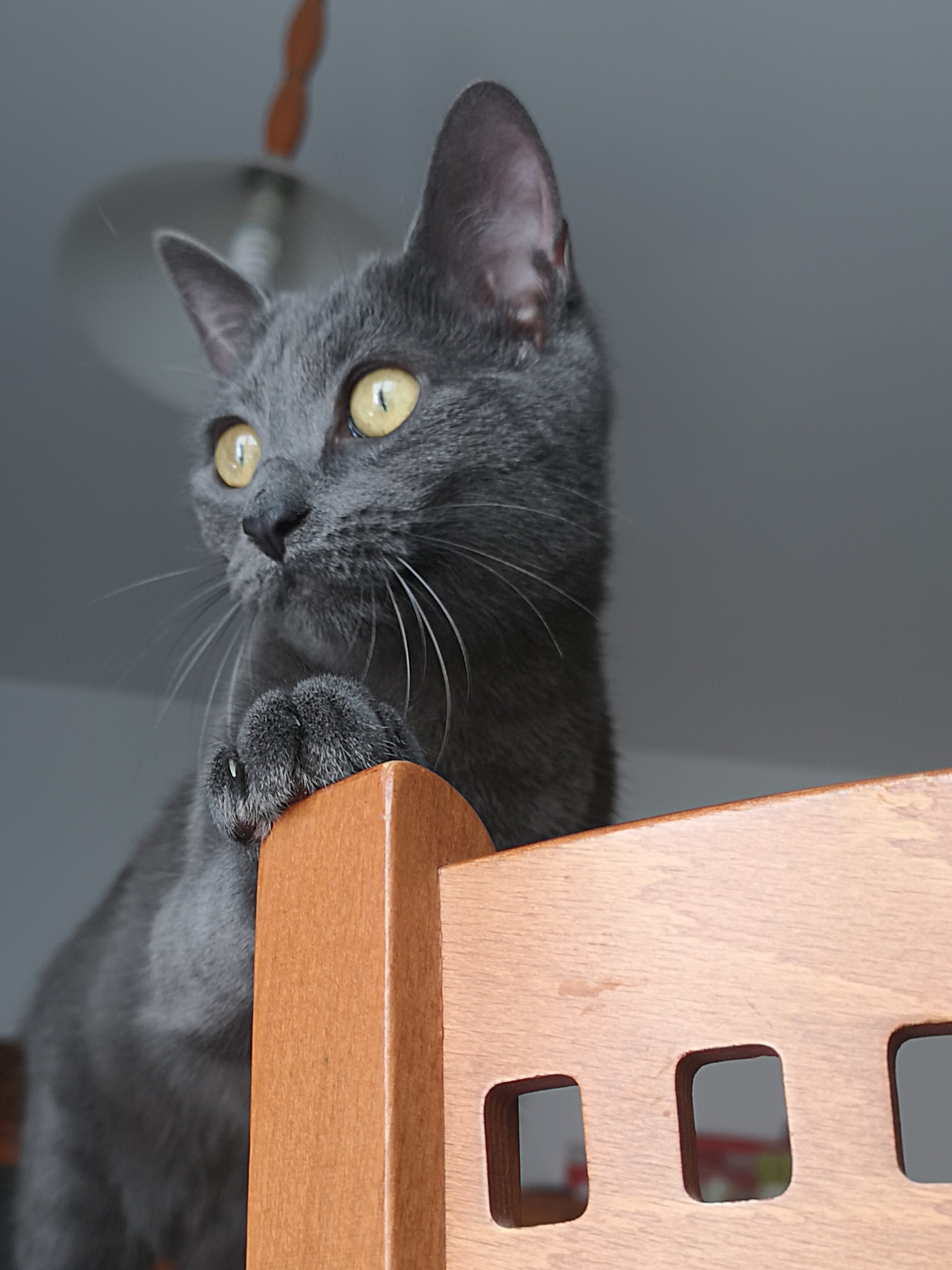 |
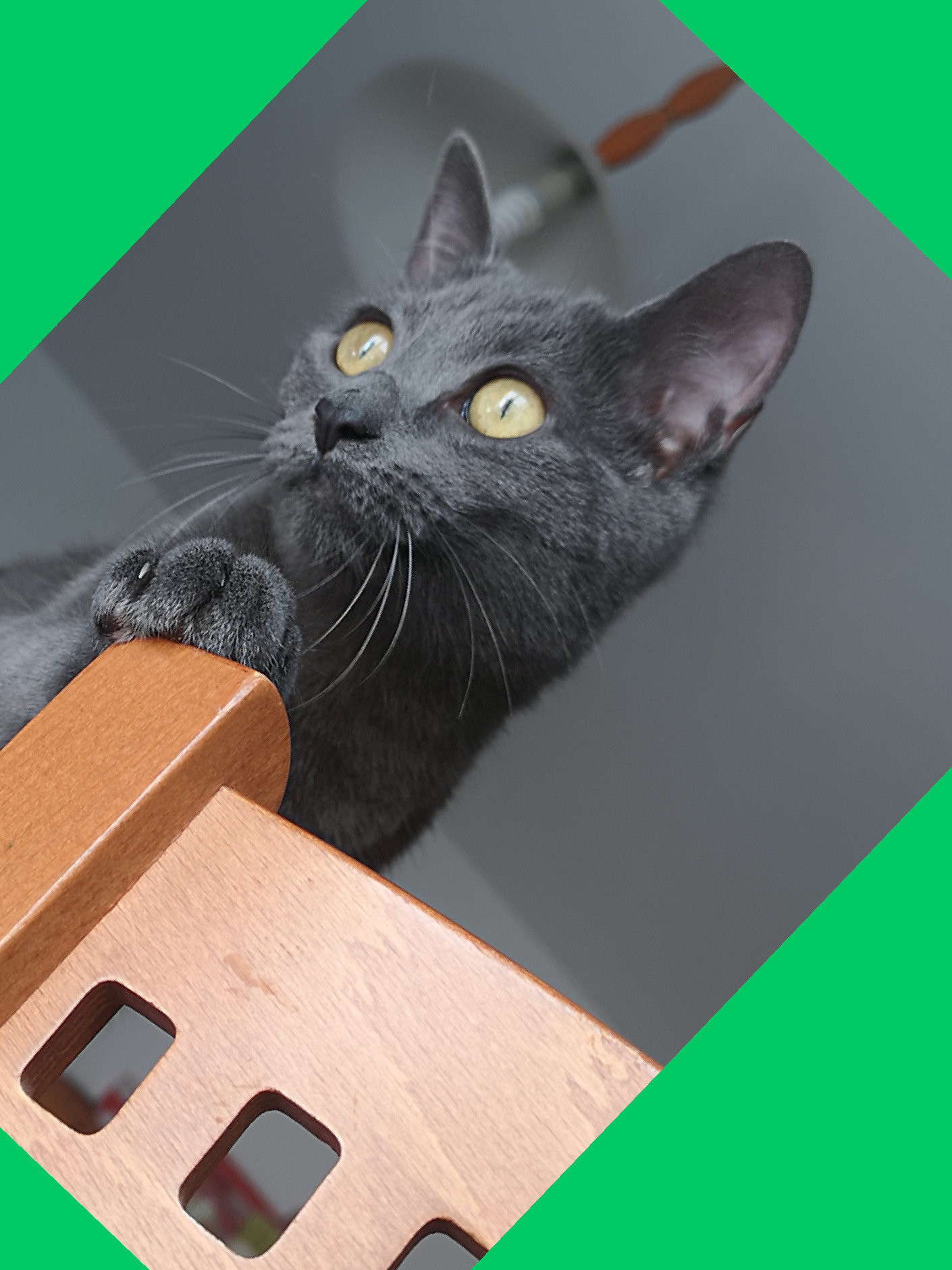 |
out_img = T.RandomAffine(45, translate=(0.1,0.5), scale=None, shear=None,
interpolation=T.InterpolationMode.NEAREST,
fill=(0,200,100), center=None)(img)
|
Original |
Augmented |
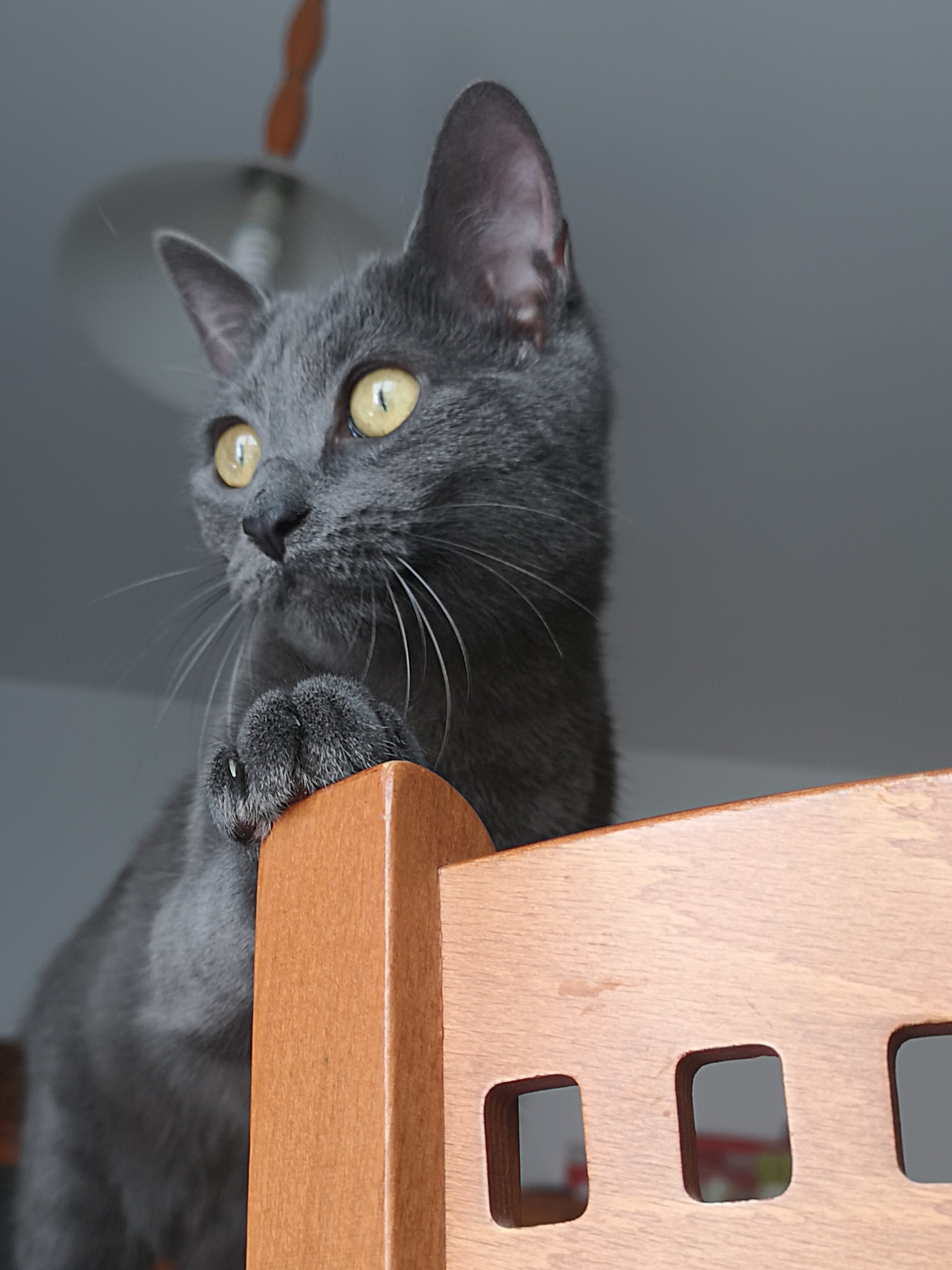 |
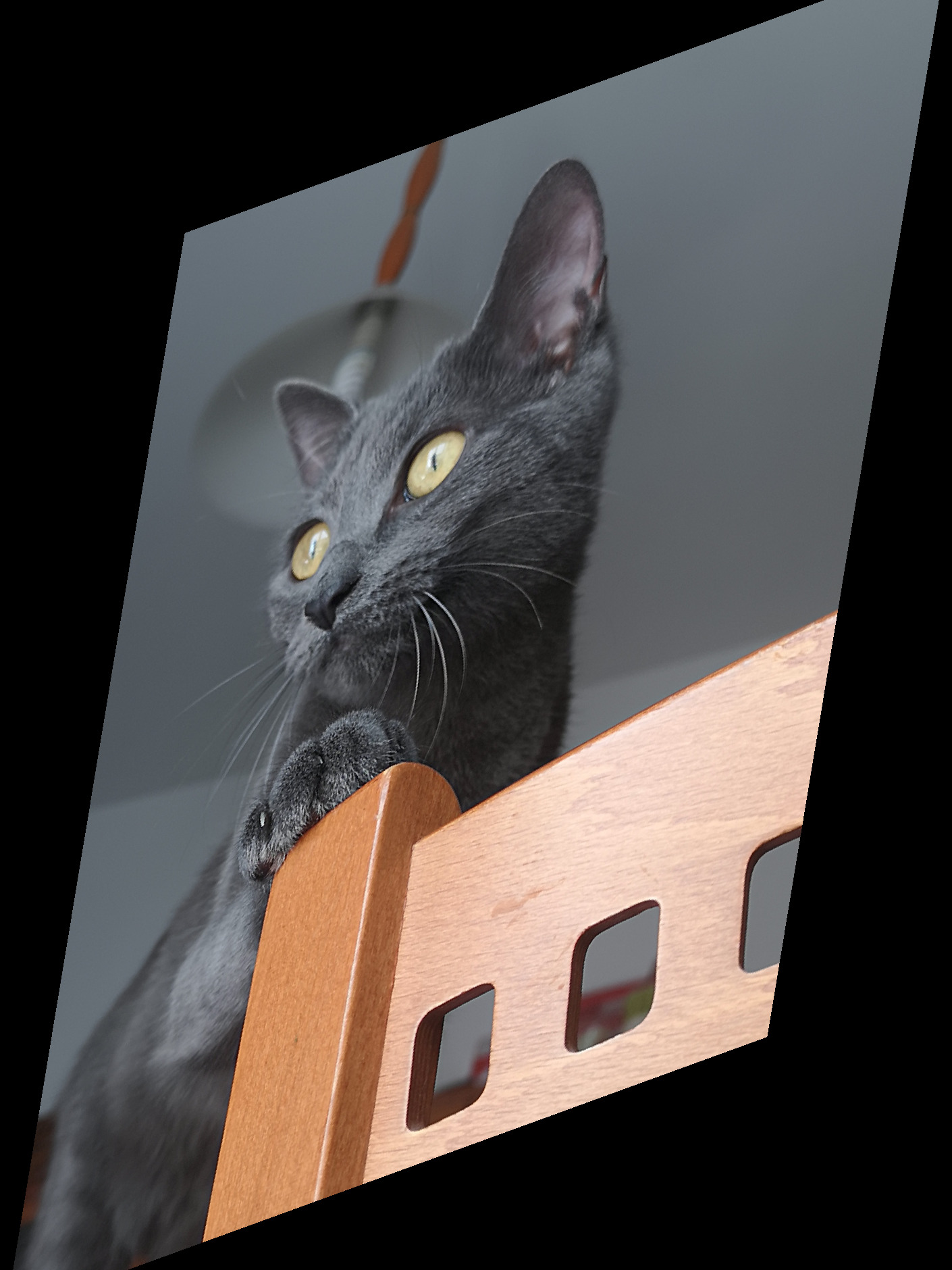 |
out_img = T.RandomAffine(0, translate=None, scale=(0.8,0.8),
shear=(10,10,20,20),
interpolation=T.InterpolationMode.NEAREST,
fill=0, center=None)(img)
|
|
13. |
|
Applies randomly a list of transformations with a given probability.
|
|
14. |
|
Autocontrasts the pixels of the given image randomly with a given probability.
|
PHOTOMETRY
|
Original |
Augmented |
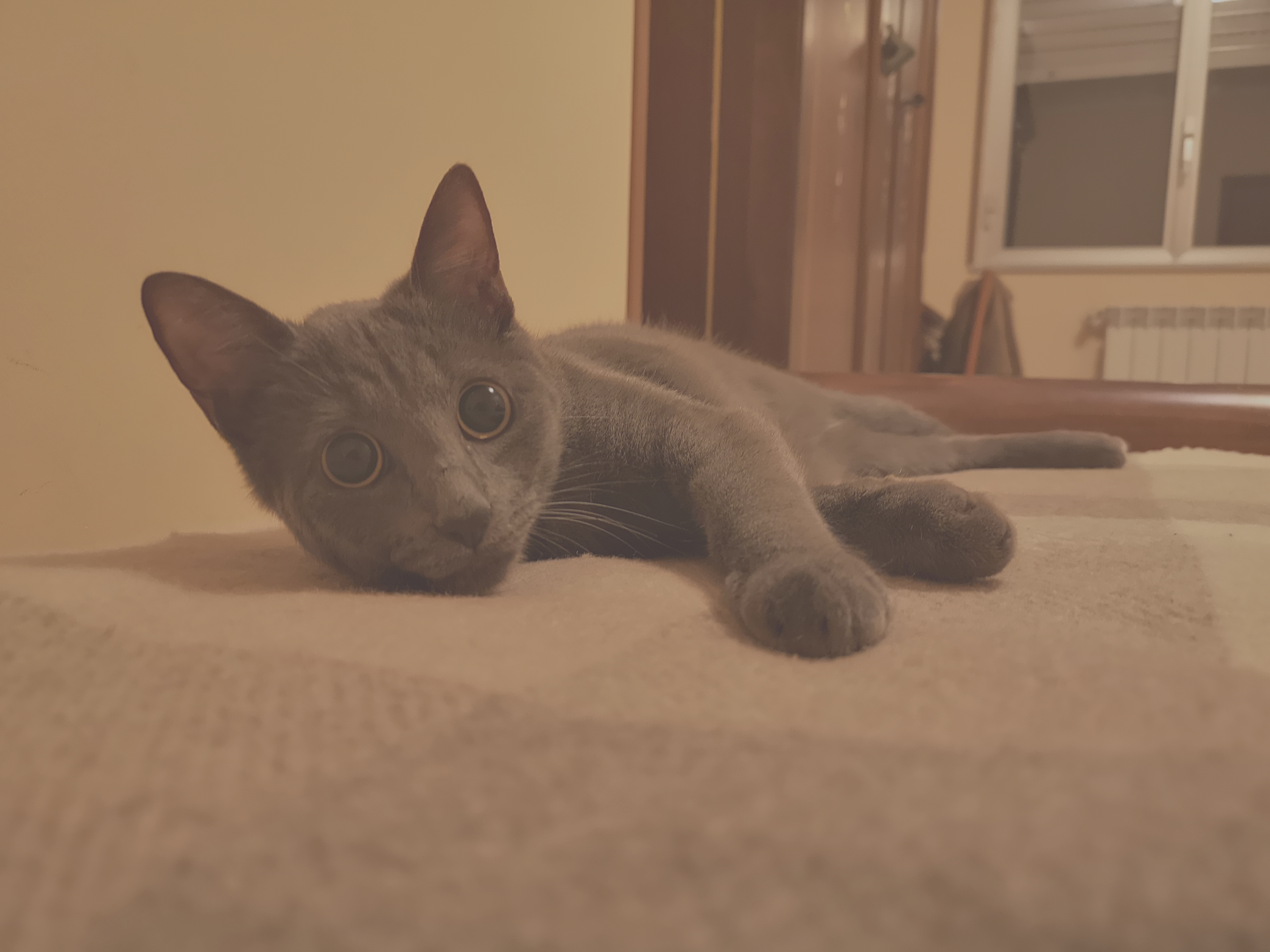 |
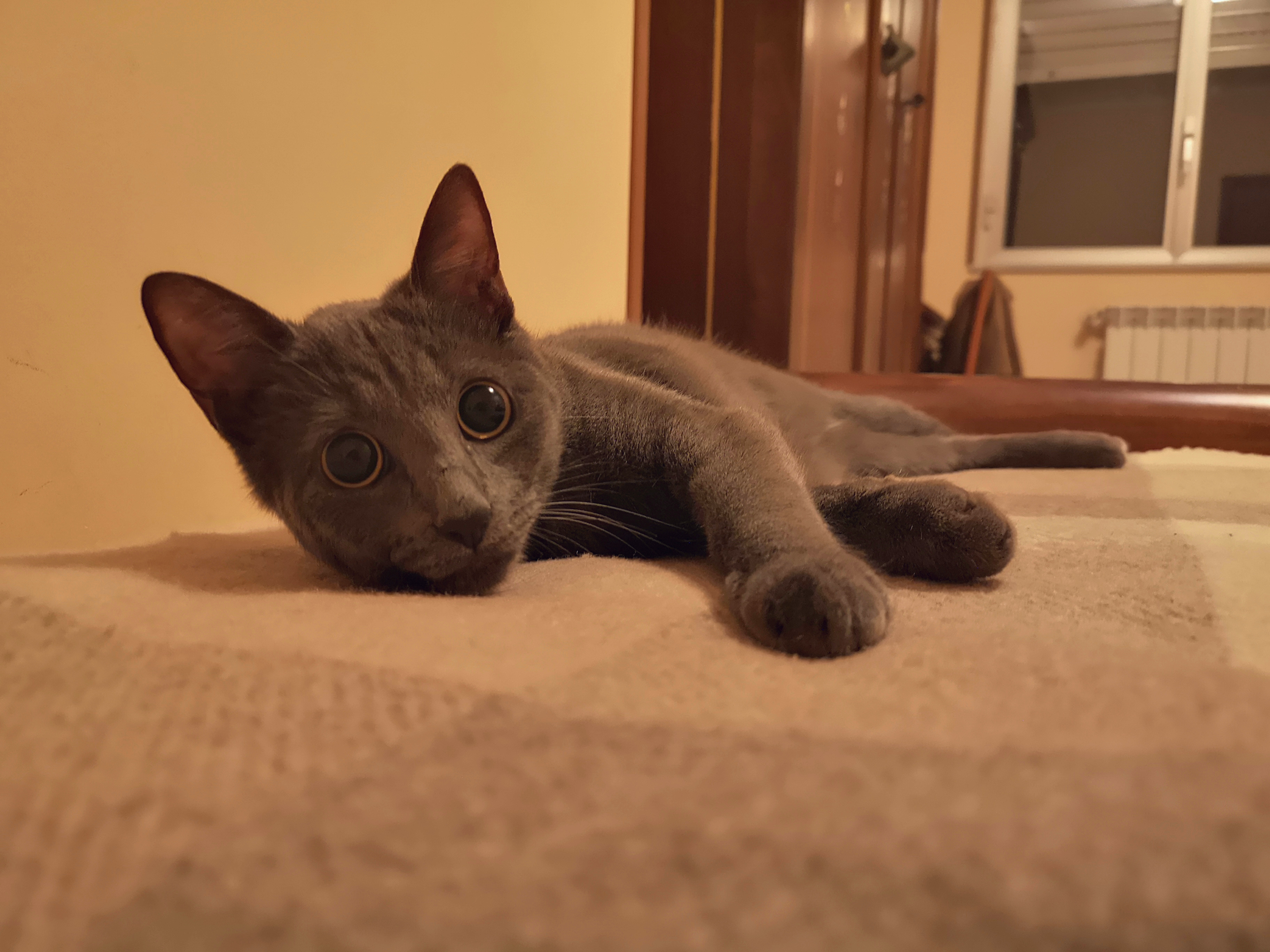 |
out_img = T.RandomAutocontrast(p=1)(img)
print(img.histogram() == out_img.histogram())
# >> False
|
Original |
Augmented |
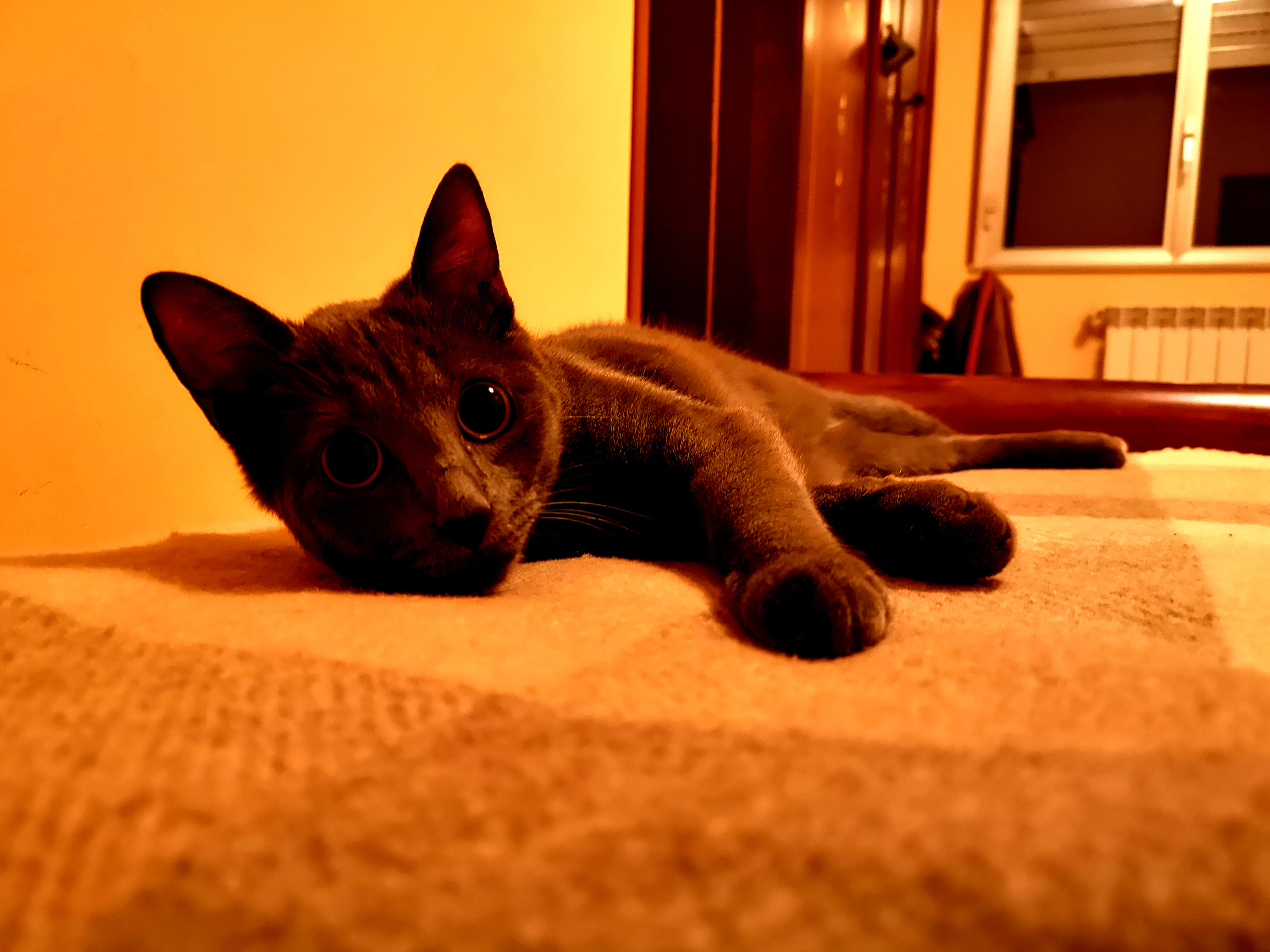 |
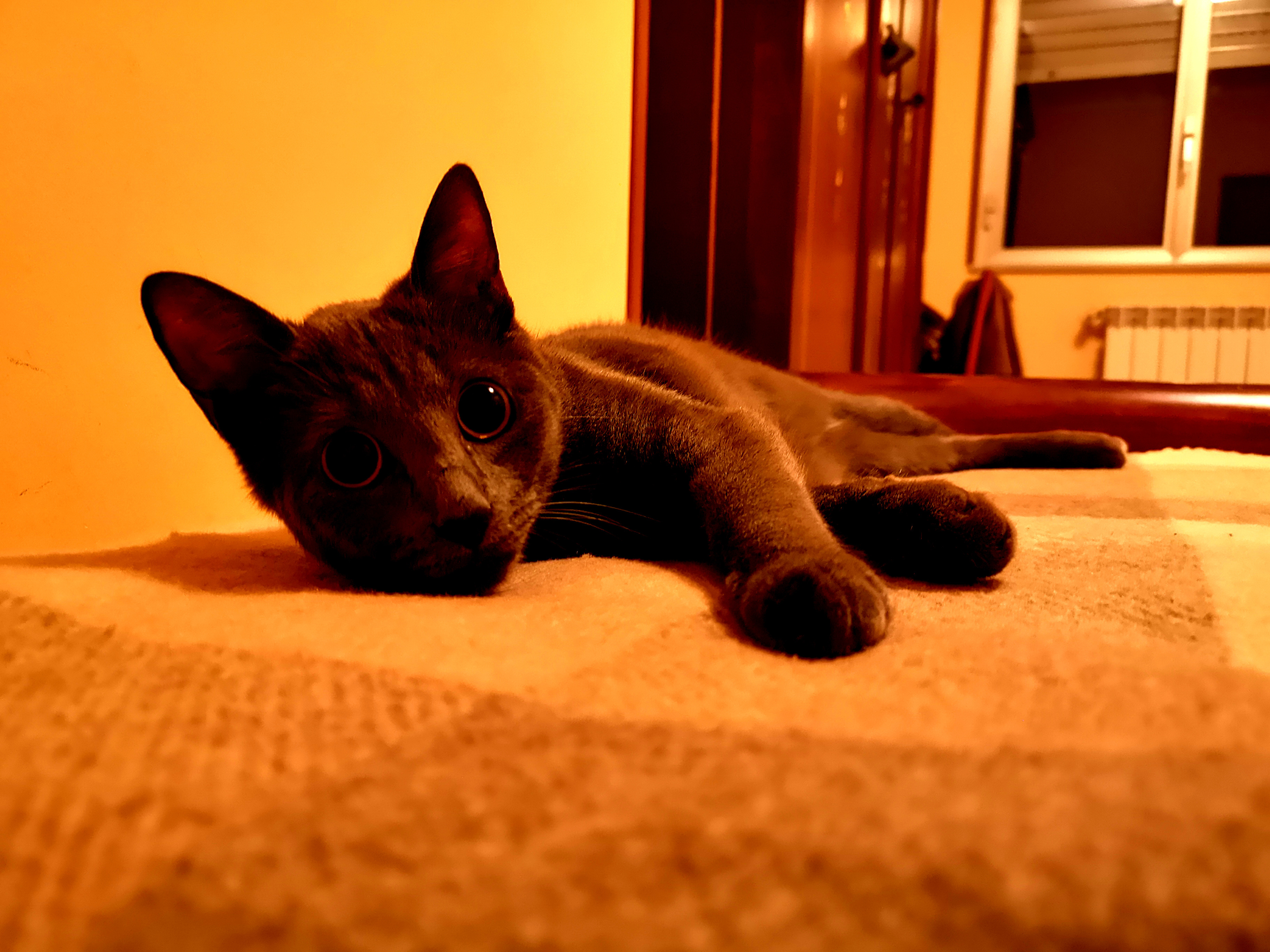 |
out_img = T.RandomAutocontrast(p=1)(img)
print(img.histogram() == out_img.histogram())
# >> True
|
|
15. |
|
Applies single transformation randomly picked from a list.
|
|
16. |
|
Crops the given image at a random location.
|
BASIC GEOMETRY
|
Original |
Augmented |
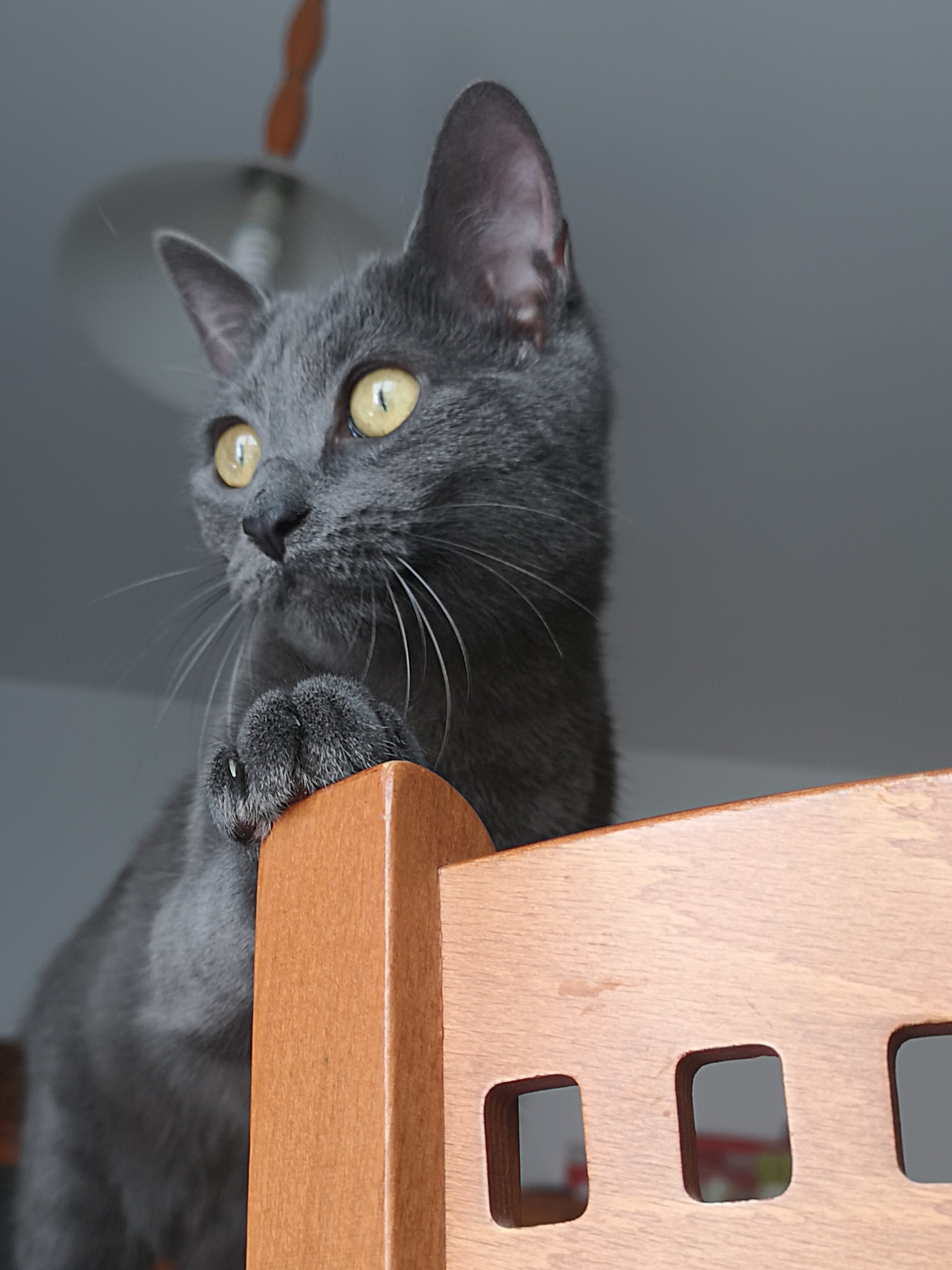 |
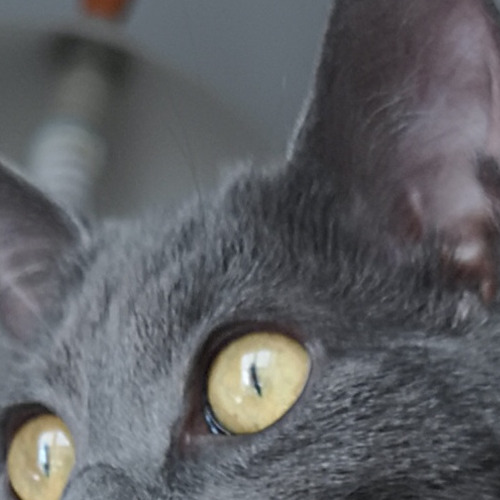 |
out_img = T.RandomCrop(500, padding=None, pad_if_needed=False,
fill=0, padding_mode='constant')(img)
|
|
17. |
|
Equalizes the histogram of the given image randomly with a given probability.
|
PHOTOMETRY
|
Original |
Augmented |
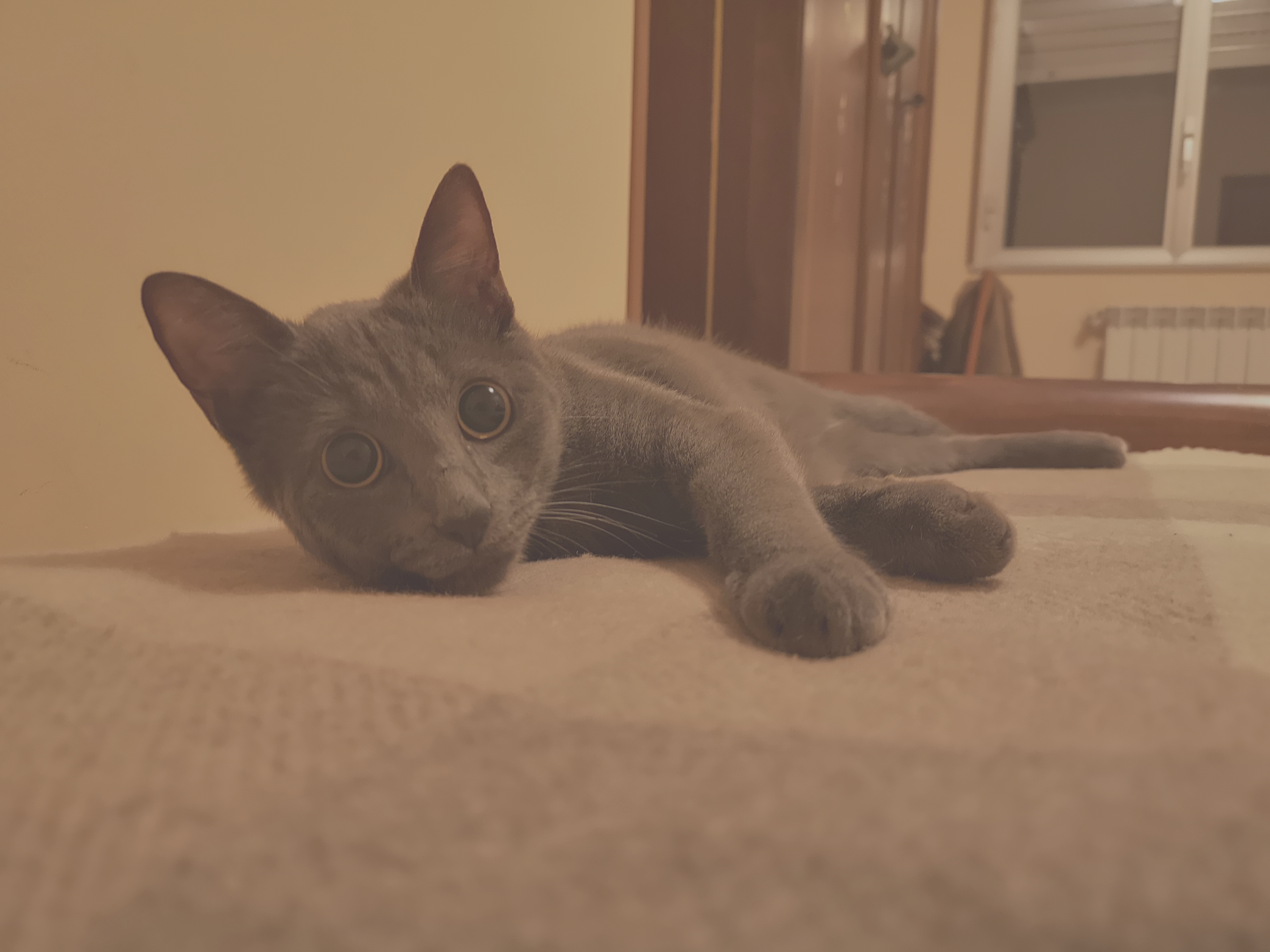 |
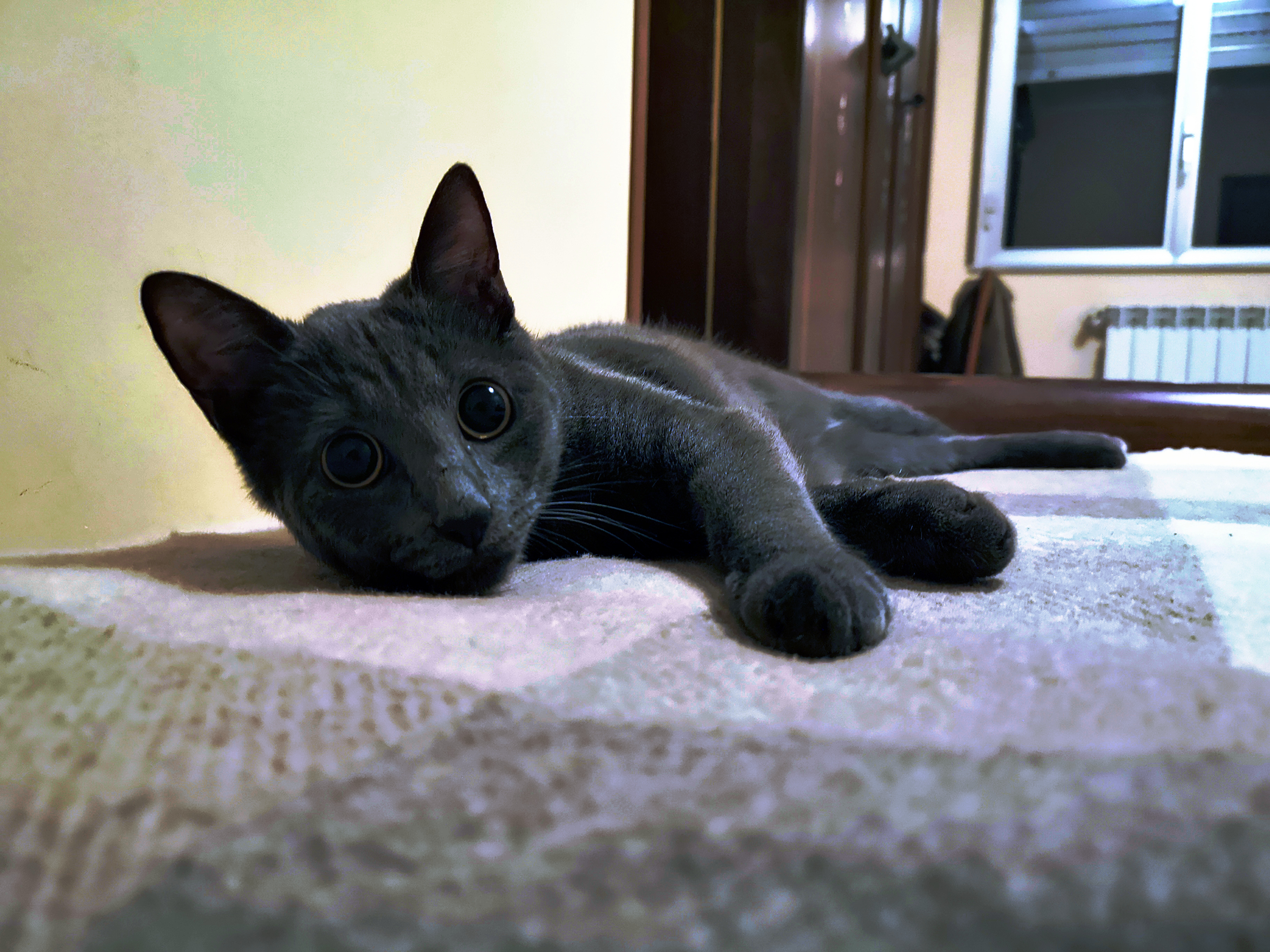 |
Original |
Augmented |
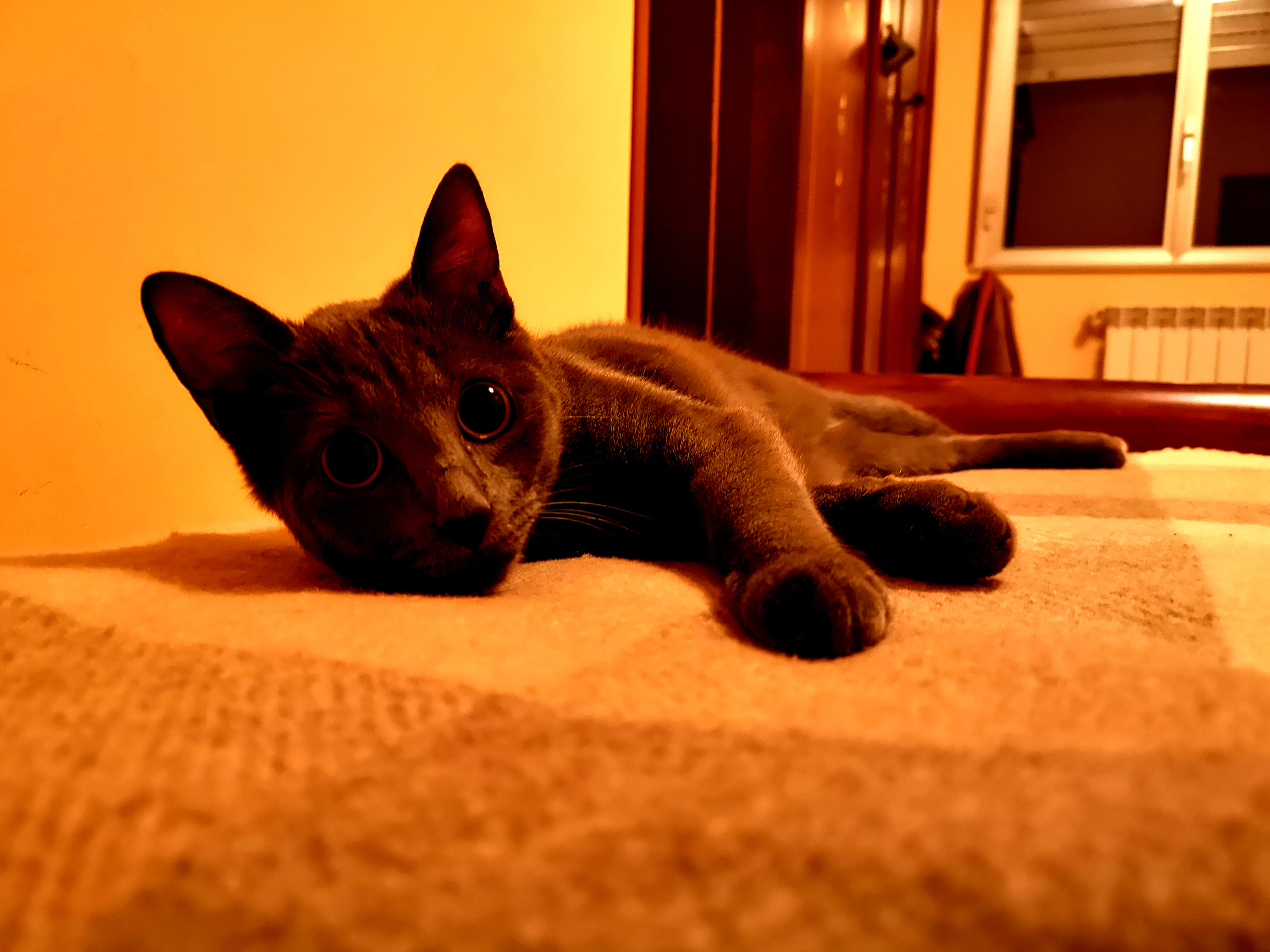 |
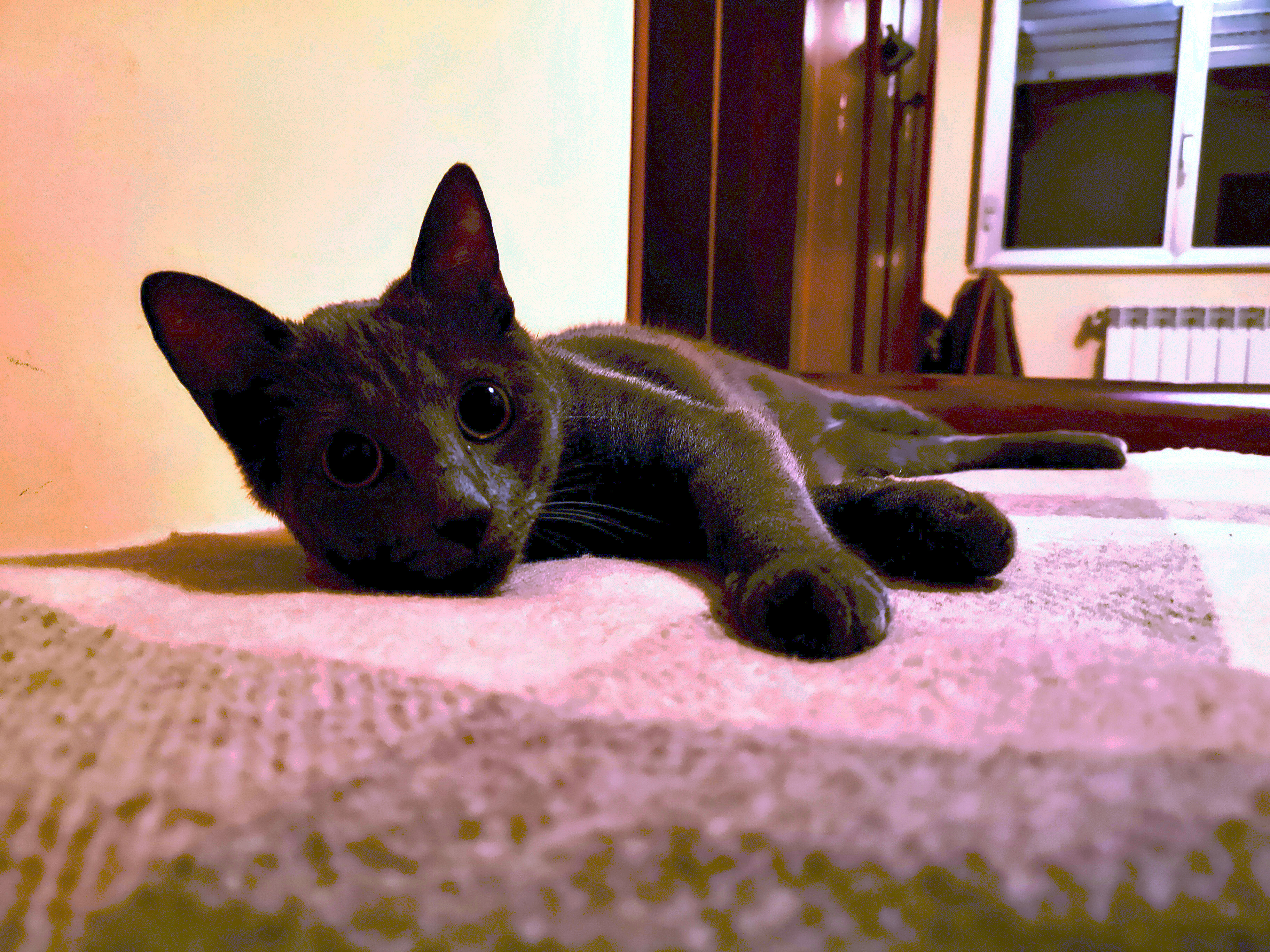 |
print(img.histogram() == out_img.histogram())
|
|
18. |
|
Randomly selects a rectangle region in a torch.Tensor image and erases its pixels. See here.
|
DROPOUT
|
Original |
Augmented |
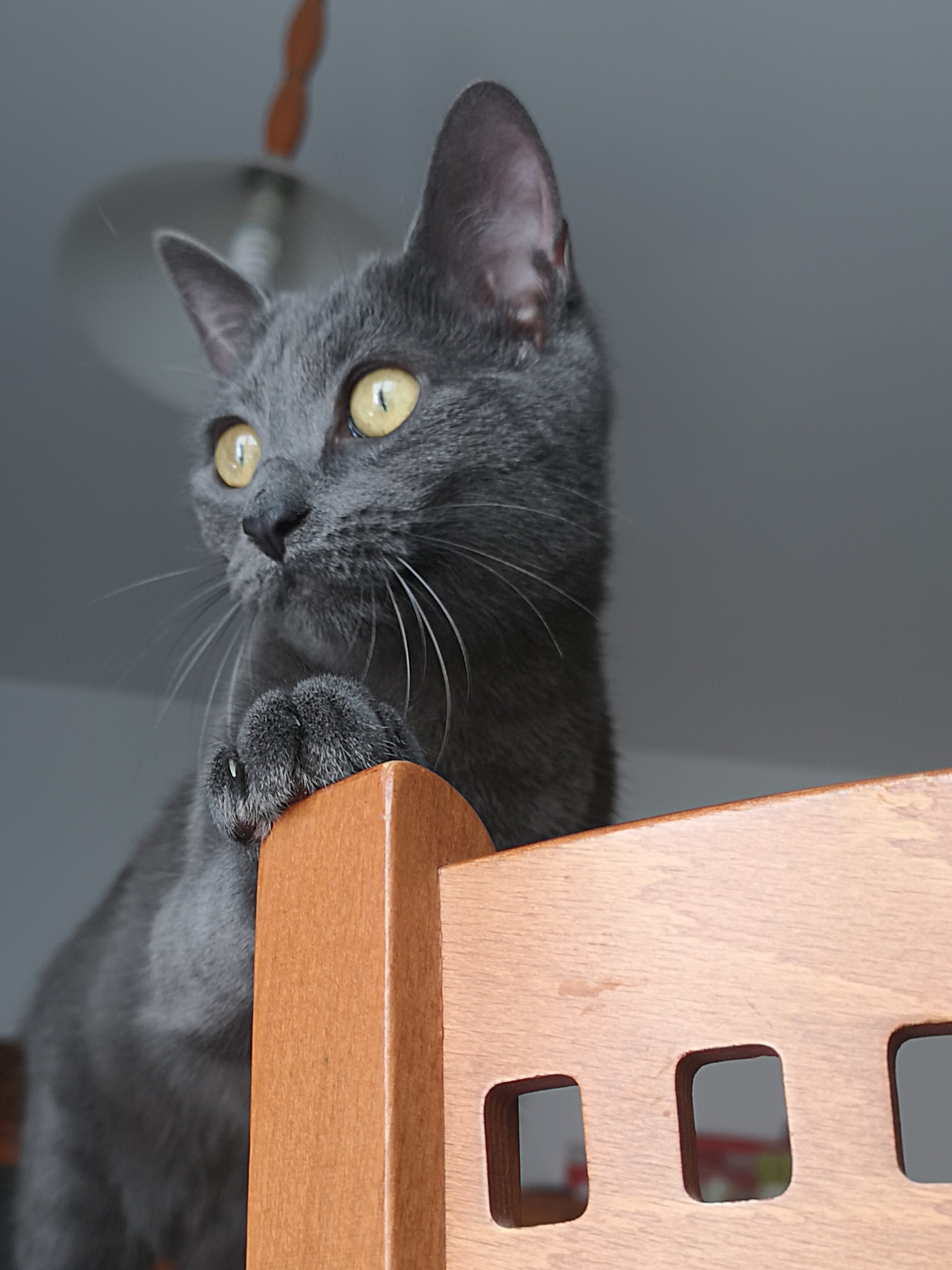 |
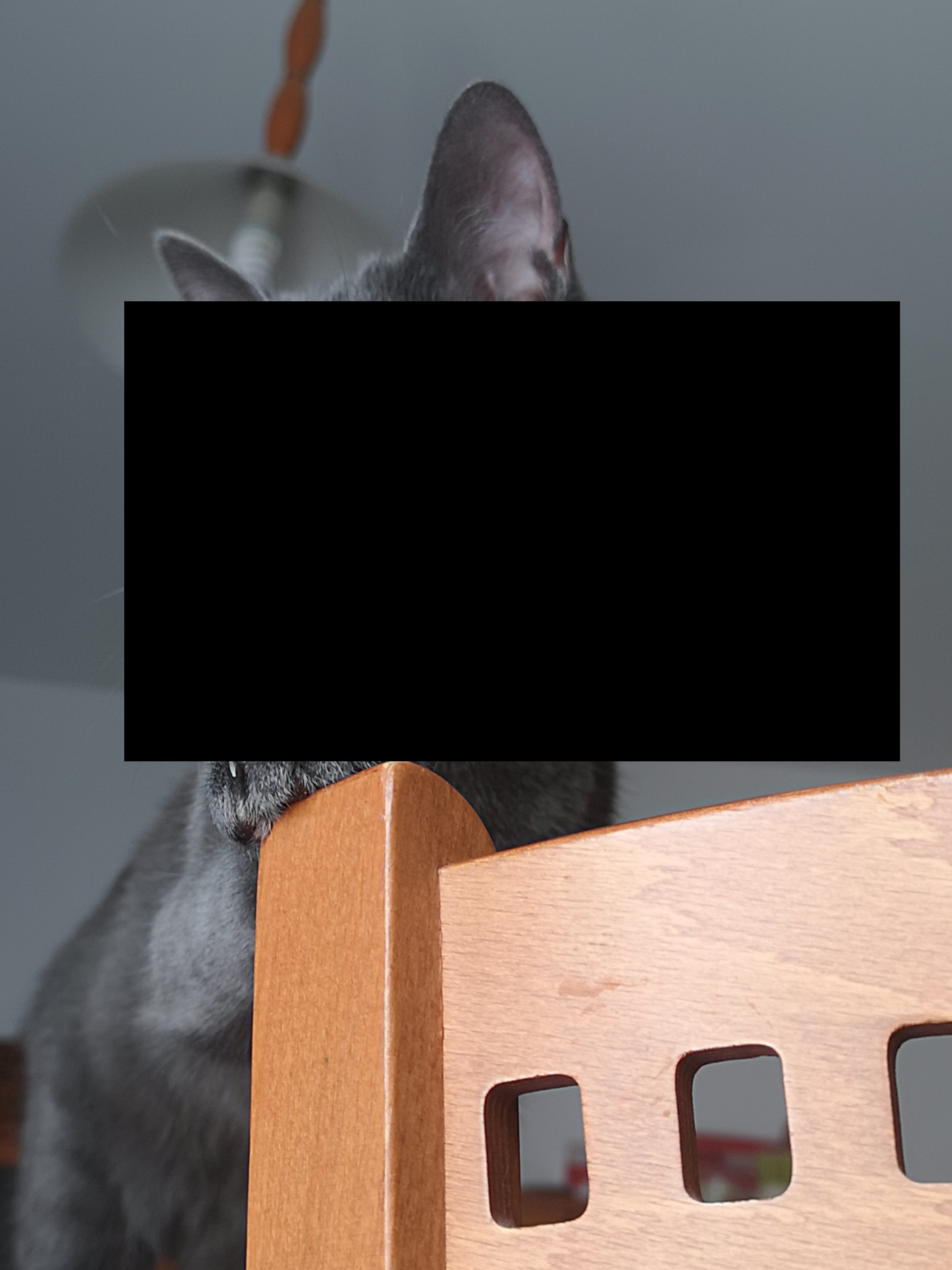 |
img = T.ToTensor()(img)
out_img = T.RandomErasing(p=1, scale=(0.02, 0.33), ratio=(0.3, 3.3),
value=0, inplace=False)(img)
out_img = T.ToPILImage()(out_img)
|
|
19. |
|
Randomly converts image to grayscale with a probability of p .
|
PHOTOMETRY
|
20. |
|
Horizontally flips the given image randomly with a given probability.
|
BASIC GEOMETRY
|
Original |
Augmented |
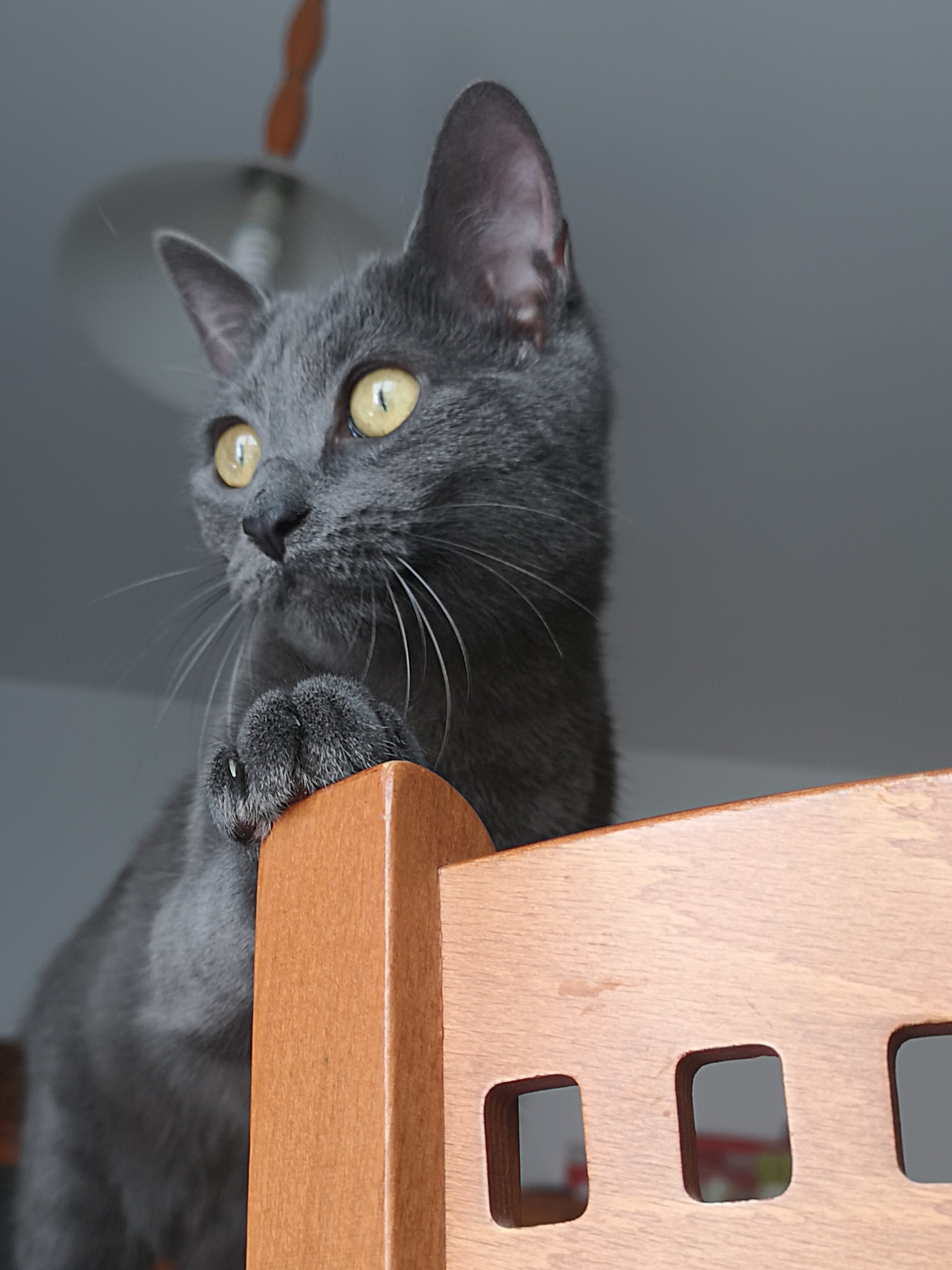 |
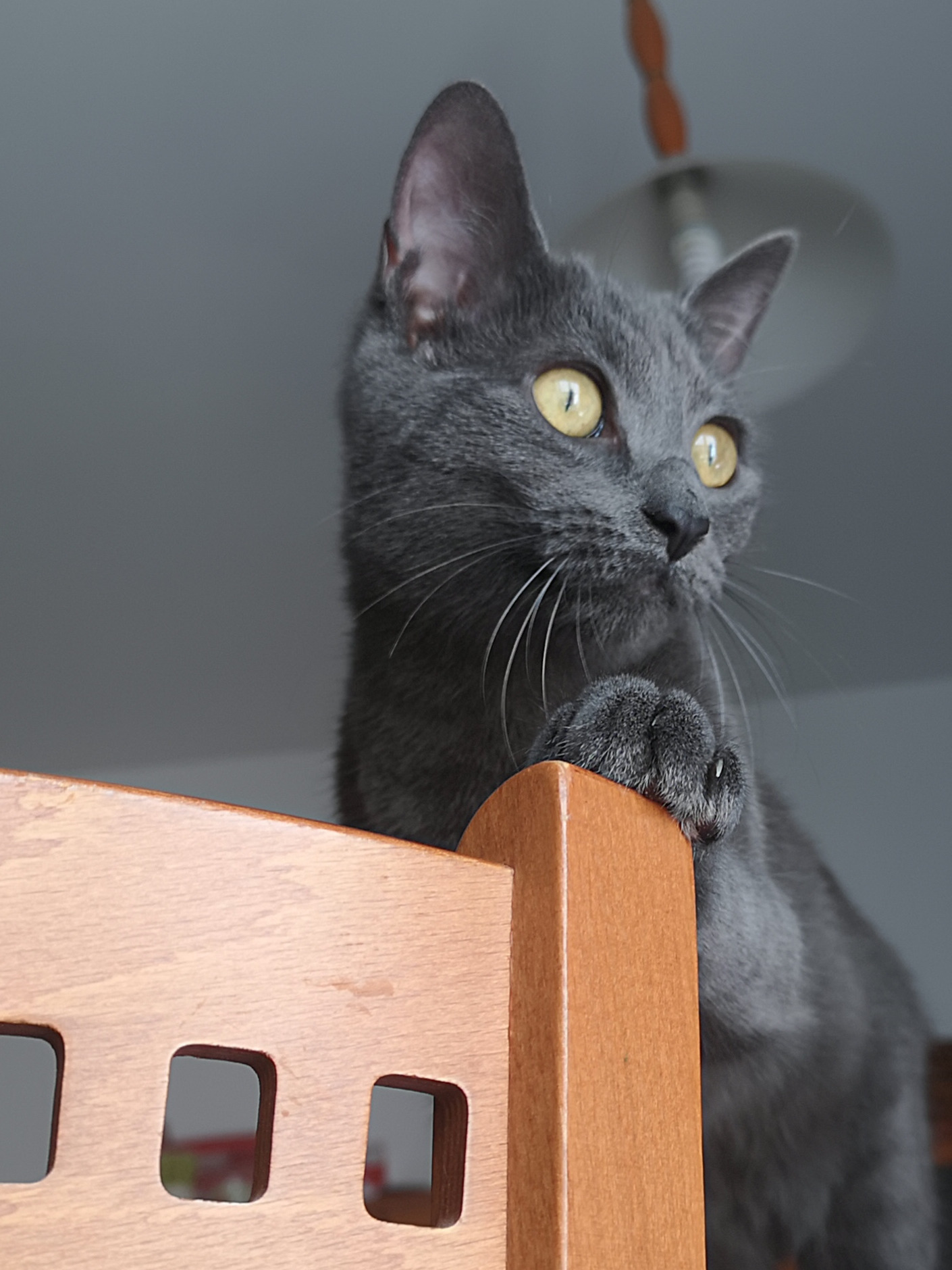 |
out_img = T.RandomHorizontalFlip(p=1)(img)
|
|
21. |
|
Inverts the colors of the given image randomly with a given probability.
|
PHOTOMETRY
|
Original |
Augmented |
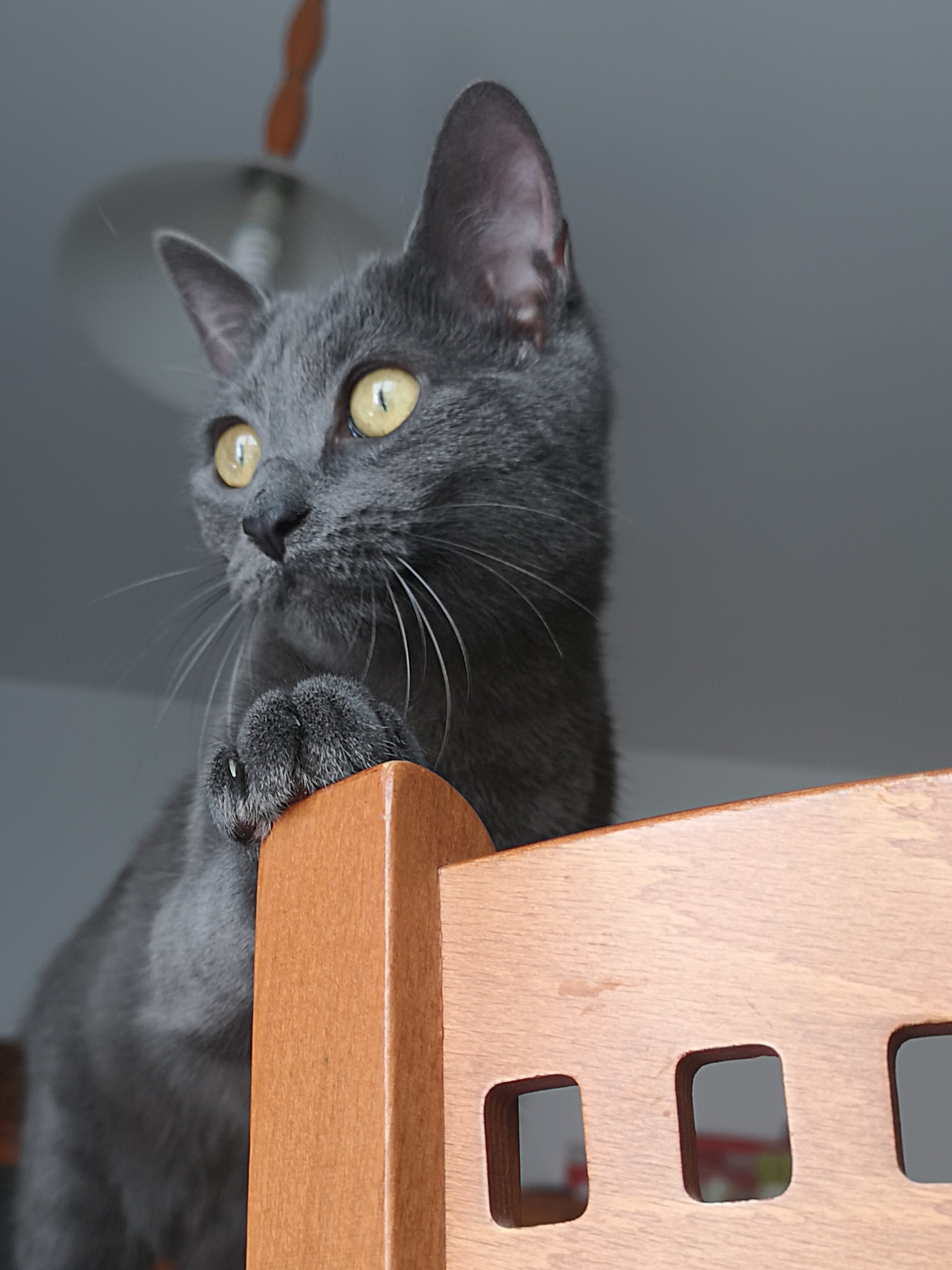 |
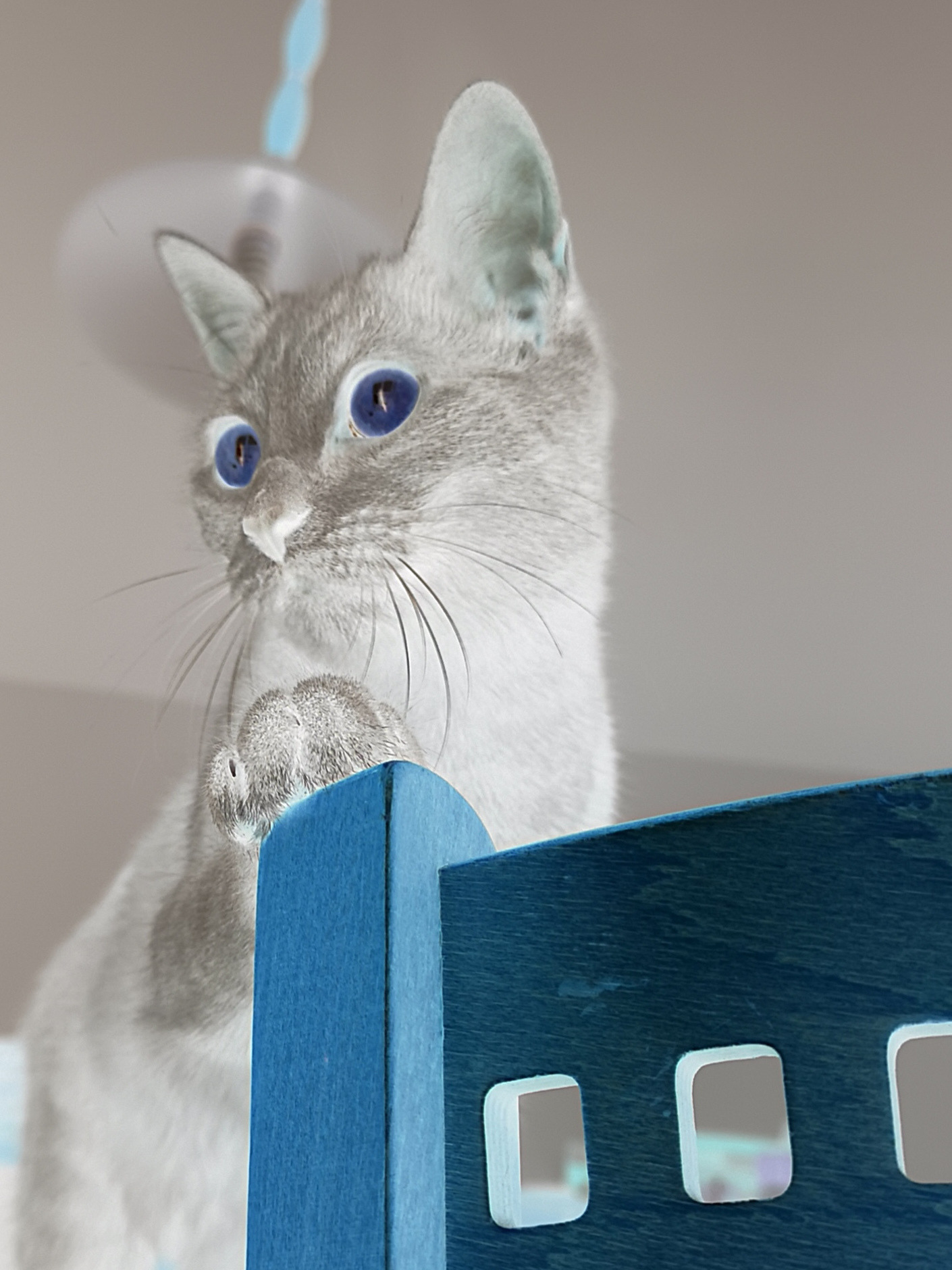 |
out_img = T.RandomInvert(p=1)(img)
|
|
22. |
|
Applies a list of transformations in a random order.
|
|
23. |
|
Performs a random perspective transformation of the given image with a given probability.
|
BASIC GEOMETRY
|
Original |
Augmented |
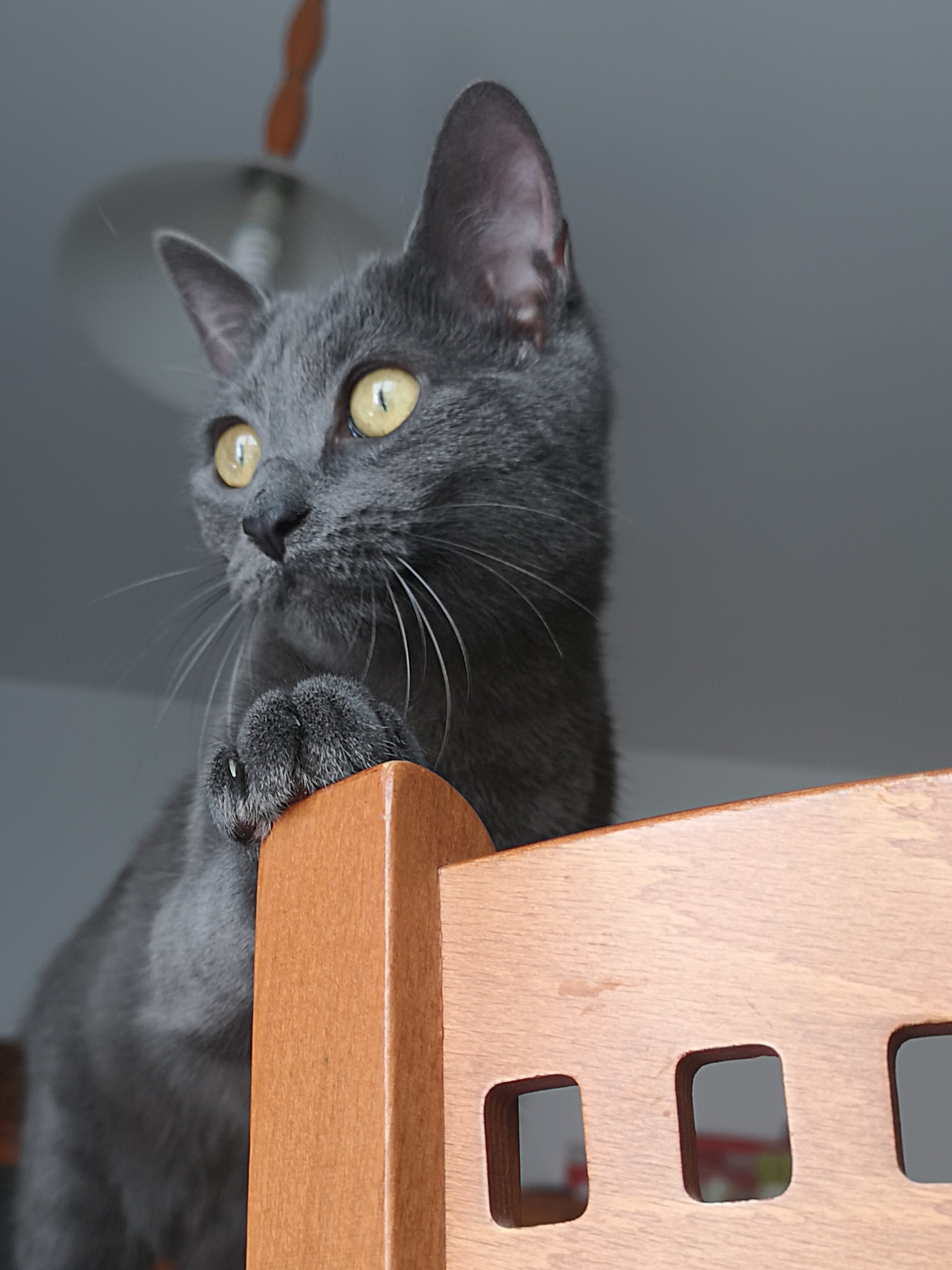 |
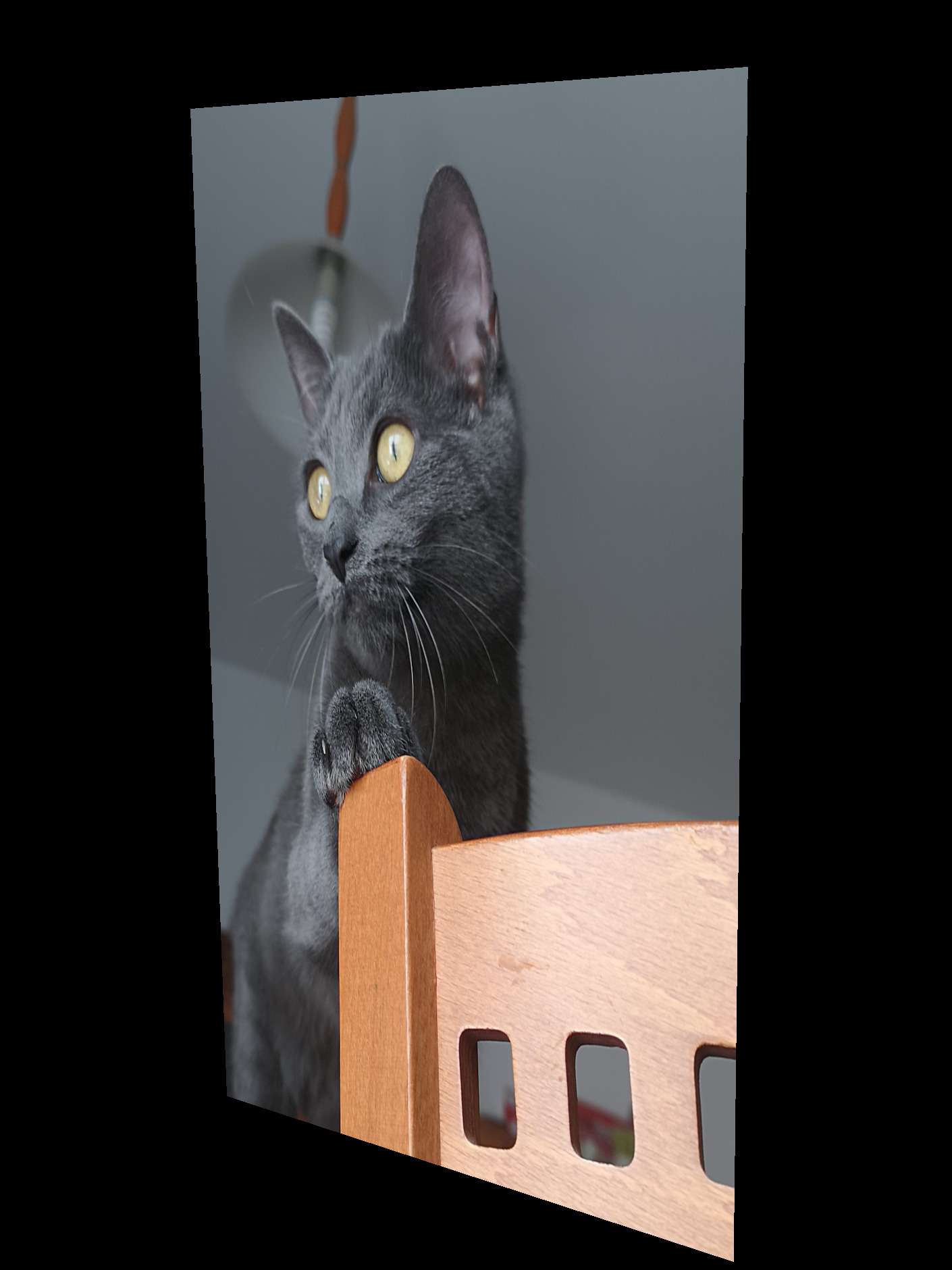 |
out_img = T.RandomPerspective(distortion_scale=0.5, p=1,
interpolation=T.InterpolationMode.BILINEAR,
fill=0)(img)
|
|
24. |
|
Posterizes the image randomly with a given probability by reducing the number of bits for each color channel.
|
STYLE FILTER
|
Original |
Augmented |
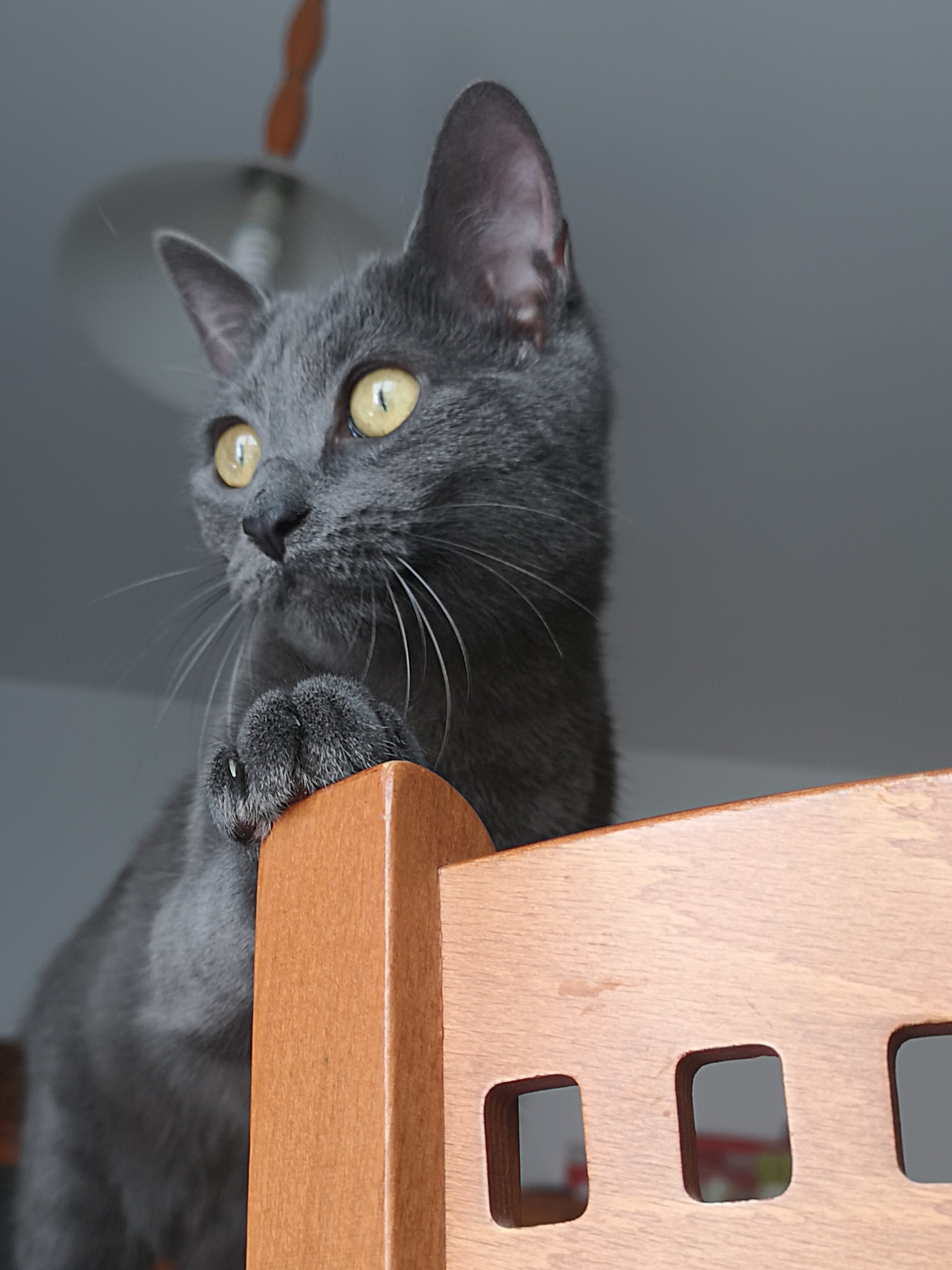 |
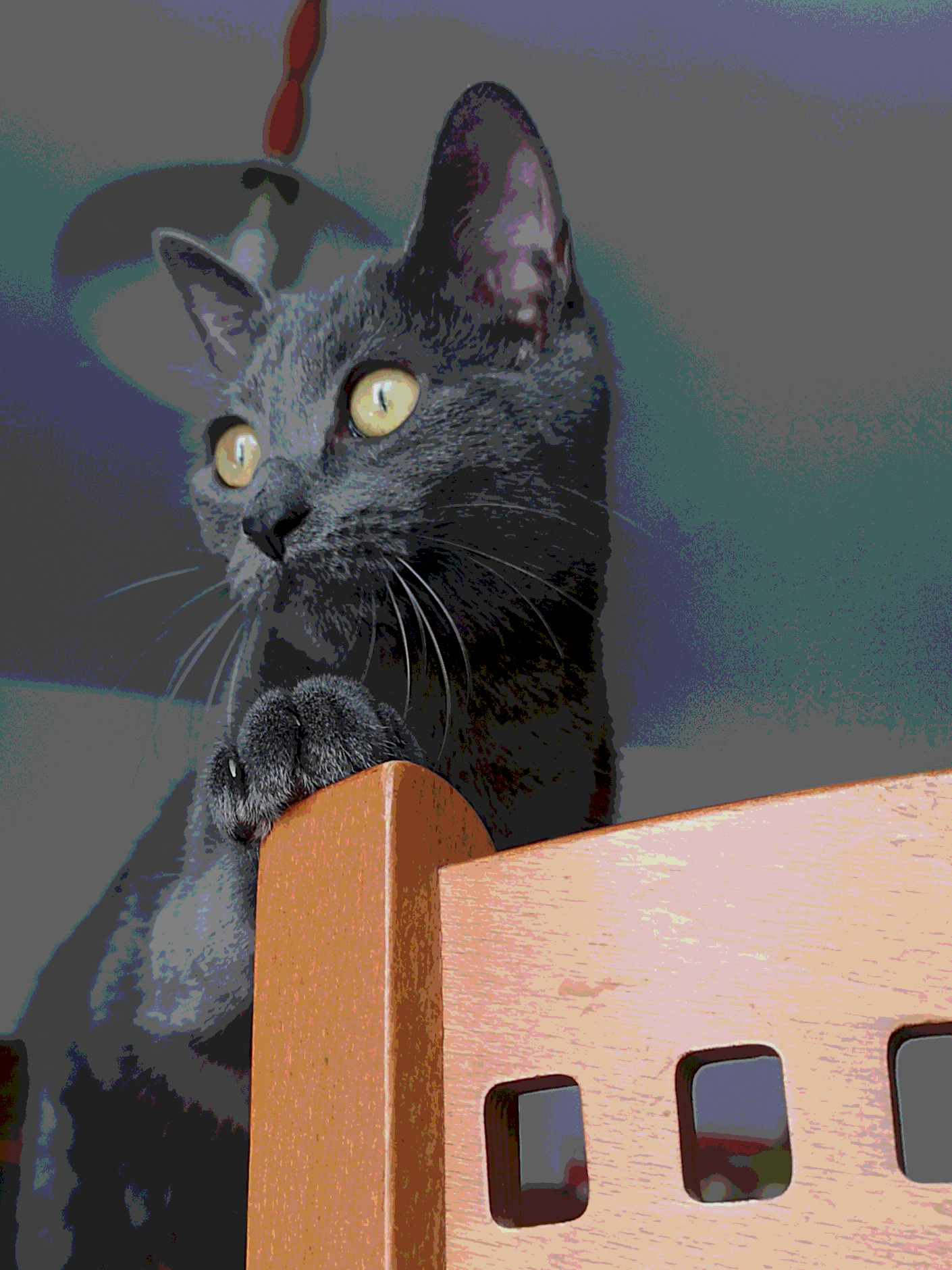 |
out_img = T.RandomPosterize(3, p=1)(img)
|
|
25. |
|
Crops a random portion of image and resize it to a given size.
|
BASIC GEOMETRY
|
Original |
Augmented |
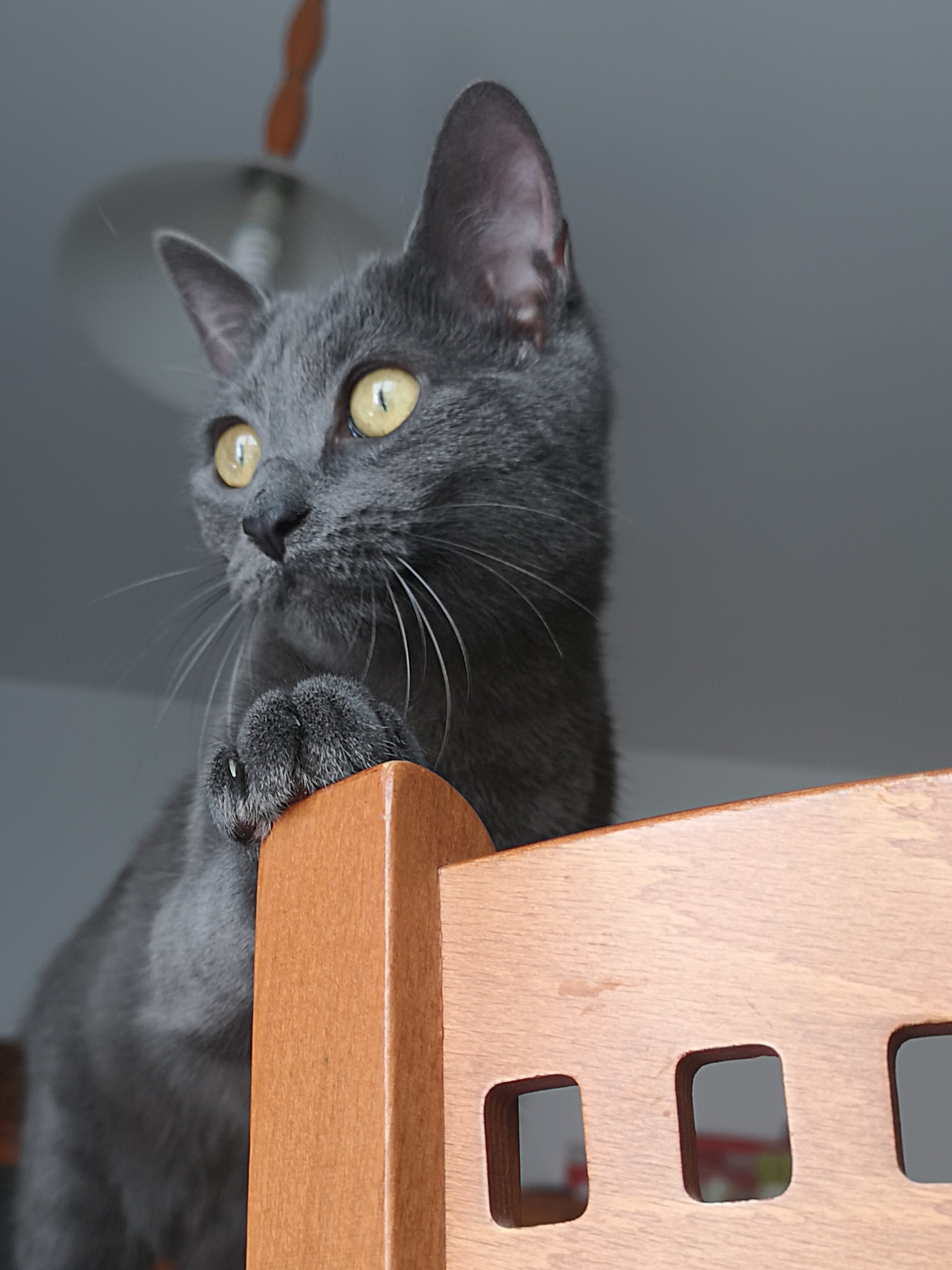 |
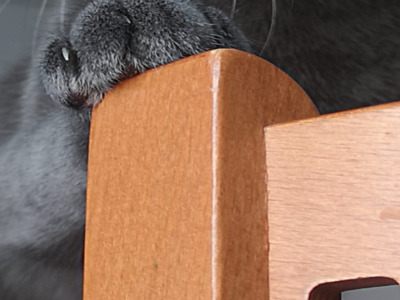 |
out_img = T.RandomResizedCrop((300,400), scale=(0.08, 1.0),
ratio=(0.75, 1.33),
interpolation=T.InterpolationMode.BILINEAR,
antialias=None)(img)
|
|
26. |
|
Rotates the image by angle.
|
BASIC GEOMETRY
|
Original |
Augmented |
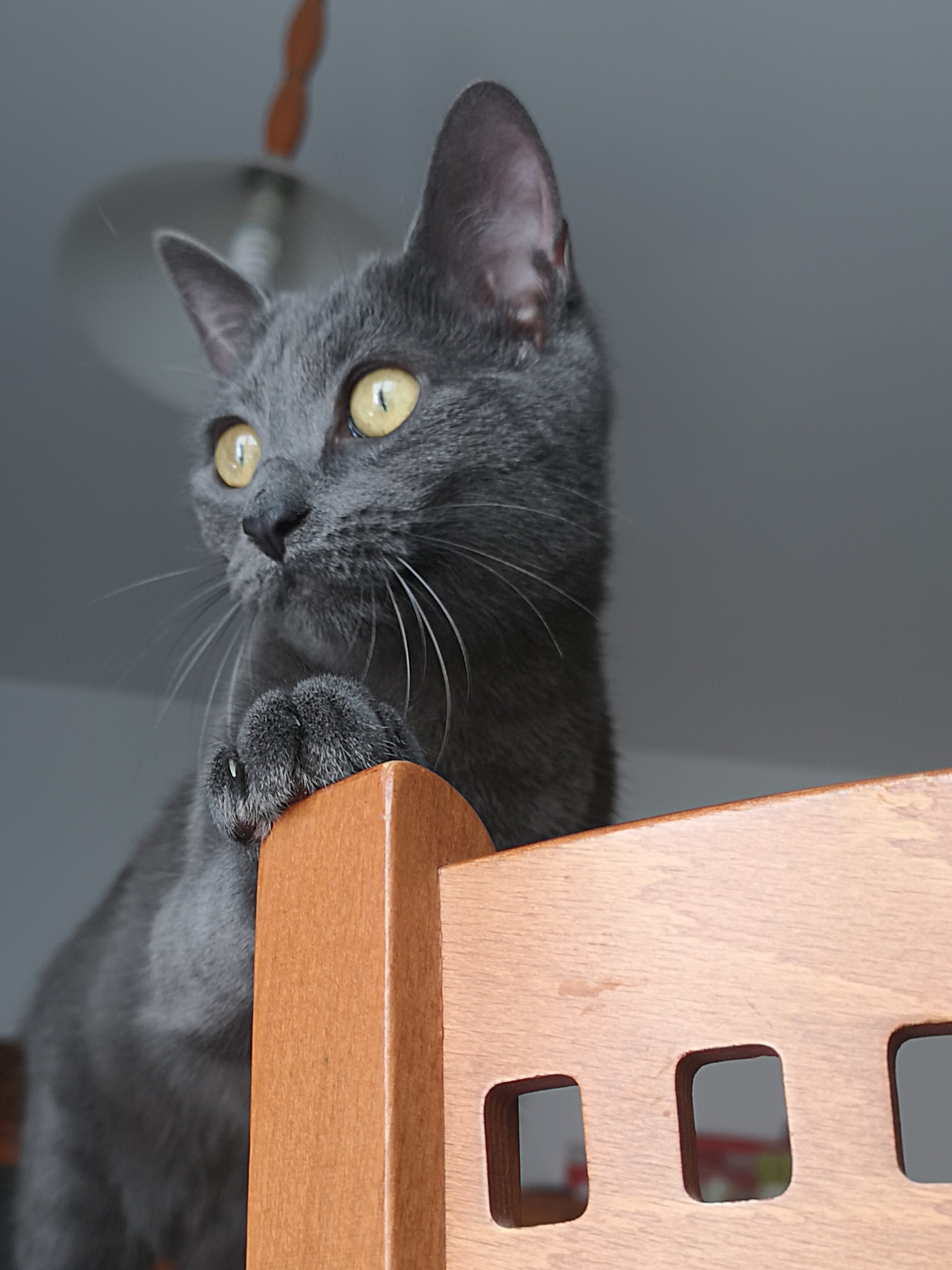 |
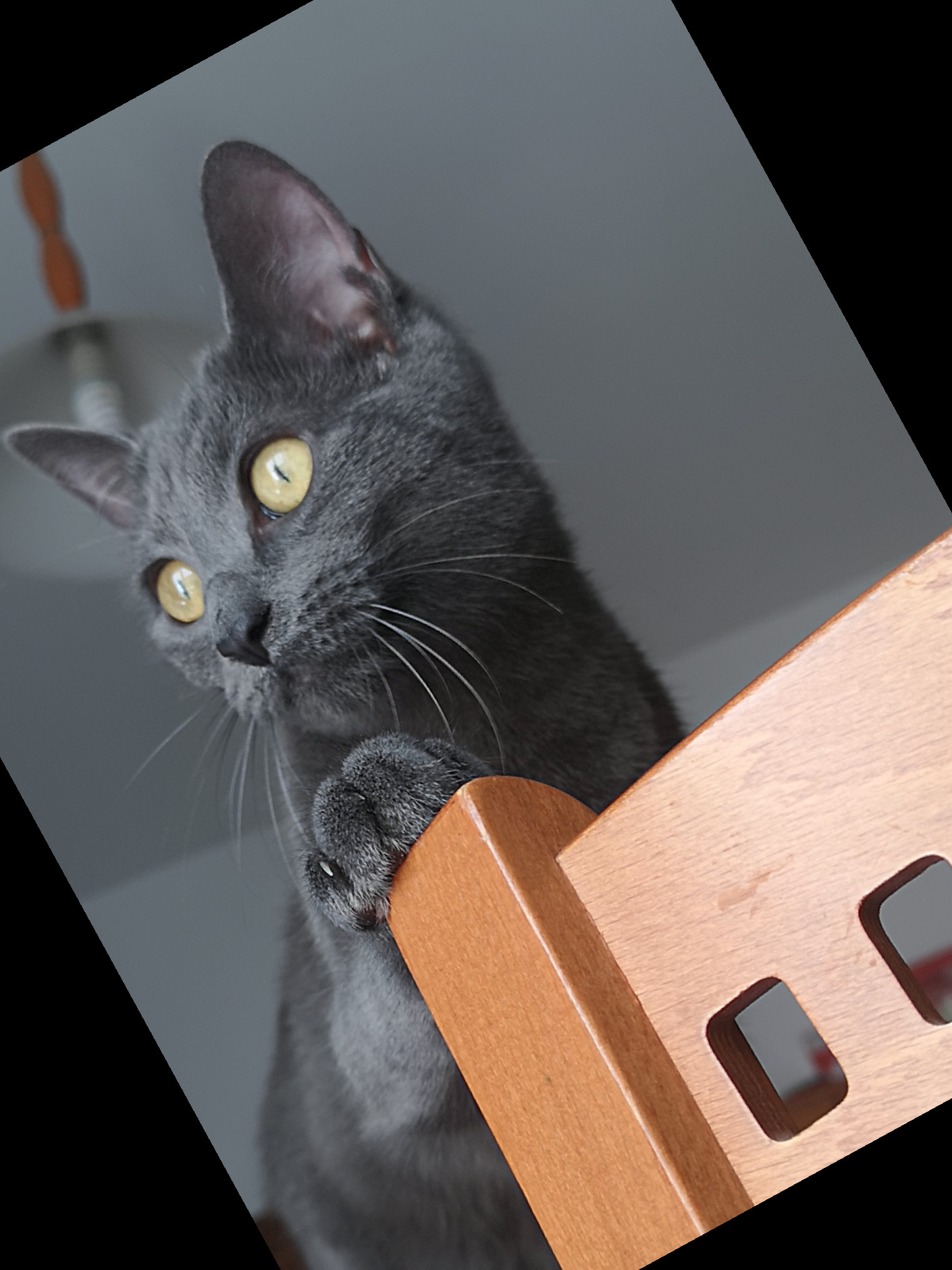 |
out_img = T.RandomRotation(90, interpolation=T.InterpolationMode.NEAREST,
expand=False, center=None, fill=0)(img)
|
Original |
Augmented |
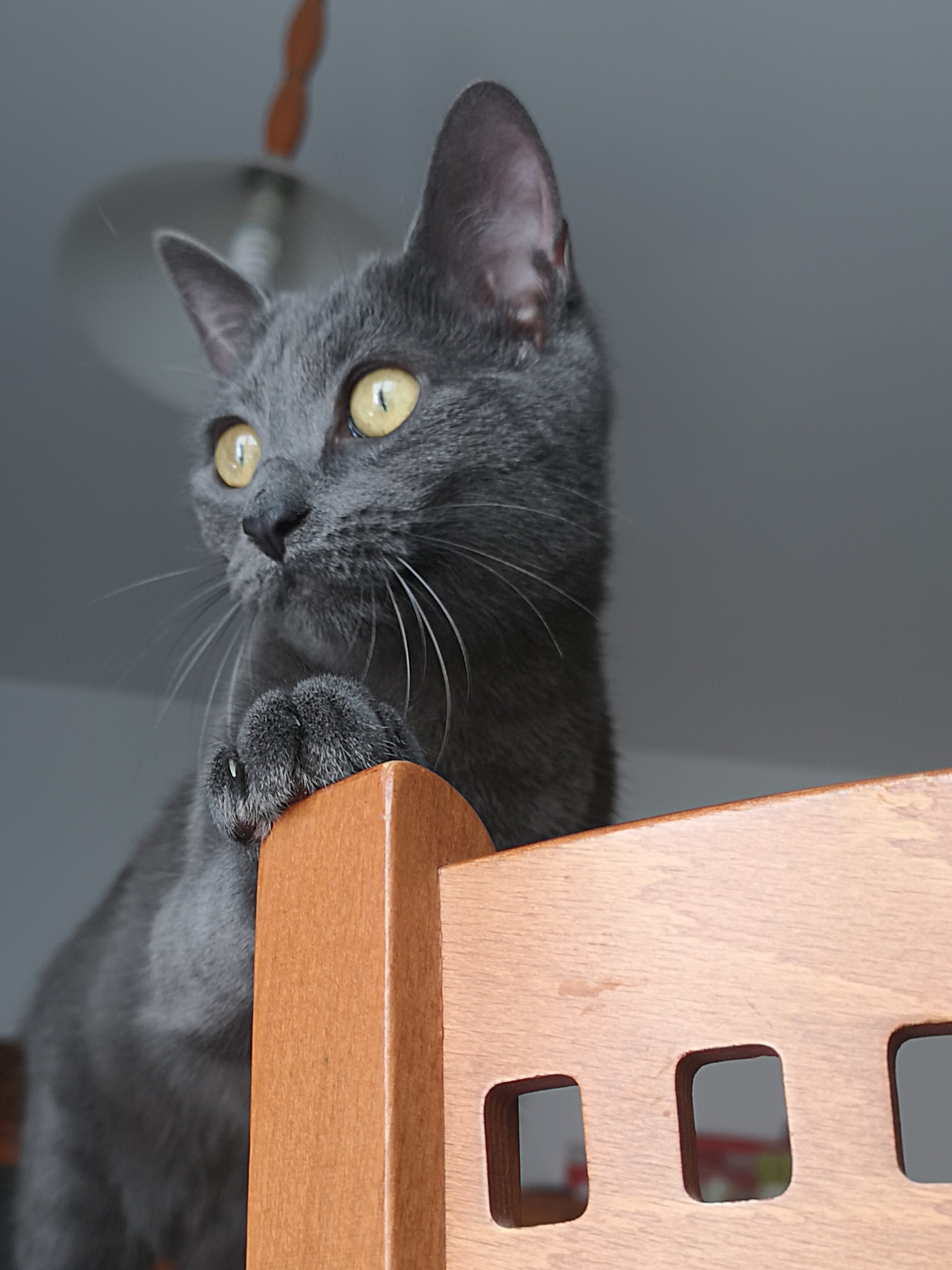 |
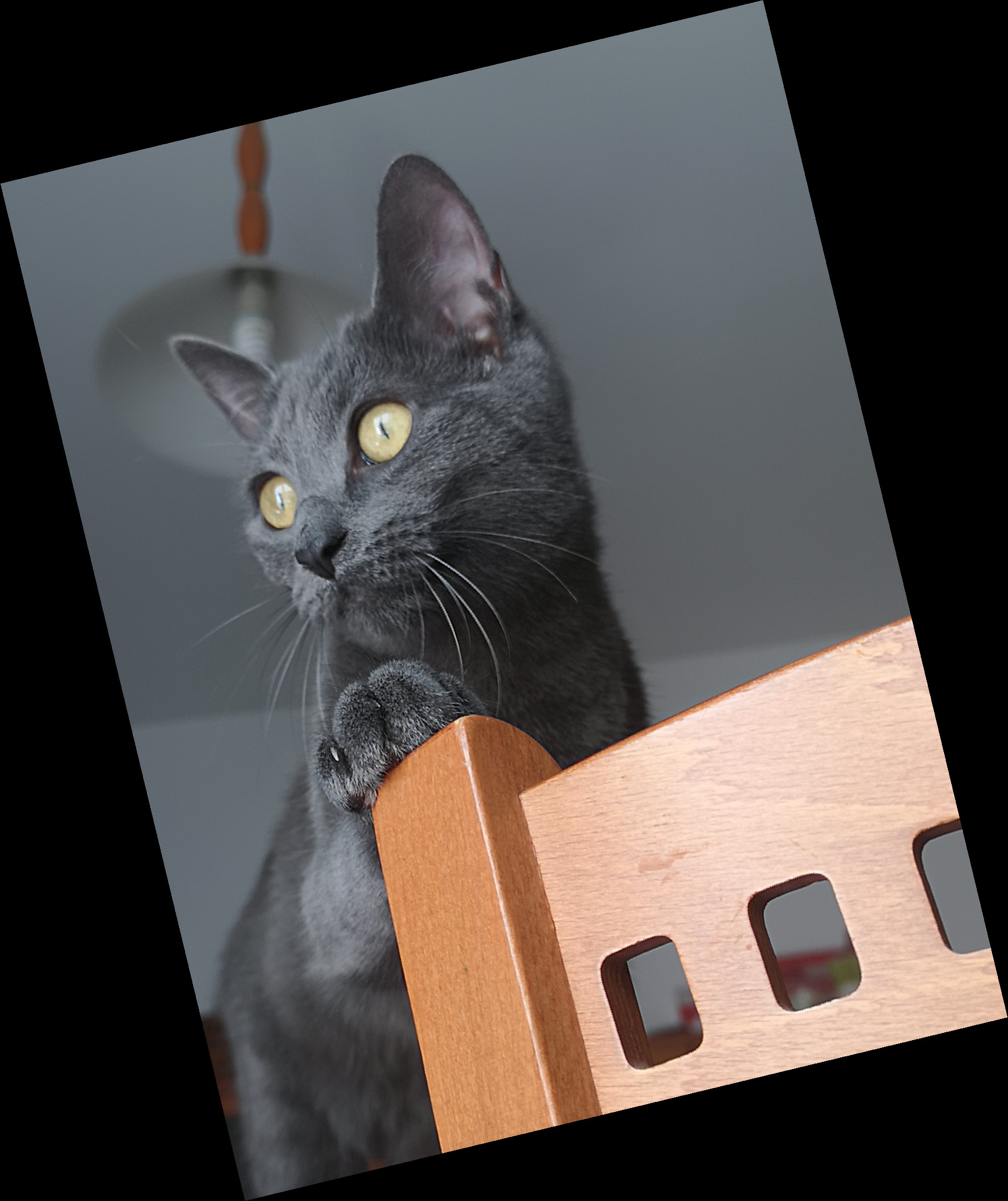 |
out_img = T.RandomRotation(90, interpolation=T.InterpolationMode.NEAREST,
expand=True, center=None, fill=0)(img)
|
|
27. |
|
Solarizes the image randomly with a given probability by inverting all pixel values above a threshold.
|
PHOTOMETRY
|
Original |
Augmented |
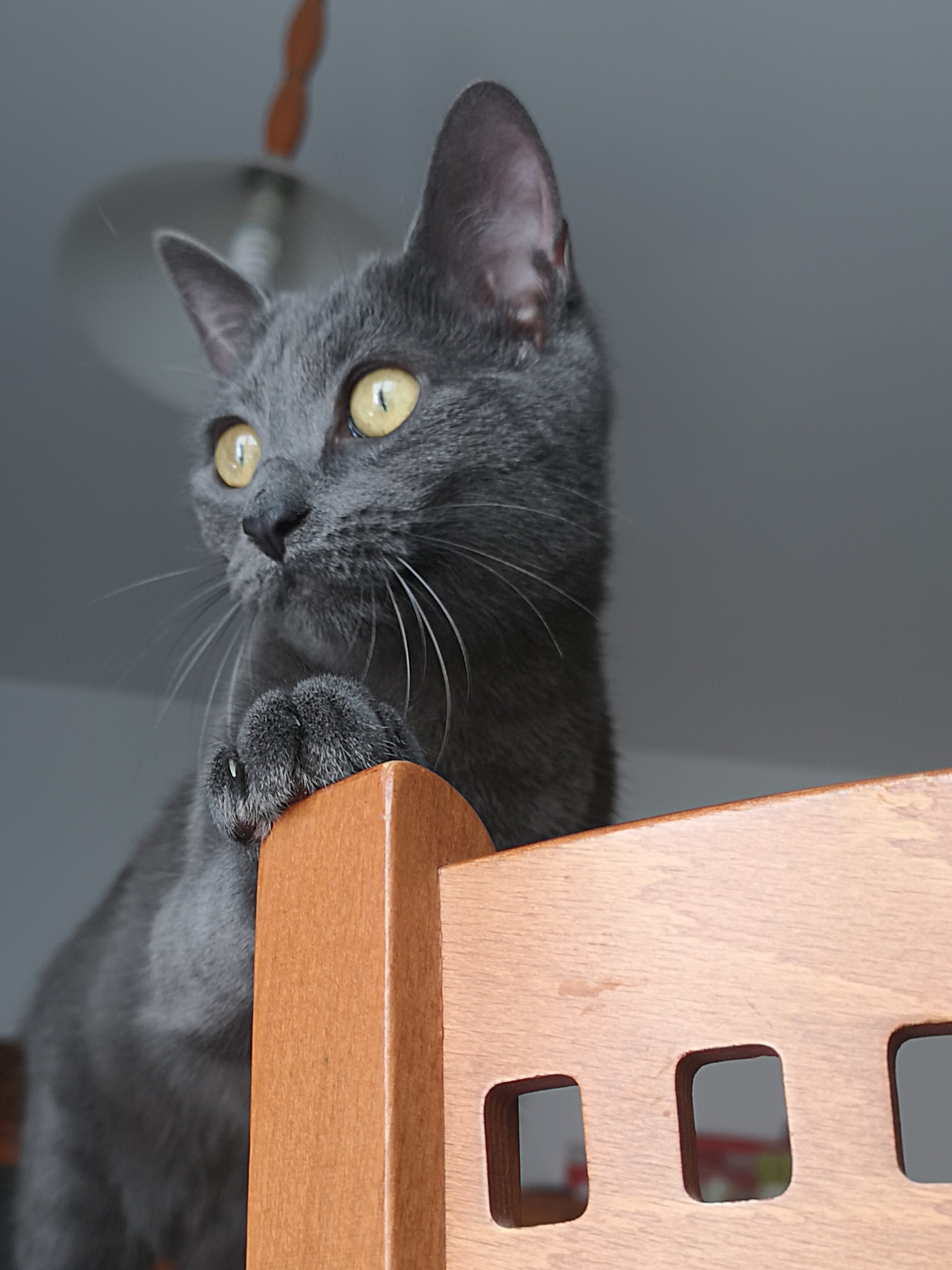 |
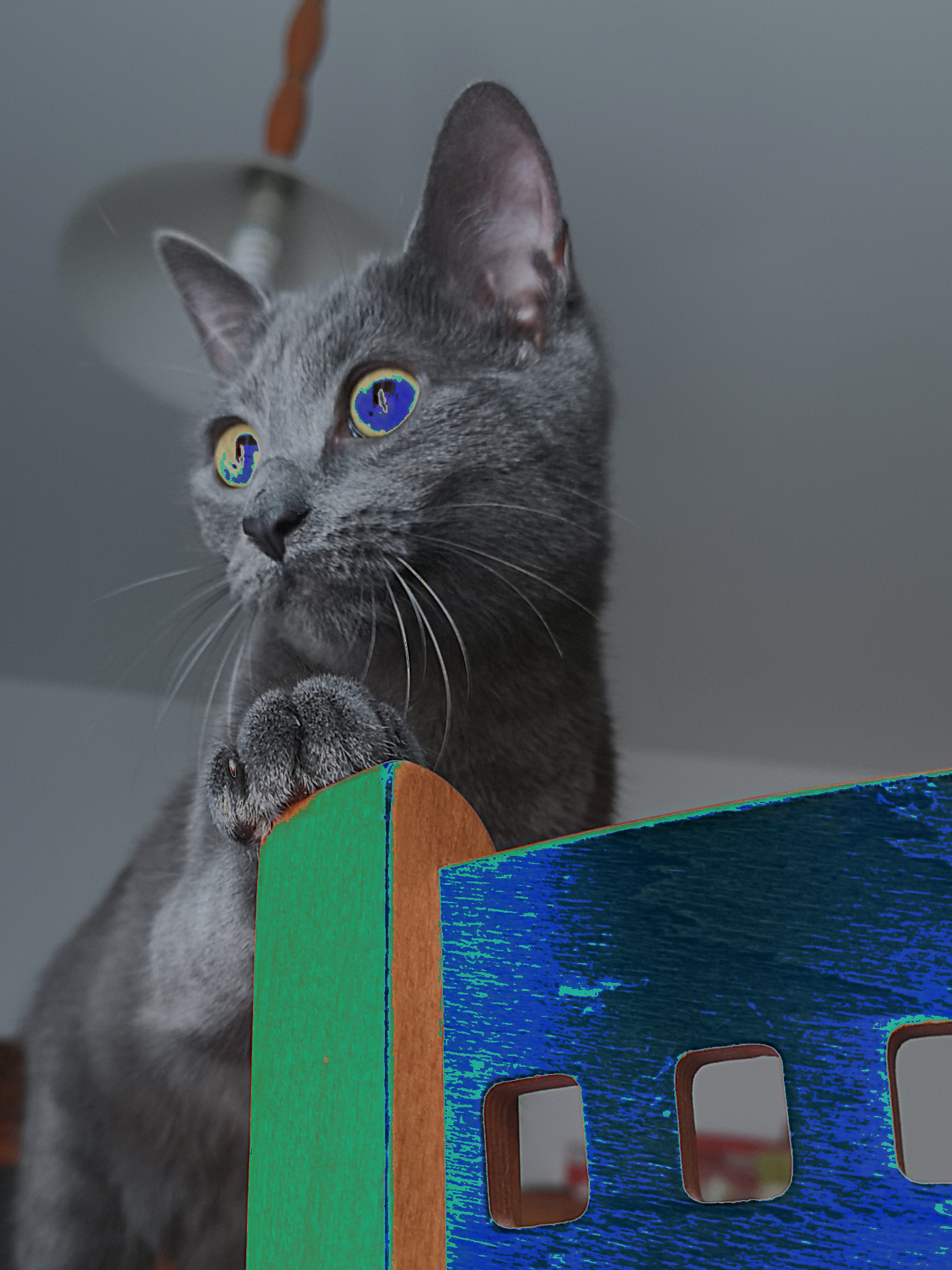 |
out_img = T.RandomSolarize(180, p=1)(img)
|
|
28. |
|
Vertically flips the given image randomly with a given probability.
|
BASIC GEOMETRY
|
Original |
Augmented |
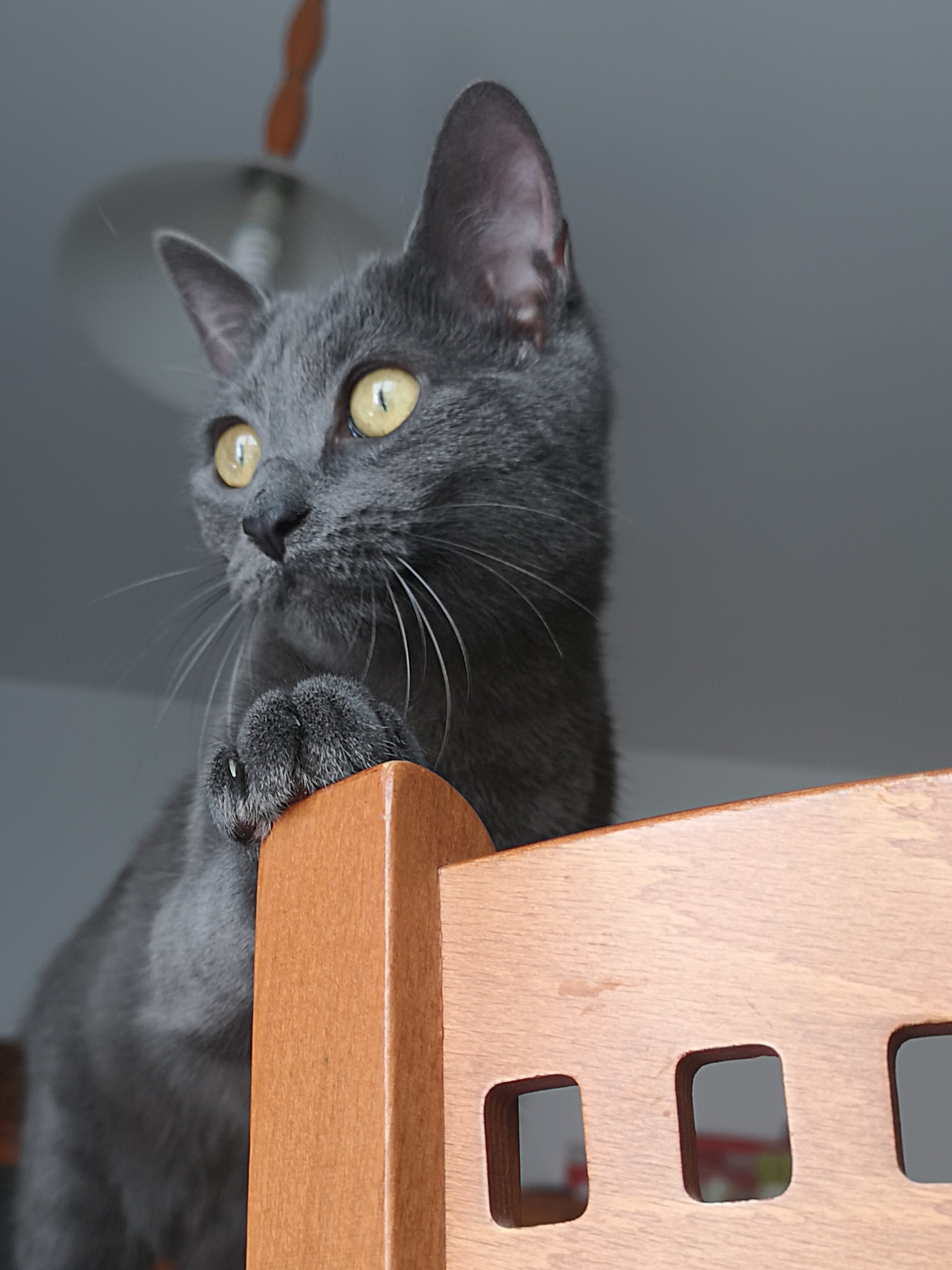 |
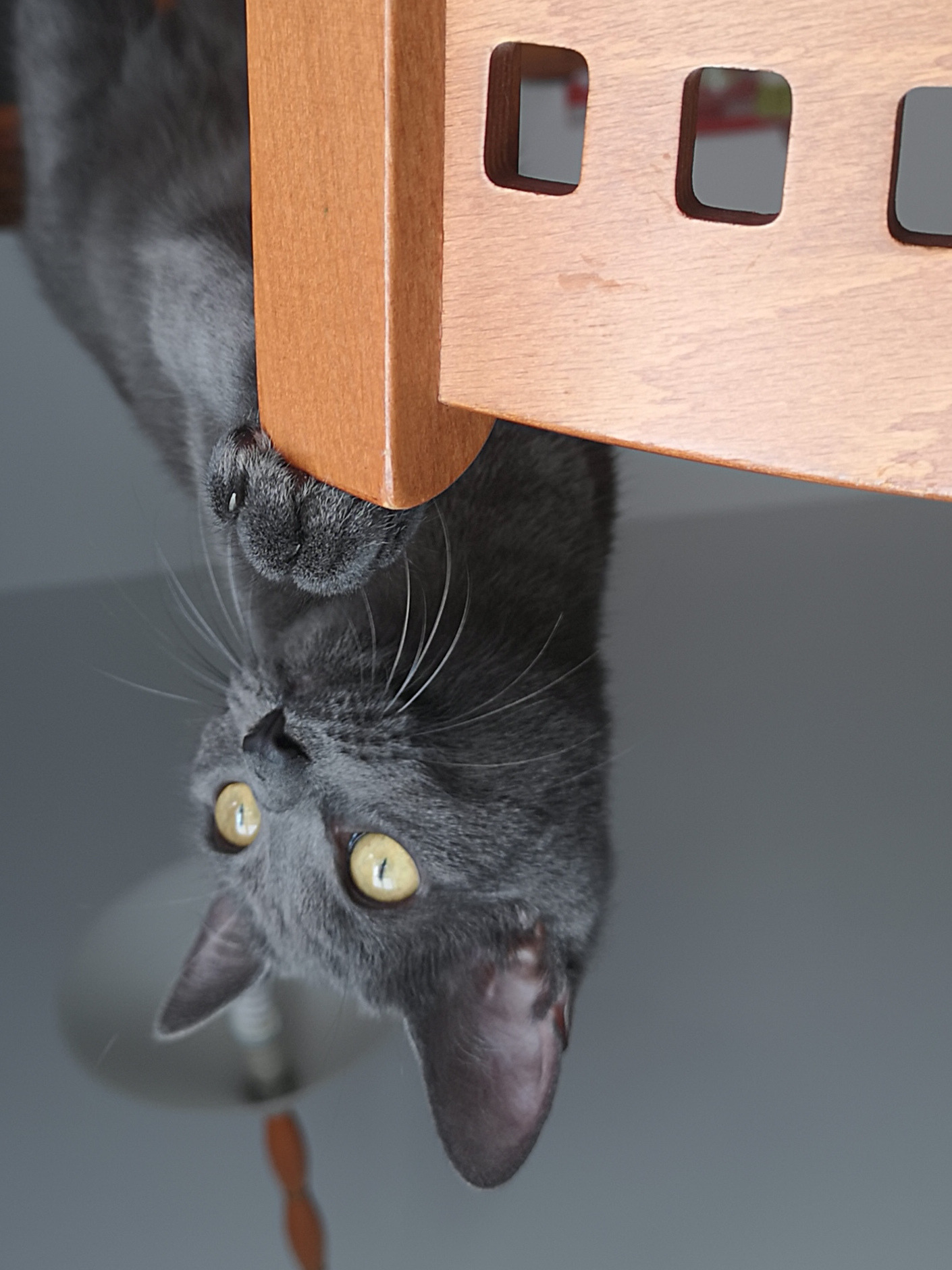 |
out_img = T.RandomVerticalFlip(p=1)(img)
|
|
29. |
|
Resizes the input image to the given size.
|
BASIC GEOMETRY
|
Original |
Augmented |
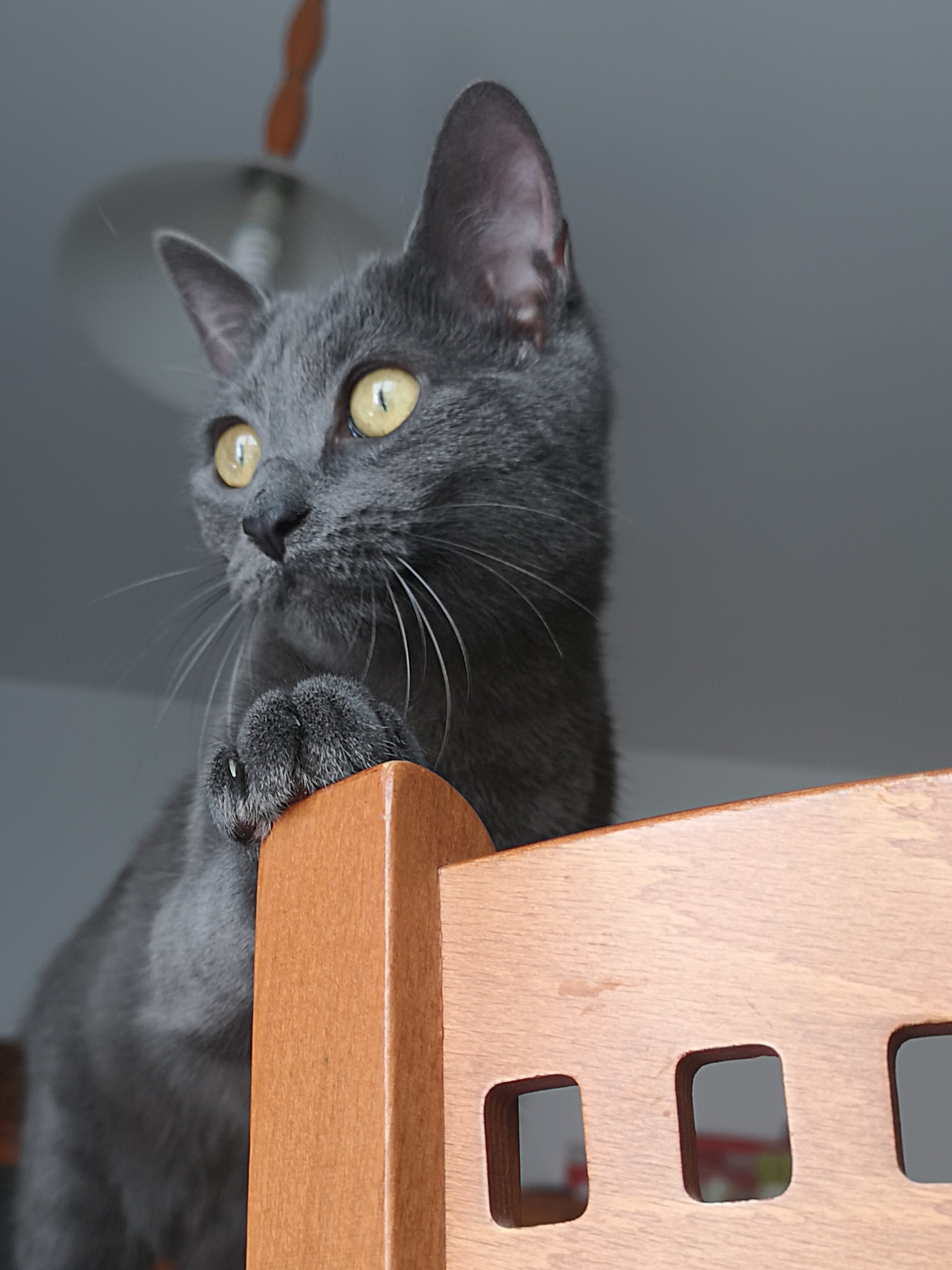 |
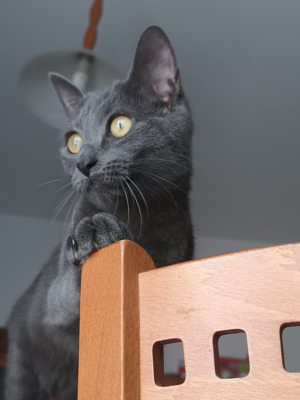 |
out_img = T.Resize(300, interpolation=T.InterpolationMode.BILINEAR, max_size=None, antialias=None)(img)
|
|
30. |
|
Crops the given image into four corners and the central crop plus the flipped version of these (horizontal flipping is used by default).
|
BASIC GEOMETRY
|
31. |
|
Dataset-independent data-augmentation with TrivialAugment Wide.
|
|
Original |
Augmented |
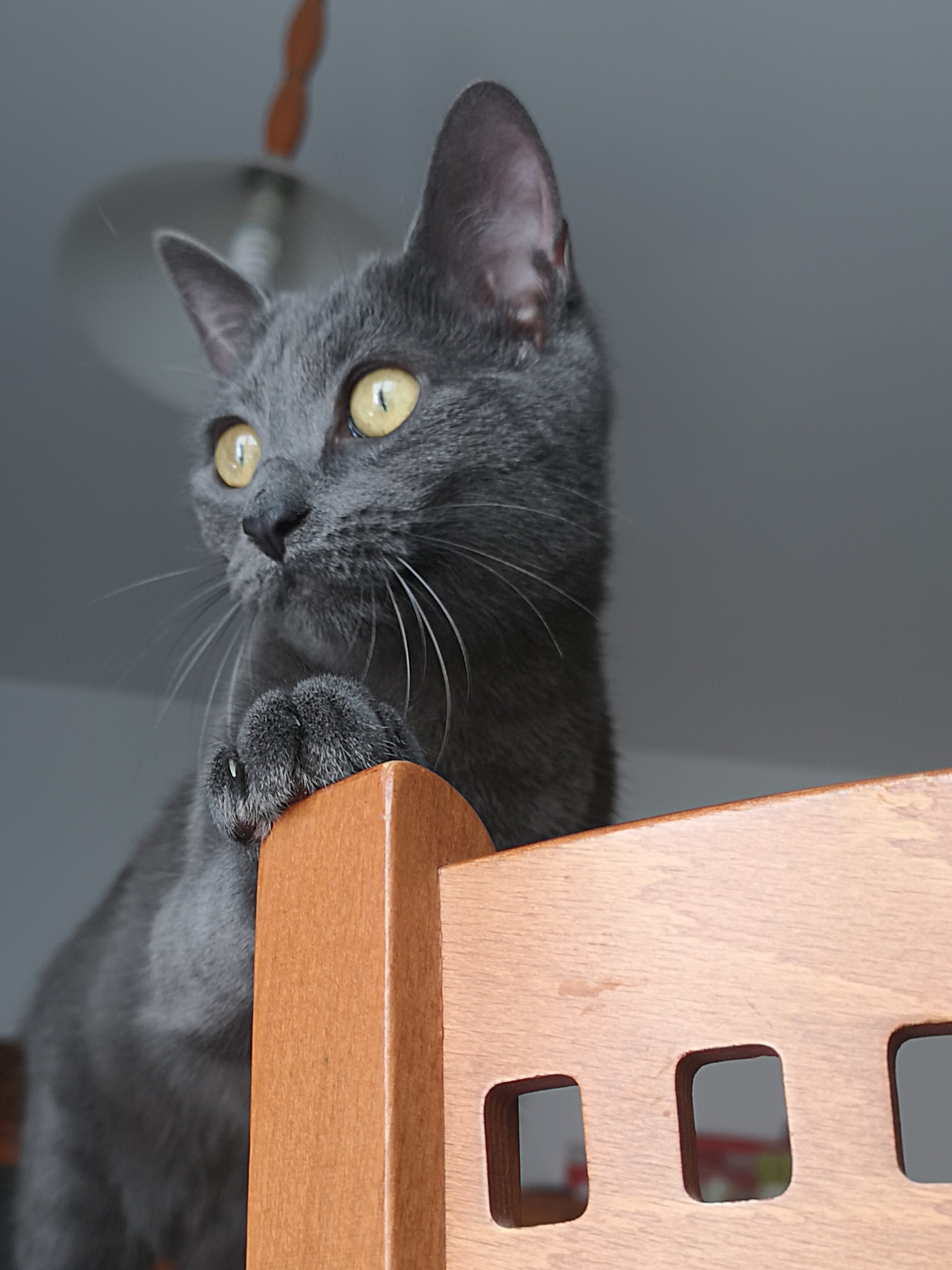 |
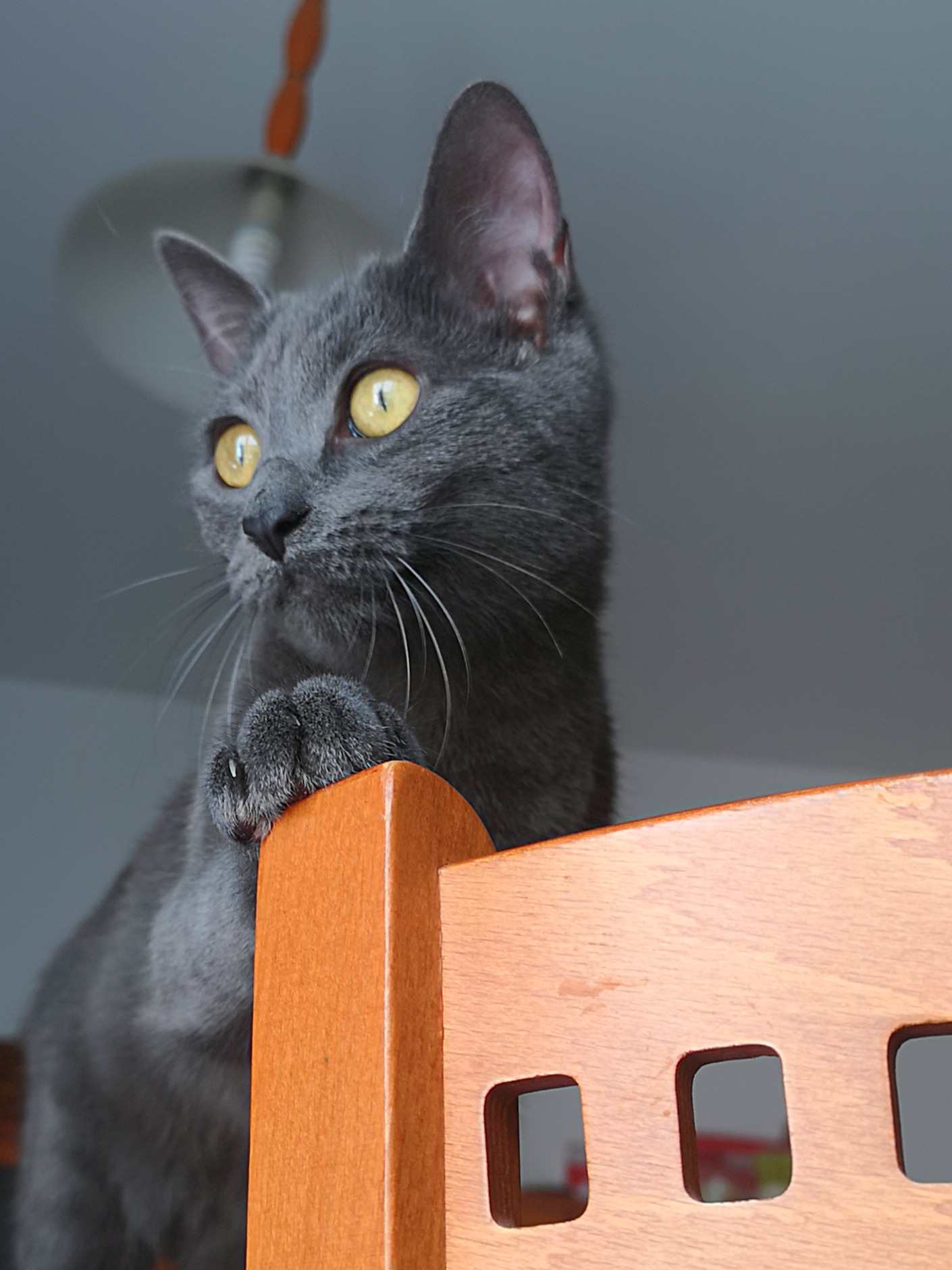 |
Original |
Augmented |
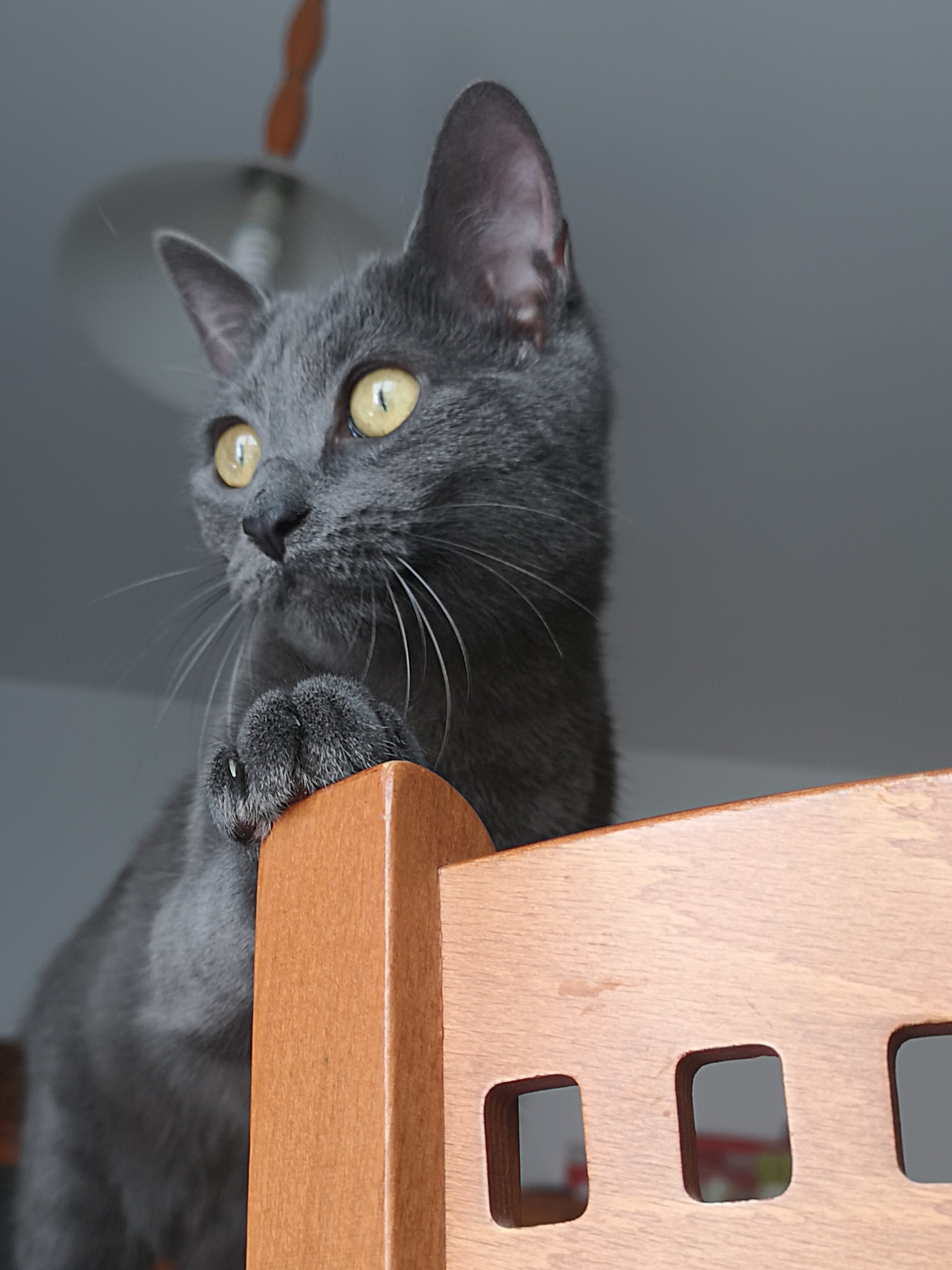 |
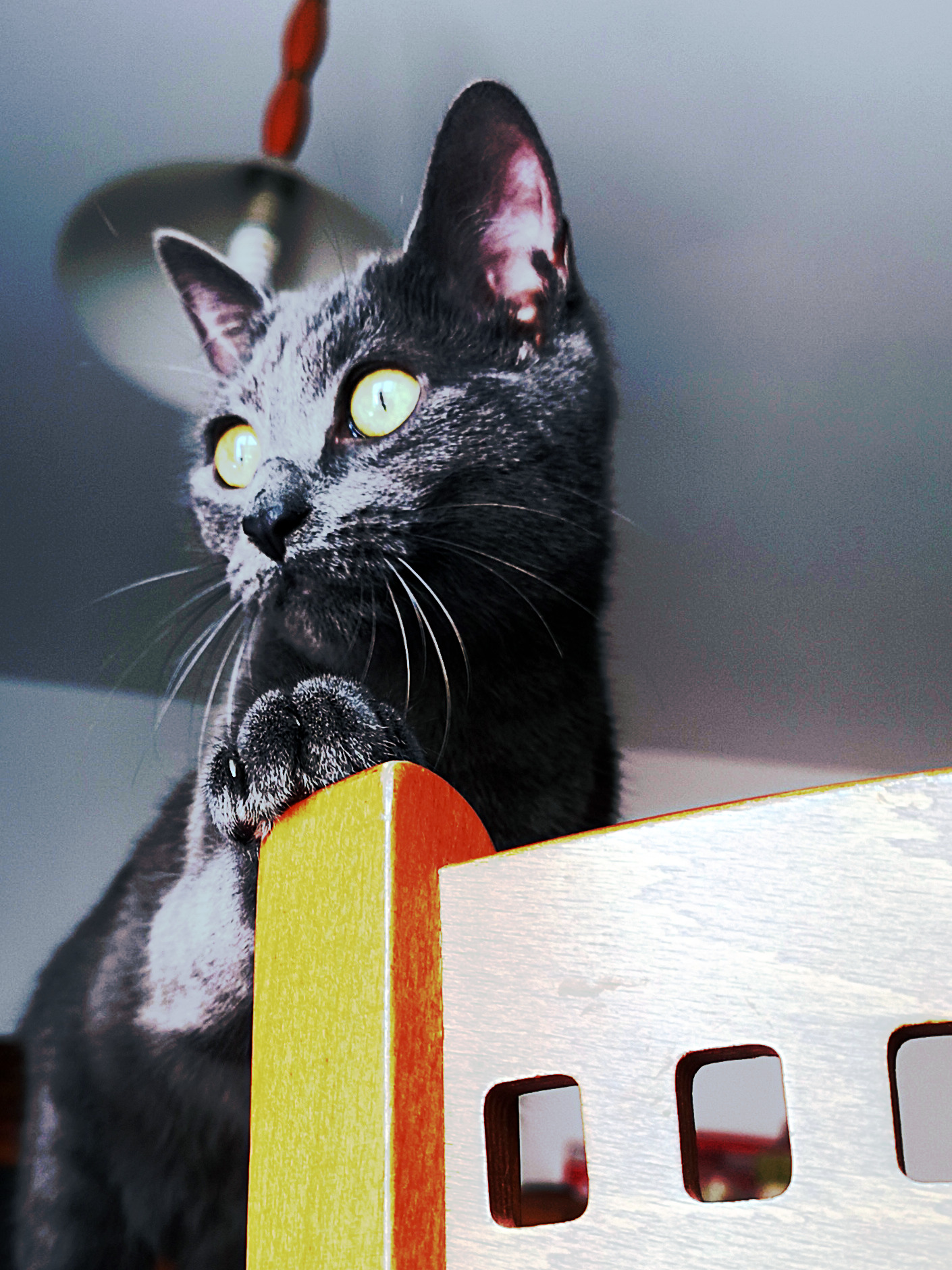 |
out_img = T.TrivialAugmentWide(num_magnitude_bins=31,
interpolation=T.InterpolationMode.NEAREST,
fill=None)(img)
|
|